An Introduction to jQuery Promises: Managing Asynchronous Operations
In the world of web development, handling asynchronous operations is a common and often challenging task. Whether you’re fetching data from an API, animating elements on a page, or waiting for user input, JavaScript provides a set of tools to work with asynchronous code. One such tool is jQuery Promises, a powerful mechanism for managing and organizing asynchronous operations. In this blog, we’ll introduce you to jQuery Promises, exploring their basics, syntax, and practical examples to help you harness their potential.
Table of Contents
1. Understanding Asynchronous Operations
Before delving into jQuery Promises, let’s briefly understand what asynchronous operations are and why they are important in web development.
1.1. What Are Asynchronous Operations?
In web development, asynchronous operations are tasks that don’t necessarily happen sequentially. Instead, they occur independently of the main program flow. Common examples include making network requests, reading/writing files, and handling user interactions.
1.2. Why Asynchronous Operations Matter
Asynchronous operations are crucial for building responsive and efficient web applications. Without them, tasks like fetching data from an API would block the entire application, leading to a poor user experience. Asynchronous operations allow your application to perform multiple tasks concurrently, ensuring that the user interface remains responsive.
Now that we understand the importance of asynchronous operations, let’s explore how jQuery Promises can simplify working with them.
2. Introducing jQuery Promises
jQuery Promises are a way to handle asynchronous operations in a more organized and predictable manner. They provide a structured approach to managing asynchronous tasks and handling their outcomes. Promises are part of the jQuery library and are also available as a standalone JavaScript feature called ES6 Promises.
2.1. The Promise Lifecycle
A Promise in jQuery has three possible states:
- Pending: This is the initial state when a Promise is created. It represents that the operation is still in progress.
- Resolved: The Promise transitions to this state when the operation is successfully completed. This state is also known as “fulfilled.”
- Rejected: If an error occurs during the operation, the Promise transitions to the rejected state. It signifies that the operation failed.
2.2. Creating a Promise
To create a Promise in jQuery, you use the $.Deferred() method. Here’s an example of creating a simple Promise that simulates fetching data from a server:
javascript function fetchData() { var deferred = $.Deferred(); // Simulate an asynchronous operation setTimeout(function () { var data = { message: "Data fetched successfully" }; deferred.resolve(data); // Resolve the Promise with data }, 2000); return deferred.promise(); } // Using the Promise fetchData().done(function (data) { console.log(data.message); // Output: Data fetched successfully });
In this example, fetchData creates a Promise that resolves after a simulated asynchronous operation (a 2-second timeout).
2.3. Chaining Promises
One of the key benefits of Promises is the ability to chain them together. This allows you to sequence asynchronous operations and handle their results in a more structured way. Here’s an example of chaining Promises:
javascript function fetchUserData() { var deferred = $.Deferred(); // Simulate fetching user data setTimeout(function () { var userData = { username: "john_doe" }; deferred.resolve(userData); }, 1000); return deferred.promise(); } function fetchPosts(username) { var deferred = $.Deferred(); // Simulate fetching user posts setTimeout(function () { var posts = ["Post 1", "Post 2", "Post 3"]; deferred.resolve(posts); }, 1500); return deferred.promise(); } fetchUserData() .then(function (userData) { console.log("User Data:", userData); return fetchPosts(userData.username); }) .then(function (posts) { console.log("User Posts:", posts); });
In this example, we first fetch user data and then use that data to fetch user posts. The .then() method is used to chain these two asynchronous operations.
3. Handling Errors with Promises
In real-world applications, errors can occur during asynchronous operations. jQuery Promises provide a convenient way to handle errors using the .fail() method.
javascript function fetchData() { var deferred = $.Deferred(); // Simulate an error during the operation setTimeout(function () { deferred.reject("An error occurred while fetching data."); }, 2000); return deferred.promise(); } fetchData() .done(function (data) { console.log("Success:", data); }) .fail(function (error) { console.error("Error:", error); });
In this example, the fetchData function intentionally rejects the Promise to simulate an error. The .fail() method is then used to handle the error.
4. Combining Multiple Promises
There are scenarios where you need to perform multiple asynchronous operations in parallel and wait for all of them to complete. jQuery provides the .when() method for this purpose.
javascript var promise1 = $.ajax({ url: "api/endpoint1" }); var promise2 = $.ajax({ url: "api/endpoint2" }); var promise3 = $.ajax({ url: "api/endpoint3" }); $.when(promise1, promise2, promise3).done(function (data1, data2, data3) { console.log("Data from endpoint 1:", data1); console.log("Data from endpoint 2:", data2); console.log("Data from endpoint 3:", data3); });
In this example, we have three AJAX requests represented as individual Promises. The .when() method is used to wait for all three Promises to resolve.
5. Promises in Modern JavaScript
While jQuery Promises are a valuable tool, it’s important to note that modern JavaScript (ES6 and beyond) includes native Promise support. Here’s how you can create and use Promises without jQuery:
javascript function fetchData() { return new Promise(function (resolve, reject) { // Simulate an asynchronous operation setTimeout(function () { var data = { message: "Data fetched successfully" }; resolve(data); }, 2000); }); } fetchData() .then(function (data) { console.log(data.message); }) .catch(function (error) { console.error(error); });
ES6 Promises provide similar functionality to jQuery Promises and are supported in all modern browsers.
Conclusion
In the world of web development, managing asynchronous operations is a fundamental skill. jQuery Promises offer a structured and organized way to handle these operations, making your code more readable and maintainable. Understanding the basics of Promises, their lifecycle, and how to handle errors is essential for building robust web applications.
As you continue your journey in web development, consider exploring other asynchronous techniques and libraries, such as async/await in modern JavaScript or the Fetch API for making network requests. Combining these tools with jQuery Promises can help you build powerful and responsive web applications that provide a seamless user experience.
Remember, the key to mastering Promises is practice. So, start experimenting with asynchronous operations in your projects and harness the full potential of jQuery Promises to create outstanding web applications.
If you found this introduction to jQuery Promises helpful, stay tuned for more in-depth tutorials and practical examples to level up your web development skills. Happy coding!
Table of Contents
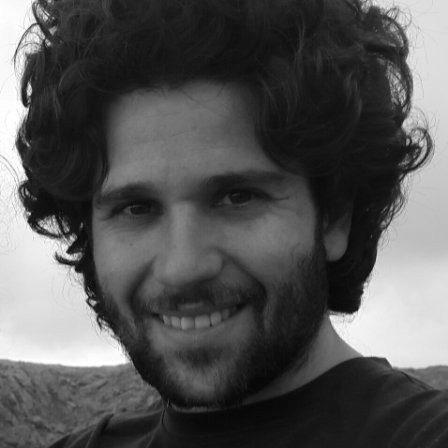
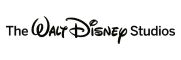