Optimizing Performance with jQuery: Best Practices and Techniques
In today’s fast-paced digital world, website performance plays a crucial role in attracting and retaining users. Slow-loading pages and unresponsive user interfaces can lead to high bounce rates and dissatisfied visitors. To address these challenges and ensure a smooth user experience, developers must optimize their websites’ performance. jQuery, a popular JavaScript library, has been widely used for its ease of use and powerful features. However, without careful implementation, jQuery can contribute to performance bottlenecks. In this blog, we’ll explore the best practices and techniques for optimizing performance with jQuery to create faster, more efficient websites.
Table of Contents
jQuery, as a powerful JavaScript library, simplifies the process of web development by providing a concise and user-friendly API to interact with the Document Object Model (DOM), handle events, and perform AJAX requests, among other functionalities. However, it’s essential to wield this power responsibly to avoid performance issues. Let’s delve into some key practices that will help you optimize the performance of your website when using jQuery.
1. Use the Latest Version of jQuery
jQuery evolves over time, and newer versions often come with performance improvements and bug fixes. Always strive to use the latest stable release of jQuery to take advantage of these enhancements. Upgrading to a newer version could significantly boost the performance of your website and provide better support for modern browsers.
Code Sample:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
2. Minimize DOM Manipulation
One of the primary areas where jQuery can impact performance is excessive DOM manipulation. Frequent modifications to the DOM can trigger unnecessary reflows and repaints, causing delays in rendering the page. Minimize the number of times you manipulate the DOM, and prefer making multiple changes together in a single operation.
Code Sample:
javascript // Inefficient Approach for (let i = 0; i < 1000; i++) { $('#element').append('<p>Item ' + i + '</p>'); } // Efficient Approach let items = ''; for (let i = 0; i < 1000; i++) { items += '<p>Item ' + i + '</p>'; } $('#element').append(items);
3. Optimize Selector Performance
The way you select elements using jQuery can significantly impact performance, especially when dealing with large DOM structures. Avoid using overly generic selectors like $(‘div’), as they force jQuery to search through the entire DOM. Be specific with your selectors to target only the necessary elements efficiently.
Code Sample:
javascript // Inefficient Selector $('.items li').css('color', 'red'); // Efficient Selector $('#item-list li').css('color', 'red');
4. Cache jQuery Objects
Whenever you use a jQuery selector, the library must query the DOM to find matching elements. If you intend to use the same selector multiple times, cache the jQuery object in a variable. This way, you can avoid redundant DOM searches and improve performance.
Code Sample:
javascript // Without Caching for (let i = 0; i < 1000; i++) { $('.element').addClass('active'); } // With Caching const $element = $('.element'); for (let i = 0; i < 1000; i++) { $element.addClass('active'); }
5. Event Delegation
Event delegation is a powerful technique to optimize event handling, particularly for large lists or dynamic content. Instead of attaching event listeners to each individual element, you attach a single event listener to a parent element and let events bubble up from the children. This approach reduces the number of event listeners and improves performance.
Code Sample:
javascript // Without Event Delegation $('.items li').on('click', function() { // Handle click event for each li element }); // With Event Delegation $('#item-list').on('click', 'li', function() { // Handle click event for li elements within #item-list });
6. Use Native JavaScript Where Appropriate
While jQuery provides a wide range of convenient abstractions, there are instances where using native JavaScript methods can be more efficient. For simple tasks like selecting elements by ID or class, consider using document.getElementById() or document.querySelector() instead of jQuery selectors.
Code Sample:
javascript // jQuery Approach $('.element').hide(); // Native JavaScript Approach document.querySelectorAll('.element').forEach(el => el.style.display = 'none');
7. Debounce and Throttle Events
Event handling can become a performance bottleneck, especially for events that fire rapidly, like resizing or scrolling. Debouncing and throttling events can help prevent excessive event handling and improve website responsiveness.
Code Sample:
javascript // Debounce Function function debounce(func, delay) { let timeout; return function() { clearTimeout(timeout); timeout = setTimeout(func, delay); }; } // Throttle Function function throttle(func, limit) { let throttling = false; return function() { if (!throttling) { func(); throttling = true; setTimeout(() => throttling = false, limit); } }; } // Usage Example window.addEventListener('resize', debounce(handleResize, 250));
8. Use Asynchronous Loading for External Scripts
If your website relies on external scripts loaded via jQuery’s AJAX methods, consider using asynchronous loading to prevent blocking the main thread. Asynchronous loading allows the rest of your page to continue rendering while waiting for the external content to load.
Code Sample:
javascript $.ajax({ url: 'external-script.js', async: true, dataType: 'script', });
Conclusion
Optimizing performance with jQuery is essential for delivering a seamless user experience and ensuring the success of your website. By following the best practices and techniques outlined in this blog, you can minimize performance bottlenecks and create a faster, more efficient website. Remember to use the latest version of jQuery, minimize DOM manipulation, optimize selectors, and cache jQuery objects when appropriate. Additionally, leverage native JavaScript where it makes sense and implement event delegation, debouncing, and asynchronous loading to further enhance performance. By applying these strategies, you’ll unlock the true potential of jQuery and provide your users with an outstanding browsing experience.
Table of Contents
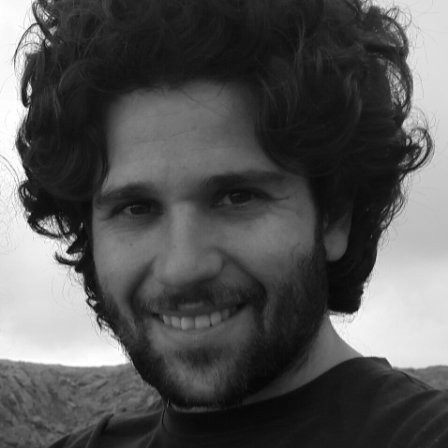
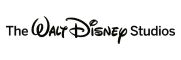