Building Responsive Data Tables with jQuery DataTables
Data tables are a fundamental component of modern web applications. They are used to display data in a structured and organized manner, making it easier for users to interpret and analyze information. However, creating responsive data tables that work seamlessly across various devices and screen sizes can be a challenging task. That’s where jQuery DataTables come into play. In this comprehensive guide, we will walk you through the process of building responsive data tables using jQuery DataTables.
Table of Contents
1. What is jQuery DataTables?
jQuery DataTables is a powerful and flexible JavaScript library that simplifies the process of creating interactive and feature-rich data tables for your web applications. It offers a wide range of functionalities, including sorting, searching, pagination, and more, out of the box. One of its most significant advantages is its responsiveness, which ensures that your data tables look and function well on both desktop and mobile devices.
2. Getting Started
Before we dive into creating responsive data tables, you need to include the jQuery DataTables library in your project. You can download it from the official DataTables website, or you can include it via a content delivery network (CDN) like this:
html <!DOCTYPE html> <html> <head> <!-- Include jQuery DataTables CSS --> <link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/1.11.6/css/jquery.dataTables.css"> <!-- Include jQuery --> <script type="text/javascript" charset="utf8" src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery DataTables JS --> <script type="text/javascript" charset="utf8" src="https://cdn.datatables.net/1.11.6/js/jquery.dataTables.js"></script> </head> <body> <!-- Your HTML content here --> </body> </html>
Now that you have jQuery DataTables integrated into your project, let’s move on to creating your first responsive data table.
3. Creating a Basic Data Table
Let’s start with a basic example of creating a data table. Suppose you have a simple HTML table like this:
html <table id="example" class="display" style="width:100%"> <thead> <tr> <th>Name</th> <th>Age</th> <th>City</th> </tr> </thead> <tbody> <tr> <td>John Doe</td> <td>30</td> <td>New York</td> </tr> <tr> <td>Jane Smith</td> <td>28</td> <td>Los Angeles</td> </tr> <!-- Add more rows as needed --> </tbody> </table>
To turn this static HTML table into a responsive jQuery DataTable, follow these steps:
Initialize the DataTable: In your JavaScript code, add the following lines to initialize the DataTable:
javascript $(document).ready(function() { $('#example').DataTable(); });
Add the DataTable CSS class: In your HTML table, make sure to include the display class, which is required for DataTables to function properly.
html <table id="example" class="display" style="width:100%">
That’s it! You now have a basic data table that is sortable, searchable, and pageable. However, it’s not responsive yet. To make it responsive, you need to make some adjustments to your table and add a few configurations.
4. Making the Data Table Responsive
To ensure that your data table adapts to different screen sizes and remains user-friendly on mobile devices, you can make use of DataTables’ responsive extension. Here’s how you can do it:
Include the Responsive CSS and JavaScript files:
html <!-- Include DataTables Responsive CSS --> <link rel="stylesheet" type="text/css" href="https://cdn.datatables.net/responsive/2.2.9/css/responsive.dataTables.css"> <!-- Include DataTables Responsive JS --> <script type="text/javascript" charset="utf8" src="https://cdn.datatables.net/responsive/2.2.9/js/dataTables.responsive.js"></script>
Modify the DataTable initialization code to enable responsiveness:
javascript $(document).ready(function() { $('#example').DataTable({ responsive: true }); });
With these changes, your data table is now responsive. When the screen size is reduced, the table will automatically hide columns and provide a horizontal scrollbar for navigation, ensuring a smooth user experience on smaller screens.
5. Customizing Responsive Behavior
While DataTables’ default responsive behavior is usually sufficient, you can customize it further to meet your specific requirements. Here are some common customizations:
5.1. Specify Which Columns to Show
You can specify which columns should remain visible on small screens using the responsivePriority option. For example, to always display the “Name” and “Age” columns, add the following to your DataTable initialization code:
javascript $(document).ready(function() { $('#example').DataTable({ responsive: true, columnDefs: [ { responsivePriority: 1, targets: 0 }, // Name column { responsivePriority: 2, targets: 1 } // Age column ] }); });
In this example, the “Name” column is given the highest priority, followed by the “Age” column. These columns will remain visible on small screens, while others may be hidden.
5.2. Disable Responsive Mode for Larger Screens
If you want to disable responsive mode entirely for larger screens, you can use the responsive option in combination with the responsive: “false” value for specific breakpoints. For instance, to disable responsiveness for screens wider than 768 pixels, use the following code:
javascript $(document).ready(function() { $('#example').DataTable({ responsive: { details: false } }); });
This code ensures that the responsive behavior is only active for screens narrower than 768 pixels.
5.3. Custom Column Priority
You can also set custom priorities for columns using JavaScript functions based on your application’s logic. For example, you might want to prioritize columns differently depending on the content or context of your table.
javascript $(document).ready(function() { $('#example').DataTable({ responsive: { details: { type: 'column', target: -1 }, columnDefs: [ { className: 'control', orderable: false, targets: -1 } ] }, order: [1, 'asc'] }); });
In this example, the responsive.details option specifies that the column with the highest priority (target -1) should always be shown. You can use the targets property to specify which column(s) this applies to.
6. Advanced Features
jQuery DataTables offers a plethora of advanced features to enhance the functionality and appearance of your data tables further. Let’s explore some of these features:
6.1. Sorting and Searching
By default, DataTables allows users to sort columns by clicking on the column headers and search for specific data in the table. These features are essential for data organization and retrieval.
6.2. Pagination
If your data table contains a large dataset, you can enable pagination to display a limited number of rows per page, improving page load times and user experience.
javascript $(document).ready(function() { $('#example').DataTable({ paging: true // Enable pagination }); });
6.3. Exporting Data
You can enable data export options that allow users to download the table data in various formats, such as CSV, Excel, or PDF.
javascript $(document).ready(function() { $('#example').DataTable({ buttons: ['csv', 'excel', 'pdf'] // Enable export buttons }); });
6.4. Custom Styling
You can customize the styling of your data table to match your website’s design by modifying DataTables’ CSS classes or using custom CSS.
6.5. Server-Side Processing
For handling large datasets efficiently, you can implement server-side processing, where DataTables interacts with your server to fetch and display data on-demand.
javascript $(document).ready(function() { $('#example').DataTable({ processing: true, serverSide: true, ajax: '/your-server-endpoint' }); });
Conclusion
Creating responsive data tables with jQuery DataTables is a powerful way to enhance the user experience of your web applications. With its built-in features and flexibility, you can easily create tables that work seamlessly across different devices and screen sizes. Whether you need a basic table or a highly customized, interactive one, jQuery DataTables has you covered. Start using it today to take your data presentation to the next level.
In this guide, we covered the basics of setting up and customizing responsive data tables with jQuery DataTables. However, jQuery DataTables offers even more advanced options and features to explore, so be sure to check out the official documentation for in-depth information and examples.
Now that you have the knowledge to build responsive data tables, go ahead and implement them in your web projects to provide a better user experience and make your data more accessible and organized.
Happy coding!
Table of Contents
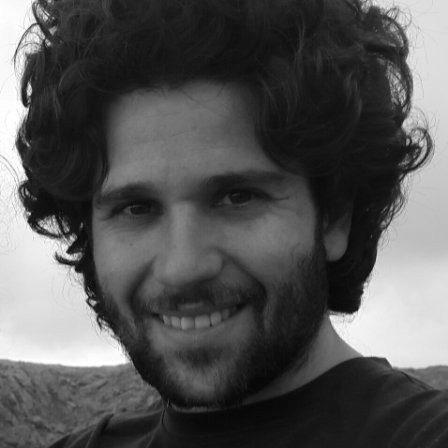
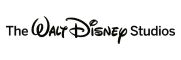