The Power of jQuery AJAX: Making Dynamic Web Requests
In the ever-evolving landscape of web development, creating dynamic and interactive web applications has become crucial to engage users effectively. One of the key technologies enabling such interactivity is AJAX (Asynchronous JavaScript and XML). With AJAX, web developers can send and receive data from the server asynchronously without the need for a page reload, leading to smoother user experiences.
Table of Contents
Among the various JavaScript libraries available, jQuery has been a favorite choice for web developers due to its simplicity, ease of use, and excellent AJAX capabilities. In this blog, we will explore the power of jQuery AJAX and how it can be used to make dynamic web requests, allowing you to create seamless and responsive web applications.
1. Understanding AJAX
1.1. What is AJAX?
AJAX is a web development technique that allows web pages to request and retrieve data from the server asynchronously in the background without interfering with the current page. It enables partial updates to a web page, reducing the need for full page reloads, and thereby enhancing the user experience. AJAX leverages a combination of technologies, including JavaScript, XML (although other formats like JSON are more common now), HTML, and CSS.
1.2. The Advantages of AJAX
AJAX offers several advantages that make it a powerful tool for web developers:
- Improved User Experience: AJAX enables real-time data retrieval and updates, leading to a more fluid and interactive user experience.
- Reduced Bandwidth Usage: By fetching only the necessary data from the server, AJAX reduces the amount of data transmitted, resulting in faster load times and reduced bandwidth consumption.
- Asynchronous Processing: AJAX requests are processed asynchronously, meaning the user can continue interacting with the page while the data is being retrieved or updated in the background.
- Selective Updates: Instead of refreshing the entire page, AJAX allows developers to update specific portions of the page, which helps maintain the user’s current context.
2. Getting Started with jQuery AJAX
To harness the power of AJAX in your web applications, jQuery provides a set of methods that make it incredibly easy to perform AJAX requests. Let’s explore the fundamental AJAX functions provided by jQuery:
2.1. $.ajax()
The $.ajax() method is the core of jQuery’s AJAX capabilities. It allows you to configure and execute AJAX requests with various options and callbacks. Here’s a basic example of making a simple GET request using $.ajax():
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', success: function(data) { // Handle the response data here console.log(data); }, error: function(xhr, status, error) { // Handle errors here console.error(error); } });
In the example above, we specify the URL to fetch data from, the HTTP method (GET, POST, PUT, DELETE, etc.), and provide success and error callbacks to handle the response or any errors that may occur during the request.
2.2. $.get() and $.post()
For simple GET and POST requests, jQuery provides shorthand methods – $.get() and $.post():
javascript // Using $.get() $.get('https://api.example.com/data', function(data) { // Handle the response data here console.log(data); }); // Using $.post() $.post('https://api.example.com/data', { key: 'value' }, function(data) { // Handle the response data here console.log(data); });
These methods are concise and perfect for straightforward requests where you don’t need extensive configuration.
2.3. $.getJSON()
When dealing with JSON data, the $.getJSON() method can be used to fetch JSON data from the server:
javascript $.getJSON('https://api.example.com/data', function(data) { // Handle the JSON response data here console.log(data); });
2.4. Specifying Additional Parameters
Often, you may need to pass additional parameters with your AJAX requests, such as query parameters or data for POST requests. You can achieve this using the data property:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'POST', data: { key1: 'value1', key2: 'value2' }, success: function(data) { // Handle the response data here console.log(data); }, error: function(xhr, status, error) { // Handle errors here console.error(error); } });
In this example, we’re sending a POST request with two data parameters, key1 and key2, to the specified URL.
3. Handling AJAX Responses
Once the AJAX request is made, handling the server’s response becomes crucial. jQuery provides simple mechanisms to work with the response data.
3.1. Handling JSON Data
When dealing with JSON responses, which are quite common in modern web APIs, you can directly access the parsed JSON data in the success callback:
javascript $.getJSON('https://api.example.com/data', function(data) { // Handle the JSON response data here console.log(data); });
The data variable in the example above contains the parsed JSON object.
3.2. Handling XML Data
If the server returns XML data instead, jQuery provides the $.parseXML() method to parse the XML response:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', dataType: 'xml', success: function(data) { // Handle the parsed XML data here console.log(data); }, error: function(xhr, status, error) { // Handle errors here console.error(error); } });
The dataType: ‘xml’ option ensures that the response is parsed as XML.
3.3. Handling Error Responses
Handling errors gracefully is essential to provide a seamless user experience. In the examples above, we’ve included error callback functions to handle error responses. The error callback receives three arguments: the XMLHttpRequest object, a string describing the type of error, and an optional exception object if available.
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', success: function(data) { // Handle the response data here console.log(data); }, error: function(xhr, status, error) { // Handle errors here console.error(error); } });
4. AJAX and Asynchronous Behavior
AJAX requests are asynchronous by nature, meaning they don’t block the execution of other scripts or the user interface during the request. Instead, the browser continues to execute scripts, allowing users to interact with the page while the AJAX request is being processed in the background.
4.1. Callbacks for Asynchronous Operations
Since AJAX operations complete asynchronously, it’s essential to use callbacks or promises to handle the response data correctly. In the previous examples, we’ve used success and error callbacks to process the data or handle errors.
4.2. Using Promises with AJAX
Alternatively, jQuery provides a Promise interface that can be used to handle AJAX responses. The $.ajax() method returns a Promise object, allowing you to use .then() and .catch() for success and error handling, respectively:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET' }) .then(function(data) { // Handle the response data here console.log(data); }) .catch(function(error) { // Handle errors here console.error(error); });
Using promises can lead to cleaner and more readable code when dealing with complex AJAX requests.
Conclusion
In this blog, we’ve explored the power of jQuery AJAX and how it empowers web developers to create dynamic and interactive web applications. AJAX has revolutionized the way data is fetched and updated in web pages, significantly enhancing the user experience. By leveraging jQuery’s AJAX capabilities, developers can seamlessly interact with servers, retrieve data, and update content without interrupting the user’s flow.
Remember, while AJAX provides immense flexibility and potential, it’s essential to use it judiciously to maintain a balance between user experience and server load. With the right implementation, jQuery AJAX can take your web applications to new heights of interactivity and responsiveness. Happy coding!
Table of Contents
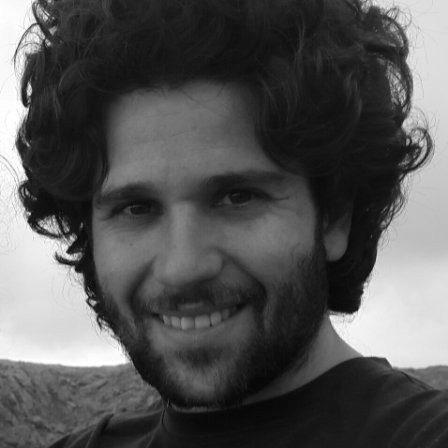
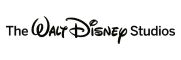