jQuery Plugins: Extending Functionality with Ease
In the ever-evolving world of web development, jQuery has been a game-changer since its inception. With its simplicity, flexibility, and extensive library of plugins, jQuery has become the go-to framework for many developers looking to create dynamic and interactive websites. jQuery plugins are powerful tools that extend jQuery’s functionality, allowing developers to add new features and capabilities effortlessly. In this blog, we will explore the world of jQuery plugins, understand how they work, and learn how to leverage them to enhance the user experience of our web projects.
1. What are jQuery Plugins?
jQuery plugins are pieces of code designed to be easily integrated into jQuery projects to perform specific tasks or add extra functionalities. These plugins are usually written in JavaScript and can be utilized by developers to enhance interactivity, provide animations, handle events, create visual effects, and more. By using plugins, developers can save time and effort, as they don’t have to build these features from scratch.
2. Why Use jQuery Plugins?
There are several advantages to using jQuery plugins in your web development projects:
- Ease of Use: jQuery plugins are straightforward to implement. With just a few lines of code, you can add powerful functionalities to your website.
- Cross-Browser Compatibility: One of the biggest headaches for web developers is ensuring their code works consistently across various browsers. jQuery plugins abstract away many of these compatibility concerns, making it easier to create a consistent user experience.
- Rapid Development: jQuery plugins enable rapid development, allowing you to meet tight project deadlines without sacrificing the quality of your code.
- Wide Variety of Options: The jQuery plugin ecosystem is vast, offering plugins for virtually any functionality you might need. This variety saves you from reinventing the wheel and lets you focus on other aspects of your project.
3. Using jQuery Plugins:
Integrating jQuery plugins into your project is a straightforward process. Let’s look at a step-by-step guide on how to use a jQuery plugin by implementing a simple image gallery with the “FancyGallery” plugin.
Step 1: Include jQuery:
First, include the jQuery library in the <head> section of your HTML file. Ensure that you link to a version that is compatible with the plugin you intend to use.
html <!DOCTYPE html> <html> <head> <title>My Fancy Gallery</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <!-- Your gallery content goes here --> </body> </html>
Step 2: Download the FancyGallery Plugin:
Next, download the “FancyGallery” plugin from its official website or a trusted jQuery plugin repository. Extract the plugin files and include them in your project folder.
Step 3: Include the FancyGallery Plugin:
In the same <head> section of your HTML file, link to the FancyGallery plugin file after linking to the jQuery library.
html <!DOCTYPE html> <html> <head> <title>My Fancy Gallery</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="path/to/fancygallery.min.js"></script> </head> <body> <!-- Your gallery content goes here --> </body> </html>
Step 4: Set Up the Gallery Markup:
Create a container element where you want to display your image gallery. Give it a unique ID or class for easy targeting with jQuery.
html <!DOCTYPE html> <html> <head> <title>My Fancy Gallery</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="path/to/fancygallery.min.js"></script> </head> <body> <div id="myGallery"> <!-- Gallery images will be dynamically added here --> </div> </body> </html>
Step 5: Initialize the Gallery with jQuery:
After including the plugin and setting up the gallery markup, it’s time to initialize the FancyGallery plugin with jQuery. You can do this by writing a few lines of JavaScript code within a <script> tag, either in the <head> section or just before the closing </body> tag.
html <!DOCTYPE html> <html> <head> <title>My Fancy Gallery</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="path/to/fancygallery.min.js"></script> </head> <body> <div id="myGallery"> <!-- Gallery images will be dynamically added here --> </div> <script> $(document).ready(function() { $('#myGallery').fancyGallery(); }); </script> </body> </html>
4. Creating Custom jQuery Plugins:
While there are countless ready-made jQuery plugins available, you may find yourself in a situation where a specific plugin doesn’t exist for your unique requirements. In such cases, you can create your custom jQuery plugin. Let’s walk through the process of creating a simple “ShowMore” plugin, which allows you to show more content within a container when a button is clicked.
Step 1: Set Up the HTML Markup:
Create an HTML container element with some content that will be initially visible. Add a button within the container that users can click to reveal more content.
html <!DOCTYPE html> <html> <head> <title>ShowMore Plugin Example</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="path/to/showmore.min.js"></script> </head> <body> <div class="show-more-container"> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p> <button class="show-more-btn">Show More</button> <div class="hidden-content"> <p>More content here...</p> </div> </div> </body> </html>
Step 2: Create the ShowMore Plugin:
Now, let’s create the “ShowMore” plugin. The plugin will toggle the visibility of the hidden content when the button is clicked. To do this, add the following JavaScript code:
javascript (function($) { $.fn.showMore = function() { return this.each(function() { var $container = $(this); var $button = $container.find('.show-more-btn'); var $hiddenContent = $container.find('.hidden-content'); $button.on('click', function() { $hiddenContent.slideToggle(); $button.text(function(_, text) { return text === 'Show More' ? 'Show Less' : 'Show More'; }); }); }); }; })(jQuery);
Step 3: Initialize the ShowMore Plugin:
Finally, initialize the “ShowMore” plugin on the desired container. Add the following script just before the closing </body> tag:
html <!DOCTYPE html> <html> <head> <title>ShowMore Plugin Example</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="path/to/showmore.min.js"></script> </head> <body> <div class="show-more-container"> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p> <button class="show-more-btn">Show More</button> <div class="hidden-content"> <p>More content here...</p> </div> </div> <script> $(document).ready(function() { $('.show-more-container').showMore(); }); </script> </body> </html>
Conclusion
jQuery plugins are invaluable tools for web developers, providing a quick and efficient way to extend the functionality of websites. Whether you use existing plugins from the vast jQuery plugin repository or create custom plugins to suit your specific needs, jQuery’s extensibility empowers you to create interactive and engaging user experiences with ease. Embrace the power of jQuery plugins, and elevate your web development projects to new heights! Happy coding!
Table of Contents
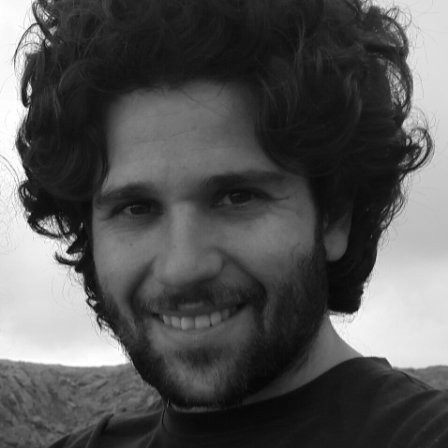
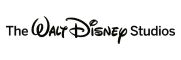