Developing Real-Time Chat Applications with jQuery and WebSocket
In today’s fast-paced digital world, real-time communication has become an integral part of our lives. From instant messaging to collaborative workspaces, the need for interactive and engaging chat applications is ever-growing. In this tutorial, we’ll explore how to develop real-time chat applications using the power of jQuery and WebSocket technology. By the end of this guide, you’ll be equipped with the skills to create seamless and dynamic chat platforms that provide users with an immersive communication experience.
Table of Contents
1. Why jQuery and WebSockets?
Before we dive into the technical details, let’s briefly discuss why jQuery and WebSocket technology are excellent choices for developing real-time chat applications.
- jQuery: jQuery is a popular JavaScript library that simplifies HTML document manipulation, event handling, and AJAX interactions. Its intuitive syntax and wide range of plugins make it an ideal choice for creating dynamic and interactive web applications.
- WebSocket: WebSocket is a communication protocol that provides full-duplex communication channels over a single TCP connection. Unlike traditional HTTP requests, WebSocket enables bidirectional, low-latency communication between the client (browser) and the server. This makes it perfect for real-time applications like chat platforms where instantaneous updates are crucial.
2. Getting Started: Setting Up the Project
Before we start coding, let’s set up the project structure and necessary files.
Create a New Project Folder: Begin by creating a new folder for your project. Name it something like “RealTimeChatApp”.
HTML Structure: Inside the project folder, create an index.html file and set up the basic HTML structure.
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Real-Time Chat Application</title> <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <div id="chat-container"> <div id="chat-messages"></div> <input type="text" id="message-input" placeholder="Type your message..."> <button id="send-button">Send</button> </div> <!-- Include WebSocket script --> <script> // WebSocket code will be added here </script> </body> </html>
3. Setting Up the WebSocket Connection:
To establish a WebSocket connection between the client and the server, you’ll need to use JavaScript’s built-in WebSocket object. Let’s create a script to handle this connection.
Create a WebSocket Instance: Inside the <script> tag in your index.html file, add the following code to create a WebSocket connection.
javascript <script> const socket = new WebSocket('ws://your-server-address'); </script>
Replace ‘ws://your-server-address’ with the actual WebSocket server address you’ll be using.
WebSocket Event Listeners: Now, let’s set up event listeners to handle different WebSocket events.
javascript <script> const socket = new WebSocket('ws://your-server-address'); // Event listener for connection open socket.addEventListener('open', (event) => { console.log('WebSocket connection opened'); }); // Event listener for receiving messages socket.addEventListener('message', (event) => { const message = event.data; // Process and display the message }); // Event listener for connection close socket.addEventListener('close', (event) => { console.log('WebSocket connection closed'); }); // Event listener for connection errors socket.addEventListener('error', (event) => { console.error('WebSocket error:', event.error); }); </script>
4. Sending and Receiving Messages:
With the WebSocket connection established and event listeners in place, it’s time to enable users to send and receive messages in real-time.
Sending Messages: Add the following code to send messages when the “Send” button is clicked.
javascript <script> const socket = new WebSocket('ws://your-server-address'); // ... Event listeners (as shown above) ... // Send button click event $('#send-button').click(() => { const messageInput = $('#message-input').val(); if (messageInput.trim() !== '') { socket.send(messageInput); $('#message-input').val(''); } }); </script>
Receiving Messages: Modify the existing message event listener to display received messages.
javascript <script> const socket = new WebSocket('ws://your-server-address'); // ... Other event listeners ... // Event listener for receiving messages socket.addEventListener('message', (event) => { const message = event.data; displayMessage(message); }); function displayMessage(message) { const messageElement = `<div class="message">${message}</div>`; $('#chat-messages').append(messageElement); } </script>
5. Styling and Enhancing the User Experience:
While the basic functionality is in place, adding some CSS styles and enhancing the user experience will make your chat application more appealing.
CSS Styling: Create a new CSS file named styles.css in your project folder and link it in your index.html file.
html <head> <!-- ... Other meta tags and scripts ... --> <link rel="stylesheet" href="styles.css"> </head>
In your styles.css file, you can add styling to improve the visual appeal of your chat interface.
css #chat-container { width: 400px; margin: auto; padding: 20px; border: 1px solid #ccc; box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1); } #chat-messages { height: 300px; overflow-y: scroll; border-bottom: 1px solid #ccc; padding-bottom: 10px; } .message { background-color: #f2f2f2; padding: 5px; margin: 5px 0; border-radius: 5px; }
Conclusion
In this tutorial, we’ve explored the process of developing real-time chat applications using jQuery and WebSocket technology. By combining the power of jQuery’s simplicity and WebSocket’s bidirectional communication, you can create dynamic and interactive chat platforms that provide users with a seamless communication experience. From setting up the project structure to establishing WebSocket connections and enabling message sending and receiving, you now have the foundation to build engaging chat applications that meet the demands of modern communication.
Remember that this tutorial is just the beginning. As you delve deeper into the world of web development, you can further enhance your chat application by adding features like user authentication, message persistence, and multimedia support. Whether you’re building internal team communication tools or social networking platforms, the skills you’ve acquired here will serve as a strong foundation for your real-time application development journey. Happy coding!
Table of Contents
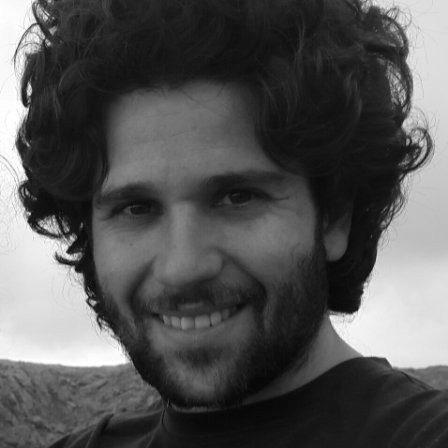
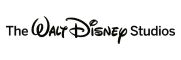