Building Responsive Accordions with jQuery
In today’s web development landscape, creating a seamless and engaging user experience is paramount. One effective way to achieve this is by using accordions. Accordions allow you to organize your content in a compact, collapsible manner, reducing clutter and enhancing user navigation. In this tutorial, we will explore how to build responsive accordions using jQuery, a versatile and widely-used JavaScript library. Whether you’re a seasoned developer or just getting started, this guide will help you create interactive accordions that adapt seamlessly to different screen sizes.
Table of Contents
1. Understanding Accordions:
1.1. What are Accordions?
Accordions are UI components that allow you to present content in a collapsible manner, often consisting of headings and their associated content. When a user clicks on a heading, the corresponding content expands or collapses, providing an organized way to display information.
1.2. Benefits of Using Accordions:
- Space Efficiency: Accordions are perfect for presenting a lot of content in a limited space, particularly useful for mobile devices.
- User-Friendly: Users can choose to engage with the content they are interested in, reducing cognitive overload.
- Visual Appeal: Accordions provide a neat and structured appearance to your web pages.
- Interactive Experience: Animations and transitions make the interaction smooth and engaging.
2. Getting Started with jQuery:
2.1. Linking jQuery in HTML:
Before we dive into building our responsive accordion, we need to include jQuery in our project. You can either download jQuery and host it locally or include it from a Content Delivery Network (CDN). Here’s an example of including jQuery from a CDN:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Responsive Accordions with jQuery</title> <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head> <body> <!-- Your content goes here --> </body> </html>
2.2. Understanding the Document Ready Event:
To ensure that your jQuery code runs after the DOM (Document Object Model) is fully loaded, use the $(document).ready() function. This prevents any code from executing before the HTML elements are ready to be manipulated.
javascript $(document).ready(function() { // Your jQuery code here });
3. Building the HTML Structure:
3.1. Container Setup:
Let’s start by creating the basic HTML structure for our accordion. We’ll use a list-based approach, where each list item represents an accordion item. Each item will consist of a heading and its corresponding content.
html <div class="accordion"> <ul> <li> <h2>Section 1</h2> <div class="content"> <p>Content for Section 1 goes here.</p> </div> </li> <li> <h2>Section 2</h2> <div class="content"> <p>Content for Section 2 goes here.</p> </div> </li> <!-- Add more sections as needed --> </ul> </div>
3.2. Item Structure:
Each list item (<li>) represents a section in the accordion. It contains a heading (<h2>) and a content container (<div class=”content”>) for the actual content associated with the section.
4. Styling Your Accordions:
4.1. Basic Styling:
To make your accordion visually appealing, you can apply basic CSS styles. Here’s a simple example to get you started:
css /* Reset default list styles */ .accordion ul { list-style: none; padding: 0; margin: 0; } /* Style for accordion headings */ .accordion h2 { background-color: #f2f2f2; padding: 10px; margin: 0; cursor: pointer; } /* Style for accordion content */ .accordion .content { display: none; padding: 10px; }
4.2. Adding Transitions for Smooth Animations:
Adding smooth animations to your accordion enhances the user experience. You can achieve this by using CSS transitions. Here’s how you can modify the styles to include transitions:
css /* Adding transitions for accordion content */ .accordion .content { display: none; padding: 10px; overflow: hidden; max-height: 0; transition: max-height 0.3s ease-in-out; }
5. Writing jQuery for Accordions:
5.1. Selecting Elements:
In the $(document).ready() function, you can start by selecting the elements you want to interact with. In our case, we want to select the accordion headings and their associated content.
javascript $(document).ready(function() { // Select accordion headings and content var accordionHeaders = $('.accordion h2'); var accordionContent = $('.accordion .content'); });
5.2. Adding Click Event:
Next, you’ll want to add a click event to the accordion headings. This event will trigger the expansion or collapse of the content when a heading is clicked.
javascript $(document).ready(function() { var accordionHeaders = $('.accordion h2'); var accordionContent = $('.accordion .content'); // Add click event to accordion headings accordionHeaders.click(function() { // Your code to toggle content here }); });
5.3. Toggling Accordion Items:
Inside the click event function, you can toggle the visibility of the associated content by using jQuery’s slideToggle() function. This function smoothly animates the height of the content, creating a collapsible effect.
javascript $(document).ready(function() { var accordionHeaders = $('.accordion h2'); var accordionContent = $('.accordion .content'); accordionHeaders.click(function() { // Toggle the content visibility $(this).next(accordionContent).slideToggle(); }); });
6. Implementing Responsiveness:
6.1. Media Queries for Mobile Devices:
To ensure that your accordions are responsive and adapt to different screen sizes, you can use CSS media queries. These queries allow you to apply different styles based on the screen width.
css /* Media query for screens up to 768px wide */ @media (max-width: 768px) { .accordion h2 { font-size: 18px; /* Adjust font size for mobile */ } }
6.2. Adapting Accordion for Smaller Screens:
To optimize the accordion for smaller screens, you can modify the jQuery code to ensure that only one section is open at a time. This prevents excessive scrolling and enhances the user experience on mobile devices.
javascript $(document).ready(function() { var accordionHeaders = $('.accordion h2'); var accordionContent = $('.accordion .content'); accordionHeaders.click(function() { // Close all other open sections accordionContent.not($(this).next()).slideUp(); // Toggle the clicked section's content $(this).next(accordionContent).slideToggle(); }); });
Conclusion
In this tutorial, we’ve explored how to create responsive accordions using jQuery. By following the step-by-step guide, you’ve learned how to build a structured HTML layout, style your accordions, write jQuery code to make them interactive, and implement responsiveness for various screen sizes. Accordions not only enhance the user experience by presenting content in an organized manner but also contribute to a visually appealing and efficient web design. As you continue to develop your web development skills, remember that accordions are just one of the many tools at your disposal to create engaging and user-friendly interfaces.
Remember, practice makes perfect, so feel free to experiment with different styles and functionality to make your responsive accordions truly stand out in your web projects. Happy coding!
Table of Contents
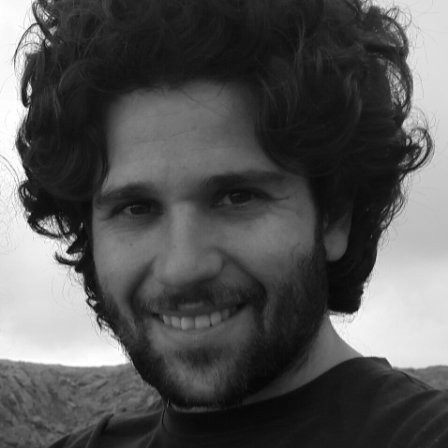
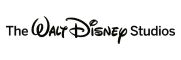