Building Real-Time Applications with jQuery and WebSockets
In today’s rapidly evolving digital landscape, user engagement and interactivity play a crucial role in determining the success of web applications. Traditional web development, reliant on request-response cycles, often falls short when it comes to creating real-time and dynamic experiences. This is where the combination of jQuery and WebSockets comes into play. In this blog post, we’ll delve into the world of building real-time applications using jQuery and WebSockets, exploring their capabilities, advantages, and a step-by-step guide to implement them effectively.
Table of Contents
1. Understanding Real-Time Web Applications
1.1. The Limitations of Traditional Web Development
Traditional web development follows a request-response model, where the client sends a request to the server, and the server responds with the requested data. While this model has served its purpose well, it falls short when it comes to creating real-time applications that require constant updates without the need for continuous requests. Imagine live collaborative tools, stock market trackers, and online gaming platforms; these scenarios demand real-time interactions that traditional approaches struggle to provide efficiently.
1.2. Introducing WebSockets for Real-Time Communication
WebSockets emerged as a solution to this problem. Unlike the traditional request-response model, WebSockets provide full-duplex communication channels, allowing both the client and server to send and receive data at any time. This persistent connection eliminates the need for frequent requests and provides a seamless way to achieve real-time communication. WebSockets overcome the limitations of techniques like polling and long polling, providing a more efficient and responsive solution.
2. Leveraging jQuery for Enhanced User Interactivity
2.1. Simplifying DOM Manipulation with jQuery
jQuery, a lightweight JavaScript library, has long been a favorite among developers for its ability to simplify DOM manipulation and interaction. With just a few lines of code, jQuery enables you to traverse and manipulate the DOM tree, making it easier to create dynamic and interactive web applications. By targeting HTML elements and applying changes on the fly, jQuery minimizes the complexity of traditional JavaScript coding.
2.2. Handling Events and Animations Effortlessly
One of the standout features of jQuery is its event handling capabilities. Instead of manually attaching event listeners to HTML elements, jQuery allows you to use concise and intuitive syntax to bind actions to events. This significantly reduces the amount of code you need to write while maintaining clarity and readability. Additionally, jQuery’s animation methods facilitate the creation of smooth and engaging animations that can enhance the user experience of your real-time applications.
3. Unleashing the Power of WebSockets with jQuery
3.1. Setting Up a WebSockets Server
Before diving into jQuery integration, it’s essential to set up a WebSockets server. Several technologies, such as Node.js with libraries like socket.io, enable you to establish a server that supports WebSockets communication. The server will be responsible for handling incoming WebSocket connections, managing data transmission, and facilitating real-time communication between clients.
3.2. Establishing a WebSockets Connection
Once the server is up and running, it’s time to establish a WebSocket connection from the client-side using jQuery. jQuery simplifies this process by providing a clean API for creating WebSocket connections. By using the WebSocket API or a library like reconnecting-websocket, you can initiate a connection and specify event handlers to manage the different stages of the connection’s lifecycle.
javascript // Establishing a WebSocket connection using jQuery const socket = new WebSocket('ws://your-websocket-server'); socket.addEventListener('open', () => { console.log('WebSocket connection established'); }); socket.addEventListener('message', (event) => { const data = JSON.parse(event.data); console.log('Received:', data); }); socket.addEventListener('close', (event) => { console.log('WebSocket connection closed', event.code); });
3.3. Sending and Receiving Real-Time Data
Once the connection is established, you can send and receive real-time data between the client and the server. JSON is commonly used to structure data for transmission. jQuery’s simplicity shines here, as sending data is as straightforward as calling the send method on the WebSocket instance.
javascript // Sending real-time data from the client const dataToSend = { message: 'Hello, server!' }; socket.send(JSON.stringify(dataToSend));
On the server side, you can listen for incoming messages, process the data, and respond accordingly.
4. Building a Real-Time Chat Application
4.1. Designing the User Interface
Let’s put the power of jQuery and WebSockets into practice by building a real-time chat application. The first step is designing the user interface. Use HTML and CSS to create a visually appealing chat box where users can see incoming and outgoing messages.
html <!DOCTYPE html> <html> <head> <title>Real-Time Chat</title> <link rel="stylesheet" type="text/css" href="styles.css"> </head> <body> <div id="chat-box"></div> <input type="text" id="message-input" placeholder="Type your message..."> <button id="send-button">Send</button> <script src="jquery.min.js"></script> <script src="websocket-client.js"></script> </body> </html>
4.2. Implementing WebSockets for Instant Messaging
In the websocket-client.js file, establish a WebSocket connection and handle incoming messages. Display these messages in the chat box to create a real-time messaging experience.
javascript // websocket-client.js $(document).ready(() => { const socket = new WebSocket('ws://your-websocket-server'); socket.addEventListener('message', (event) => { const data = JSON.parse(event.data); const messageBox = $('#chat-box'); const messageElement = $('<div class="message"></div>'); messageElement.text(data.message); messageBox.append(messageElement); }); $('#send-button').click(() => { const inputElement = $('#message-input'); const message = inputElement.val(); if (message) { const dataToSend = { message }; socket.send(JSON.stringify(dataToSend)); inputElement.val(''); } }); });
4.3. Enhancing the User Experience with jQuery
To enhance the user experience, consider adding features like auto-scrolling to the latest message, displaying the sender’s name, and incorporating animations for message transitions. jQuery’s animations and effects can bring the chat interface to life, making the real-time interaction even more engaging.
5. Enhancing Real-Time Applications for Scalability
5.1. Load Balancing and Horizontal Scaling
As your real-time application gains popularity, it’s crucial to ensure scalability to handle a growing number of users. Load balancing and horizontal scaling become necessary to distribute the incoming WebSocket connections across multiple server instances. Technologies like NGINX can be used as a load balancer to evenly distribute incoming connections among different server instances.
5.2. Handling Failures and Reconnections
Real-time applications should be resilient in the face of failures. Implement mechanisms to handle WebSocket connection drops and unexpected server failures gracefully. Libraries like reconnecting-websocket automatically manage reconnection attempts, helping maintain a seamless user experience even in unstable network conditions.
Conclusion
The combination of jQuery and WebSockets opens up exciting possibilities for building dynamic and real-time web applications. By leveraging jQuery’s simplicity for DOM manipulation and event handling alongside the power of WebSockets for efficient real-time communication, developers can create engaging user experiences that were once challenging to achieve. Whether it’s a live chat application, collaborative tool, or real-time dashboard, the fusion of these technologies empowers developers to elevate their projects and deliver exceptional real-time interactions. So, dive into the world of jQuery and WebSockets, and unlock the potential of real-time web applications like never before.
Table of Contents
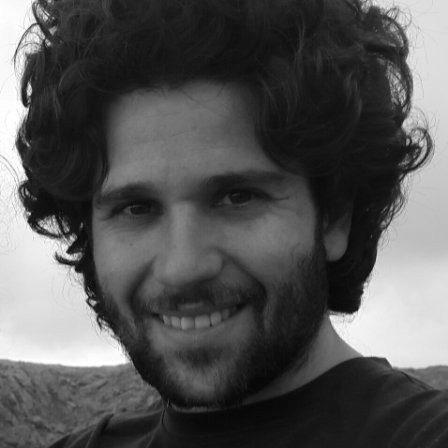
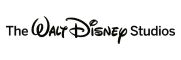