jQuery Magic: Unleashing the Full Potential of JavaScript Library
JavaScript is a powerful programming language that forms the backbone of interactive web development. It enables developers to create dynamic and engaging user experiences. However, working with raw JavaScript can sometimes be tedious and time-consuming, requiring extensive code to achieve desired functionalities. This is where jQuery comes in. jQuery is a popular JavaScript library that simplifies and enhances web development, allowing developers to write concise, efficient, and highly functional code. In this blog, we’ll explore the magic of jQuery and how it unleashes the full potential of JavaScript.
1. What is jQuery?
1.1 Understanding the Basics
jQuery is a fast, small, and feature-rich JavaScript library designed to simplify the client-side scripting of HTML. It provides a high-level API that abstracts complex JavaScript functions into simple and intuitive methods. By utilizing a combination of CSS-style selectors and DOM traversal methods, jQuery makes it easy to manipulate HTML elements, handle events, create animations, and perform AJAX requests.
1.2 Key Features of jQuery
Simplified DOM Manipulation
jQuery excels in simplifying DOM manipulation. With just a few lines of code, you can effortlessly select HTML elements using CSS-style selectors and perform various actions on them. Whether it’s updating content, adding or removing elements, changing styles, or handling events, jQuery provides an elegant syntax that greatly reduces the amount of code required compared to traditional JavaScript.
Code Sample 1:
javascript // Change the text of an element $("#myElement").text("Hello, jQuery!"); // Add a class to an element $(".myClass").addClass("highlight"); // Handle a click event $("button").click(function() { // Code to execute on button click });
AJAX Made Easy
One of jQuery’s most powerful features is its AJAX capabilities. It simplifies the process of making asynchronous HTTP requests, allowing you to fetch data from a server without refreshing the entire page. jQuery provides a set of methods, such as $.ajax() and $.get(), that handle the complexities of creating, sending, and processing AJAX requests, saving you from writing boilerplate code.
Code Sample 2:
javascript // Fetch data from a server using AJAX $.get("https://api.example.com/data", function(response) { // Process the received data console.log(response); });
Animation and Effects
jQuery makes it a breeze to add animation and effects to your web pages. With its built-in animation methods, you can easily create smooth transitions, fades, slides, and custom animations. Whether you want to animate an element’s position, opacity, or size, jQuery provides an intuitive and powerful interface for achieving stunning visual effects.
Code Sample 3:
javascript // Animate an element's width $("#myElement").animate({ width: "200px" }, 1000); // Fade out an element $(".myClass").fadeOut(500); // Slide up a div $("#myDiv").slideUp(300);
2. Advantages of Using jQuery
2.1 Simplicity and Productivity Boost
jQuery’s intuitive syntax and powerful API make it an excellent choice for both beginners and experienced developers. Its simplicity reduces the amount of code needed to accomplish common tasks, saving time and improving productivity. With jQuery, complex operations that would typically require multiple lines of JavaScript can be achieved in just a few concise statements.
2.2 Cross-Browser Compatibility
One of the biggest challenges in web development is ensuring that your code works consistently across different browsers. jQuery abstracts browser-specific inconsistencies and provides a unified interface, ensuring consistent behavior across various browsers. It takes care of the nitty-gritty details, allowing you to focus on building your application without worrying about cross-browser issues.
2.3 Extensibility and Plugins
jQuery’s modular architecture and extensive ecosystem of plugins make it highly extensible. You can enhance its capabilities by incorporating plugins for specialized functionality like data visualization, form validation, and more. Thousands of plugins are available, allowing you to tap into a wealth of community-contributed solutions that address common development challenges.
3. Getting Started with jQuery
3.1 Including jQuery in Your Project
To start using jQuery in your project, you need to include the jQuery library in your HTML file. You can either download the library and host it locally or use a Content Delivery Network (CDN) to fetch the library from a remote server. Simply add the following script tag to your HTML file:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
3.2 jQuery Selectors and DOM Manipulation
Selecting Elements
jQuery selectors allow you to target and manipulate specific elements on a web page. You can use various selectors, such as element names, class names, IDs, attribute values, and more. Selectors are similar to CSS selectors, making it easy to target elements in a familiar way.
Code Sample 4:
javascript // Select an element by its ID $("#myElement") // Select all elements with a specific class $(".myClass") // Select all paragraphs inside a div $("div p") // Select elements with a specific attribute value $("input[type='text']")
Manipulating Elements
Once you’ve selected elements, jQuery provides a wide range of methods to manipulate them. You can change their content, attributes, styles, and more. The text(), html(), val(), addClass(), removeClass(), and attr() methods are just a few examples of the powerful element manipulation capabilities offered by jQuery.
Code Sample 5:
javascript // Change the text of an element $("#myElement").text("New text"); // Change an element's attribute $("img").attr("src", "new-image.jpg"); // Add a class to an element $(".myElement").addClass("highlight"); // Modify the HTML content of an element $("#myDiv").html("<p>New content</p>");
Conclusion
jQuery is a versatile JavaScript library that empowers developers to create highly functional and interactive web applications with ease. Its simplicity, extensive features, and large community support make it a go-to choice for web developers worldwide. By leveraging the power of jQuery, you can unlock the full potential of JavaScript and streamline your development process. So, embrace the magic of jQuery and take your web development skills to the next level!
Table of Contents
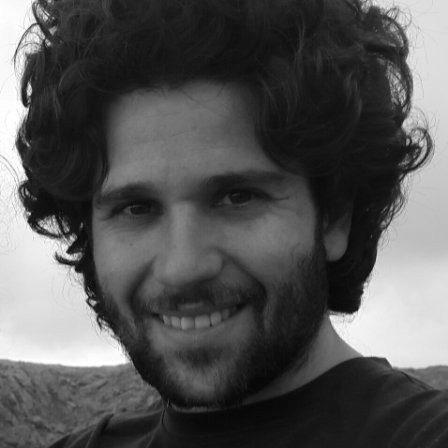
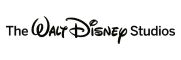