Smooth Page Transitions with jQuery: Enhancing User Navigation
In today’s fast-paced digital world, having a visually appealing and user-friendly website is crucial for success. One aspect of web design that can significantly impact user experience is page transitions. When users navigate between pages on your website, a smooth transition can make the browsing experience more engaging and enjoyable. In this blog, we’ll explore how to achieve smooth page transitions using jQuery, a popular JavaScript library, and enhance user navigation on your website.
Table of Contents
1. Why Smooth Page Transitions Matter
When a user clicks on a link or a button to navigate from one page to another, the standard browser behavior is to load the new page, causing a noticeable delay and sometimes even a flicker. These abrupt transitions can create a disjointed experience for the user, making them feel disconnected from your website.
Smooth page transitions, on the other hand, offer a seamless flow as users move from one page to another. By employing smooth transitions, you can maintain the user’s attention and reduce the perception of load times, leading to higher engagement and satisfaction.
2. Getting Started with jQuery
Before we dive into creating smooth page transitions, let’s set up our development environment. First, make sure you have the latest version of jQuery. You can include it in your project by downloading it from the official website or using a Content Delivery Network (CDN) link.
html <!DOCTYPE html> <html> <head> <!-- Add your meta tags, title, and other head elements here --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <!-- Your website content goes here --> </body> </html>
3. The Basic Structure
To implement smooth page transitions, we’ll use a combination of jQuery and CSS. The basic idea is to intercept link clicks, prevent the default behavior (loading the new page), fetch the content of the linked page using AJAX, and then transition the content smoothly.
4. HTML
Let’s create a simple HTML structure for our website, with a navigation menu and a content area where the page transitions will occur.
html <!DOCTYPE html> <html> <head> <!-- Add your meta tags, title, and other head elements here --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <nav> <ul> <li><a href="page1.html">Page 1</a></li> <li><a href="page2.html">Page 2</a></li> <li><a href="page3.html">Page 3</a></li> </ul> </nav> <div id="content"> <!-- Page content will be loaded here --> </div> </body> </html>
5. CSS
We’ll use CSS to hide the page content during transitions and apply animation effects. Let’s add some basic CSS styles.
css body { font-family: Arial, sans-serif; margin: 0; padding: 0; } nav { background-color: #333; color: #fff; padding: 10px; } nav ul { list-style: none; margin: 0; padding: 0; display: flex; } nav li { margin-right: 20px; } nav li:last-child { margin-right: 0; } nav a { text-decoration: none; color: #fff; } #content { padding: 20px; transition: opacity 0.5s; } .page-hidden { display: none; }
6. Implementing Smooth Page Transitions
Now that we have our basic structure in place, let’s implement the smooth page transitions using jQuery.
javascript // Wait for the document to be ready $(document).ready(function() { // Intercept link clicks $('nav a').on('click', function(event) { // Prevent default link behavior event.preventDefault(); // Get the target page URL const targetPage = $(this).attr('href'); // Fade out the content area $('#content').fadeOut(300, function() { // Fetch the content of the target page $.ajax({ url: targetPage, success: function(data) { // Update the content area with the new page content $('#content').html(data).fadeIn(300); }, error: function() { // Handle errors, e.g., show a user-friendly message $('#content').html('<p>Oops! Something went wrong.</p>').fadeIn(300); } }); }); }); });
In the above code, we’ve attached a click event handler to all the links in the navigation menu. When a link is clicked, the event handler is triggered, and we prevent the default behavior using event.preventDefault(). Next, we fade out the content area using fadeOut().
Inside the fadeOut() method, we initiate an AJAX request to fetch the content of the linked page. On a successful response, we update the content area with the new page content and fade it back in using fadeIn().
In case of an error during the AJAX request, we handle it gracefully by displaying a user-friendly error message.
7. Enhancing the User Experience
While the above implementation provides a basic smooth page transition, we can further enhance the user experience by adding more features and animations.
7.1. Loading Animation
During the transition, it’s a good idea to show a loading animation to let users know that something is happening. We can modify our JavaScript code to add a loading spinner.
html <!-- Add the following HTML inside the content area --> <div id="loading"> <div class="spinner"></div> </div> css /* Add the following CSS for the loading animation */ #loading { display: none; position: fixed; top: 50%; left: 50%; transform: translate(-50%, -50%); z-index: 9999; } .spinner { border: 4px solid rgba(0, 0, 0, 0.3); border-top: 4px solid #333; border-radius: 50%; width: 50px; height: 50px; animation: spin 1s linear infinite; } @keyframes spin { 0% { transform: rotate(0deg); } 100% { transform: rotate(360deg); } }
Update the JavaScript code as follows:
javascript $(document).ready(function() { $('nav a').on('click', function(event) { event.preventDefault(); const targetPage = $(this).attr('href'); // Show the loading animation $('#loading').fadeIn(200); $('#content').fadeOut(300, function() { $.ajax({ url: targetPage, success: function(data) { $('#content').html(data).fadeIn(300, function() { // Hide the loading animation on content fade-in $('#loading').fadeOut(200); }); }, error: function() { $('#content').html('<p>Oops! Something went wrong.</p>').fadeIn(300, function() { // Hide the loading animation on error $('#loading').fadeOut(200); }); } }); }); }); });
Now, when a user clicks on a link, the loading animation will appear until the new content is loaded and displayed.
7.2. Preserve Scroll Position
By default, when a new page is loaded, the scroll position resets to the top of the page. To preserve the scroll position during transitions, we can make use of the history.pushState() method.
javascript $(document).ready(function() { $('nav a').on('click', function(event) { event.preventDefault(); const targetPage = $(this).attr('href'); // Show the loading animation $('#loading').fadeIn(200); const scrollPosition = $(window).scrollTop(); // Get the current scroll position $('#content').fadeOut(300, function() { $.ajax({ url: targetPage, success: function(data) { $('#content').html(data).fadeIn(300, function() { // Hide the loading animation on content fade-in $('#loading').fadeOut(200); $(window).scrollTop(scrollPosition); // Restore the scroll position }); }, error: function() { $('#content').html('<p>Oops! Something went wrong.</p>').fadeIn(300, function() { // Hide the loading animation on error $('#loading').fadeOut(200); }); } }); }); }); });
With this enhancement, users will stay at their current scroll position after the page transition, creating a seamless experience.
7.3. Handling Browser Back/Forward Buttons
To ensure smooth transitions even when users use the browser’s back and forward buttons, we can utilize the popstate event.
javascript // Add the following code outside the click event handler $(window).on('popstate', function() { const targetPage = location.pathname; // Get the target page URL const scrollPosition = $(window).scrollTop(); // Get the current scroll position $('#loading').fadeIn(200); $('#content').fadeOut(300, function() { $.ajax({ url: targetPage, success: function(data) { $('#content').html(data).fadeIn(300, function() { $('#loading').fadeOut(200); $(window).scrollTop(scrollPosition); }); }, error: function() { $('#content').html('<p>Oops! Something went wrong.</p>').fadeIn(300, function() { $('#loading').fadeOut(200); }); } }); }); });
Now, when users click the browser’s back or forward buttons, the page transitions will be just as smooth as when clicking on the navigation links.
Conclusion
In this blog, we’ve explored how to implement smooth page transitions using jQuery to enhance user navigation on your website. By creating seamless transitions between pages, you can significantly improve user experience, engagement, and overall satisfaction.
Remember that the key to successful smooth page transitions lies in finding the right balance between creativity and simplicity. Too many complex animations might overwhelm users, while too few might not make a significant impact. Test different transition styles and gather user feedback to optimize your website’s navigation experience.
So, why wait? Start implementing smooth page transitions on your website today and delight your visitors with an engaging and immersive browsing experience! Happy coding!
Table of Contents
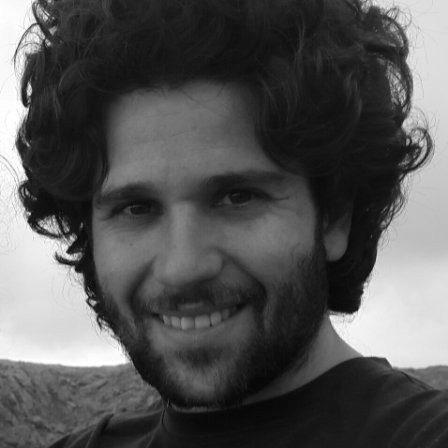
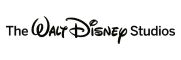