Creating Responsive Tabs with jQuery Tabs
In the ever-evolving landscape of web design, providing an optimal user experience across various devices is paramount. Responsive web design has become a standard practice, and one essential element of this is creating responsive tabs. Tabs allow you to organize and present content in a user-friendly manner, especially when dealing with a substantial amount of information.
Table of Contents
In this comprehensive guide, we’ll explore how to create responsive tabs using jQuery Tabs. jQuery Tabs is a versatile and easy-to-use JavaScript library that enables you to implement tabs quickly and efficiently on your website. We’ll cover everything from the basics of jQuery Tabs to advanced customization techniques, ensuring your tabs look great and function flawlessly on all devices.
1. Understanding the Importance of Responsive Tabs
Before we dive into the technical aspects, let’s understand why responsive tabs are crucial for cyour website.
1.1. Improved User Experience
Responsive tabs ensure that your website remains user-friendly regardless of the screen size or device being used. Visitors can easily navigate and access content, enhancing their overall experience.
1.2. Efficient Content Organization
Tabs allow you to organize content in a clean and compact manner. This is especially useful when you have a lot of information to present, such as product specifications or service details.
1.3. Reduced Page Clutter
Tabs help reduce clutter on your web pages. Instead of displaying all information at once, you can present it in a structured way, making your site visually appealing and easier to read.
Now that we understand the benefits let’s start building responsive tabs using jQuery Tabs.
2. Getting Started with jQuery Tabs
To begin, you’ll need to include the jQuery library in your project. You can either download it from the official jQuery website or include it from a Content Delivery Network (CDN) like this:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Next, download the jQuery Tabs plugin from the jQuery UI website. This plugin extends the functionality of jQuery and provides the tools we need to create tabs easily.
html <link rel="stylesheet" href="jquery-ui.min.css"> <script src="jquery-ui.min.js"></script>
Make sure to include the CSS file for styling. You can also customize the styling later to match your website’s design.
3. Creating Basic Tabs
Let’s start by creating a simple set of tabs. Suppose you have a web page with three sections: “Overview,” “Features,” and “Contact.” You want to display each of these sections within tabs.
html <div id="tabs"> <ul> <li><a href="#overview">Overview</a></li> <li><a href="#features">Features</a></li> <li><a href="#contact">Contact</a></li> </ul> <div id="overview"> <p>This is the overview section.</p> </div> <div id="features"> <p>These are the features of our product.</p> </div> <div id="contact"> <p>Contact us for more information.</p> </div> </div>
In this code snippet, we have created a div with the id “tabs” that will contain our tabbed content. Inside this div, we have an unordered list (<ul>) representing the tabs and a set of div elements for the content of each tab.
To activate the tabs, you need to initialize them in your JavaScript code. Place the following script in your HTML file:
html <script> $(function() { $("#tabs").tabs(); }); </script>
This script uses jQuery to target the “tabs” div and initializes it as a set of tabs using the tabs() function provided by the jQuery Tabs plugin.
Now, when you load your web page, you should see the tabs in action. Clicking on each tab will display the corresponding content.
4. Adding Content to Tabs
While the basic structure is in place, let’s add more content to each tab to make it informative and engaging.
html <div id="tabs"> <ul> <li><a href="#overview">Overview</a></li> <li><a href="#features">Features</a></li> <li><a href="#contact">Contact</a></li> </ul> <div id="overview"> <h2>Overview</h2> <p>Welcome to our website! We provide innovative solutions for...</p> </div> <div id="features"> <h2>Features</h2> <ul> <li>Feature 1: Lorem ipsum dolor sit amet.</li> <li>Feature 2: Consectetur adipiscing elit.</li> <li>Feature 3: Nulla ac dui et turpis consectetur.</li> </ul> </div> <div id="contact"> <h2>Contact</h2> <p>If you have any questions or need assistance, please...</p> </div> </div>
In this updated code, each tab’s content includes a heading (e.g., <h2>) and descriptive text or a list of features. This formatting makes the information more scannable and user-friendly.
Now, when you click on each tab, you’ll see the structured content within them.
5. Customizing Tab Appearance
While the default styling of jQuery Tabs is functional, you might want to customize the appearance to match your website’s design. Let’s explore some basic styling options.
5.1. Changing Tab Colors
To change the background color of the active tab, you can add the following CSS to your HTML file or a separate stylesheet:
css .ui-tabs .ui-tabs-nav li.ui-tabs-active a { background-color: #0073e6; /* Change to your desired color */ color: #fff; /* Change text color for visibility */ }
This CSS snippet targets the active tab and changes its background color. Customize the background-color property to your preferred color and adjust the color property for text visibility.
5.2. Modifying Tab Borders
You can also modify the borders of the tabs to make them visually distinct. Here’s an example:
css .ui-tabs .ui-tabs-nav li { border: 1px solid #ddd; /* Add a border to each tab */ margin-right: 5px; /* Add spacing between tabs */ }
This CSS code adds a border around each tab and provides a small margin between tabs. Adjust the border property to change the border style, color, or width according to your design requirements.
5.3. Styling Tab Content
To style the content within each tab, you can target the tab content div elements by their IDs. For instance:
css #overview { background-color: #f5f5f5; /* Set background color */ padding: 10px; /* Add padding for content */ border: 1px solid #ddd; /* Add a border around the content */ } #features { background-color: #fff; padding: 10px; border: 1px solid #ddd; } #contact { background-color: #f5f5f5; padding: 10px; border: 1px solid #ddd; }
In this example, we’ve added background colors, padding, and borders to the tab content div elements. Customize these styles to achieve the desired look for your tabs.
6. Making Tabs Responsive
Now that you have stylish tabs, let’s ensure they remain responsive on different devices. Responsive design is about adapting to various screen sizes, such as smartphones and tablets. jQuery Tabs already provide some responsiveness, but you can fine-tune it further.
6.1. Enabling Vertical Tabs for Small Screens
By default, jQuery Tabs display horizontal tabs. However, for small screens, vertical tabs are more space-efficient. To enable vertical tabs, add the following CSS:
css @media (max-width: 768px) { .ui-tabs .ui-tabs-nav { display: block; } .ui-tabs .ui-tabs-nav li { display: block; } }
This CSS code makes the tabs stack vertically when the screen width is 768 pixels or less. Adjust the max-width value to fit your design requirements.
6.2. Hiding Tabs on Very Small Screens
For screens with very limited space, you may want to hide the tabs altogether and display a button or icon to toggle them. Here’s how you can achieve this:
css @media (max-width: 480px) { .ui-tabs .ui-tabs-nav { display: none; } .ui-tabs .ui-tabs-panel { margin-top: 20px; /* Add space above content */ } .show-tabs-button { display: block; /* Display the toggle button */ text-align: center; background-color: #0073e6; color: #fff; padding: 10px; cursor: pointer; } }
In this code, tabs are hidden when the screen width is 480 pixels or less. A button with the class “show-tabs-button” is displayed, which users can click to reveal the tabs. Customize the button’s appearance to match your site’s design.
6.3. Adding JavaScript for Tab Toggle
To implement the tab toggle functionality, you’ll need some JavaScript code. Add the following script to your HTML file:
html <script> $(function() { // Hide the tabs initially on small screens $(".ui-tabs .ui-tabs-nav").hide(); // Toggle the tabs when the button is clicked $(".show-tabs-button").on("click", function() { $(".ui-tabs .ui-tabs-nav").toggle(); }); }); </script>
This script hides the tabs initially and toggles their visibility when the “show-tabs-button” is clicked.
With these responsive adjustments, your tabs will adapt gracefully to various screen sizes, providing an excellent user experience across devices.
7. Handling Tab Events
Tabs can do more than just display content. You can also perform actions when a tab is clicked or activated. Let’s explore some common tab events and how to handle them.
7.1. Tab Activation Event
You can execute code when a tab is activated by using the activate event. Here’s an example of how to change the background color of the active tab when it’s clicked:
html <script> $(function() { $("#tabs").tabs({ activate: function(event, ui) { // Reset the background color of all tabs $(".ui-tabs .ui-tabs-nav li a").css("background-color", ""); // Set the background color of the active tab $(ui.newTab).find("a").css("background-color", "#0073e6"); } }); }); </script>
In this code, we’re using the activate event to reset the background color of all tabs and then set the background color of the active tab to a different color.
7.2. Tab Load Event
You can also execute code when a tab is loaded for the first time using the load event. This is useful if you want to perform actions when a specific tab is initially displayed.
html <script> $(function() { $("#tabs").tabs({ load: function(event, ui) { if (ui.panel.id === "features") { // Code to execute when the "Features" tab is loaded console.log("Features tab loaded"); } } }); }); </script>
Here, we check if the loaded tab has the ID “features” and execute custom code accordingly.
These events allow you to add interactivity and dynamic behavior to your tabs, enhancing the user experience.
8. Advanced Customization
To truly make your tabs stand out and match your website’s unique design, you can explore advanced customization options. Here are some ideas to get you started:
8.1. Icon Tabs
Instead of plain text tabs, you can use icons to represent each tab. Combine icon fonts or SVGs with your tabs for a visually appealing navigation system.
8.2. Animated Transitions
Add smooth animations when switching between tabs. CSS transitions or JavaScript animations can provide a polished look and feel.
8.3. Tab Load Effects
Apply entrance animations or effects to the content of each tab when they are loaded. This can captivate your users and draw attention to the tab’s content.
8.4. Tab Drag-and-Drop
Implement a drag-and-drop feature to allow users to rearrange the order of tabs according to their preferences. This can be a valuable feature for applications with multiple tabs.
8.5. Tab Pagination
For cases where you have a significant number of tabs, consider adding pagination or a “More” dropdown to manage the space efficiently.
Remember, advanced customization requires a good understanding of HTML, CSS, and JavaScript, so be prepared to invest some time and effort in achieving your desired results.
Conclusion
Creating responsive tabs using jQuery Tabs is an effective way to enhance user experience, organize content efficiently, and maintain a visually appealing website across different devices. We’ve covered the basics of setting up tabs, adding content, styling, making them responsive, handling events, and even explored advanced customization options.
By implementing these techniques, you can take your website’s navigation and content presentation to the next level, ensuring that visitors have a seamless and engaging experience. Responsive tabs are a valuable tool in your web design toolkit, and with a bit of creativity, you can make them uniquely your own.
Table of Contents
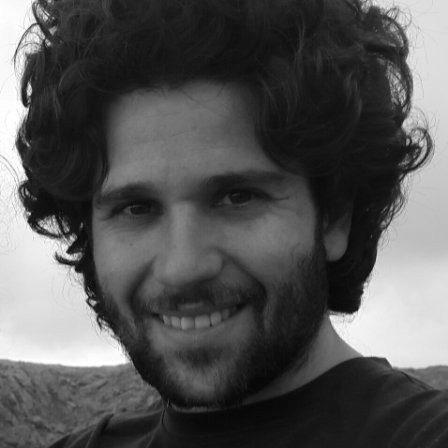
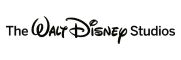