Creating Scalable Image Galleries with jQuery Masonry
In the ever-evolving landscape of web design, visual content holds a paramount place. Whether you’re a photographer, an artist, a designer, or simply want to showcase your products or memories, creating visually appealing image galleries is essential. Enter jQuery Masonry – a flexible and efficient grid layout library that empowers you to create stunning, scalable, and responsive image galleries on your website. In this article, we’ll dive into the world of jQuery Masonry and explore how to build captivating image galleries that adapt to various screen sizes and maintain a polished look.
Table of Contents
1. Why jQuery Masonry?
Traditional grid layouts often fall short when it comes to handling images of different sizes and orientations. Sticking rigidly to a fixed grid structure can result in uneven gaps and misaligned images, disrupting the overall aesthetic of your gallery. This is where jQuery Masonry comes to the rescue. It’s a popular library that arranges elements vertically, optimizing the use of space and eliminating those unsightly gaps.
2. Getting Started: Installation and Setup
Before we delve into the nitty-gritty of creating image galleries with jQuery Masonry, let’s make sure you’re set up to get started. First, you’ll need to include the jQuery library in your project. You can either download it and link it in your HTML or use a Content Delivery Network (CDN). Here’s an example of how to include jQuery using a CDN:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Next, you’ll need to download the jQuery Masonry plugin from its official website or use a CDN link as well:
html <script src="https://cdnjs.cloudflare.com/ajax/libs/masonry/4.2.2/masonry.pkgd.min.js"></script>
Now that you have both jQuery and Masonry set up, let’s move on to creating your image gallery.
3. Building the Image Gallery: HTML Markup
The structure of your image gallery is crucial to its functionality and appearance. We’ll start with a simple example using HTML and CSS. Here’s a basic HTML structure for your image gallery:
html <div class="image-gallery"> <div class="gallery-item"><img src="image1.jpg" alt="Image 1"></div> <div class="gallery-item"><img src="image2.jpg" alt="Image 2"></div> <div class="gallery-item"><img src="image3.jpg" alt="Image 3"></div> <!-- Add more gallery items as needed --> </div>
4. Styling Your Gallery: CSS
To make sure your gallery displays properly, you’ll need to apply some CSS. Here’s a simple example to get you started:
css .image-gallery { display: grid; grid-gap: 10px; /* Adjust the gap between images */ } .gallery-item { width: 100%; margin-bottom: 10px; /* Adjust the margin as needed */ } .gallery-item img { max-width: 100%; height: auto; }
5. Implementing jQuery Masonry: JavaScript
Now comes the exciting part – applying jQuery Masonry to your image gallery. Include the following JavaScript code at the end of your HTML, just before the closing </body> tag:
html <script> $(document).ready(function() { $('.image-gallery').masonry({ itemSelector: '.gallery-item', columnWidth: '.gallery-item', percentPosition: true }); }); </script>
In this code snippet, we’re using the $(document).ready() function to ensure that the JavaScript code executes after the DOM is fully loaded. The .masonry() function initializes the Masonry layout on the .image-gallery element. We provide the itemSelector as .gallery-item to specify the elements within the gallery, and columnWidth as .gallery-item to determine the width of the columns. The percentPosition option is set to true to ensure the gallery adapts proportionally to the available space.
6. Responsive Design: Adapting to Various Screen Sizes
One of the major advantages of using jQuery Masonry is its responsiveness. The grid layout automatically adjusts to different screen sizes and orientations. This means your image gallery will look great on everything from large desktop monitors to small mobile screens.
7. Adding Interactivity: Lightbox Effect
To enhance the user experience, you can add interactivity to your image gallery. A popular way to achieve this is by implementing a lightbox effect, which allows users to view larger versions of images in an overlay.
There are several lightbox libraries available, such as Fancybox or Lightbox2, that work well with jQuery Masonry. Here’s a simple example using Fancybox:
- Include the Fancybox CSS and JavaScript files in your HTML:
html <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/fancybox/3.5.7/jquery.fancybox.min.css"> <script src="https://cdnjs.cloudflare.com/ajax/libs/fancybox/3.5.7/jquery.fancybox.min.js"></script>
2. Add a class to your gallery images (e.g., lightbox) and modify the gallery structure:
html <div class="image-gallery"> <div class="gallery-item"><a class="lightbox" href="image1_large.jpg"><img src="image1.jpg" alt="Image 1"></a></div> <div class="gallery-item"><a class="lightbox" href="image2_large.jpg"><img src="image2.jpg" alt="Image 2"></a></div> <div class="gallery-item"><a class="lightbox" href="image3_large.jpg"><img src="image3.jpg" alt="Image 3"></a></div> <!-- Add more gallery items as needed --> </div>
3. Initialize Fancybox on the lightbox links:
html <script> $(document).ready(function() { $('.lightbox').fancybox(); }); </script>
8. Optimizing Performance: Lazy Loading
As your image gallery grows, loading all the images at once can impact the page’s performance. To mitigate this, you can implement lazy loading. Lazy loading delays the loading of off-screen images until the user scrolls to them.
html <script> $(document).ready(function() { $('.image-gallery').masonry({ // Masonry options... }); $('.lightbox').fancybox(); // Implement lazy loading $('img').on('load', function() { $(this).addClass('loaded'); }); $(window).on('scroll', function() { $('.gallery-item img:not(.loaded)').each(function() { if ($(this).offset().top < window.innerHeight + window.scrollY) { $(this).attr('src', $(this).attr('data-src')); } }); }); }); </script>
In this code, we’re adding a loaded class to images after they’ve loaded. We then use the scroll event to check for images within the viewport and replace their src attributes with the actual image URLs stored in the data-src attribute.
Conclusion
jQuery Masonry empowers web designers and developers to create visually captivating and dynamic image galleries that adapt seamlessly to various screen sizes and orientations. By leveraging this powerful grid layout library, you can create responsive and engaging galleries that showcase your content in the best possible light. Whether you’re building a portfolio, an online store, or a personal blog, the techniques covered in this article will help you craft stunning galleries that leave a lasting impression on your audience. So go ahead, unleash your creativity, and transform your website’s image galleries into a truly immersive visual experience.
Table of Contents
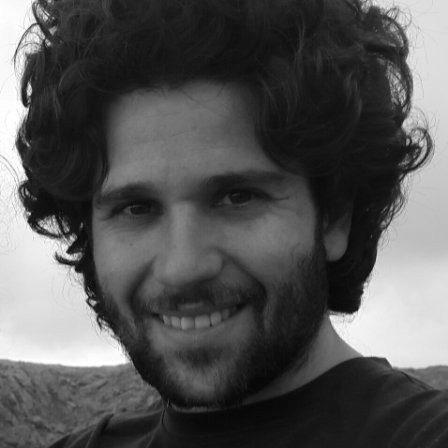
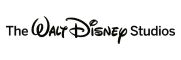