jQuery and Responsive Design: Adapting to Different Devices
In today’s digital landscape, where users access websites and applications on a plethora of devices, ensuring a seamless user experience across all screen sizes is paramount. Responsive design is the key to achieving this goal, and jQuery, a powerful JavaScript library, plays a significant role in enhancing responsive web development. In this article, we will delve into the world of jQuery and responsive design, exploring how jQuery can be used to adapt web content effectively to different devices.
Table of Contents
1. Understanding Responsive Design
Responsive design is an approach to web design that focuses on creating websites and applications that adapt and respond to the user’s environment, irrespective of the device they are using. With the advent of smartphones, tablets, and a wide array of screen sizes, building a static website that looks good only on a desktop monitor is no longer sufficient. Responsive design ensures that your web content is accessible and visually appealing on devices of all sizes.
2. The Three Pillars of Responsive Design
Responsive design relies on three fundamental principles:
- Fluid Grids: Rather than fixed pixel-based layouts, responsive design uses fluid grids that adjust and reorganize content based on the screen size. This allows the layout to adapt seamlessly to various devices.
- Flexible Images: Images in responsive design should scale proportionally to fit the screen without distorting or overflowing the layout. This is achieved by setting the max-width property to 100% on images.
- Media Queries: Media queries are CSS techniques that enable you to apply different styles based on the characteristics of the device, such as screen width, height, and orientation.
3. Leveraging jQuery for Responsive Design
jQuery, a fast and feature-rich JavaScript library, simplifies the process of creating interactive and dynamic web experiences. When it comes to responsive design, jQuery provides a range of tools and functionalities that make adapting to different devices more efficient and user-friendly.
3.1. DOM Manipulation
One of the core features of jQuery is DOM manipulation. This enables developers to alter the structure and content of a webpage dynamically. In the context of responsive design, jQuery can be used to insert, modify, or remove elements based on the screen size.
javascript $(document).ready(function() { if ($(window).width() < 768) { $('#sidebar').hide(); } });
In this code snippet, the jQuery script hides the sidebar when the screen width is less than 768 pixels. This ensures that on smaller devices, the user’s focus remains on the main content, optimizing the user experience.
3.2. Smooth Animations and Transitions
jQuery simplifies the process of adding animations and transitions to web elements. These animations can be leveraged to enhance the user experience during layout changes on different devices.
javascript $('#menu-button').click(function() { $('#menu').slideToggle('fast'); });
Here, a simple jQuery code snippet creates a smooth sliding animation for a navigation menu when the menu button is clicked. This animation maintains the user’s engagement while presenting the navigation options effectively.
3.3. Handling Touch Events
With the rise of touch-enabled devices, ensuring smooth touch interactions is crucial. jQuery offers touch event handling to make interactions like swiping, tapping, and pinching seamless and intuitive.
javascript $('#carousel').on('swipeleft', function() { // Move to the next slide });
In this example, the jQuery code detects a left swipe gesture on a carousel element and triggers an action to move to the next slide. This touch-friendly approach enhances the user experience on mobile devices.
3.4. Dynamic Content Loading
Loading unnecessary content on small screens can slow down the page and frustrate users. jQuery’s AJAX capabilities allow for dynamic content loading, fetching additional content only when needed.
javascript $('#load-more-button').click(function() { $.ajax({ url: 'load-more-content.php', success: function(response) { $('#content-container').append(response); } }); });
By utilizing AJAX with jQuery, this code snippet loads more content when the user clicks the “Load More” button, reducing initial page load times and catering to the user’s preferences.
4. Best Practices for Combining jQuery and Responsive Design
While jQuery can greatly enhance responsive design, it’s important to follow best practices to ensure a smooth and optimized experience across devices.
4.1. Progressive Enhancement
Start with a solid base layout and functionality that works without JavaScript. Then, use jQuery to enhance the user experience for devices that support it. This approach ensures that your website remains functional even if JavaScript is disabled or not supported.
4.2. Test Extensively
Thoroughly test your responsive design and jQuery enhancements on a variety of devices and screen sizes. Emulators, simulators, and real devices can help identify any issues that may arise.
4.3. Optimize Performance
Ensure that your jQuery scripts are optimized for performance. Minify your JavaScript files and use asynchronous loading techniques to prevent slowing down page load times.
4.4. Keep It Simple
Avoid overloading your website with complex jQuery animations and interactions. Focus on enhancing the user experience and usability without overwhelming the user.
4.5. Stay Updated
jQuery is constantly evolving, with updates and improvements being released. Stay up-to-date with the latest versions to take advantage of new features and bug fixes.
Conclusion
In a world where users access websites and applications on a diverse range of devices, responsive design has become a non-negotiable aspect of web development. jQuery empowers developers to enhance responsive design by providing tools for DOM manipulation, animations, touch event handling, and dynamic content loading. By following best practices and combining the power of jQuery with responsive design principles, you can create web experiences that are engaging, user-friendly, and adaptable to various devices. Embrace the synergy between jQuery and responsive design to ensure your digital creations reach and delight users across the digital landscape.
Table of Contents
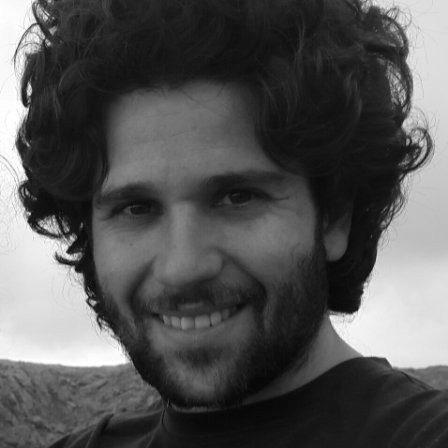
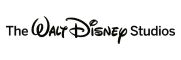