Simplifying Data Visualization with jQuery Charting Libraries
Data visualization plays a crucial role in understanding complex datasets and presenting them in an easily digestible format. As web developers, we often face the challenge of creating visually appealing charts and graphs that effectively convey information to users. To simplify this process, jQuery Charting Libraries come to the rescue. These powerful tools enable us to build dynamic and interactive charts with minimal effort. In this blog, we’ll explore the benefits of using jQuery Charting Libraries, delve into popular options, and provide step-by-step guides along with code samples to help you get started.
Table of Contents
1. Why Choose jQuery Charting Libraries?
jQuery Charting Libraries offer several advantages over traditional charting methods and other JavaScript libraries. Here’s why you should consider using them for your data visualization needs:
1.1. User-Friendly Interface:
One of the primary reasons developers opt for jQuery Charting Libraries is their user-friendly interface. With a simple and intuitive API, these libraries make it easy to create and customize charts, even for those with minimal experience in web development.
1.2. Cross-Browser Compatibility:
Ensuring your charts work seamlessly across various browsers can be challenging. jQuery Charting Libraries handle this task for you, saving time and effort on cross-browser testing. They provide consistent results on major browsers, making them a reliable choice.
1.3. Rich Set of Chart Types:
Whether you need to create pie charts, line graphs, bar charts, or more specialized visualizations like heatmaps or treemaps, jQuery Charting Libraries offer a wide range of chart types to suit your specific requirements.
1.4. Interactive Features:
Interactivity is vital for engaging user experiences. jQuery Charting Libraries come equipped with interactive features such as zooming, panning, tooltips, and hover effects, enhancing the user’s ability to explore the data.
1.5. Responsiveness:
In today’s mobile-driven world, responsiveness is key. jQuery Charting Libraries are designed to adapt to different screen sizes and resolutions, ensuring your charts look great on any device.
2. Popular jQuery Charting Libraries
Let’s take a look at some of the popular jQuery Charting Libraries that have gained widespread adoption among developers:
2.1. Chart.js:
Chart.js is an open-source library that offers a simple and elegant API for creating various chart types. It supports line charts, bar charts, pie charts, polar area charts, and more. Chart.js is beginner-friendly and requires minimal setup, making it an excellent choice for quick data visualizations.
Code Sample:
html <!DOCTYPE html> <html> <head> <title>Chart.js Example</title> <script src="https://cdn.jsdelivr.net/npm/chart.js"></script> </head> <body> <canvas id="myChart" width="400" height="200"></canvas> <script> var ctx = document.getElementById('myChart').getContext('2d'); var myChart = new Chart(ctx, { type: 'bar', data: { labels: ['January', 'February', 'March', 'April', 'May'], datasets: [{ label: 'Sales', data: [12, 19, 3, 5, 2], backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } } } }); </script> </body> </html>
2.2. Highcharts:
Highcharts is a feature-rich library known for its extensive charting capabilities. It offers a wide range of interactive charts, including line, spline, area, column, bar, and scatter plots. Highcharts provides an impressive set of customization options, making it suitable for advanced data visualization needs.
Code Sample:
html <!DOCTYPE html> <html> <head> <title>Highcharts Example</title> <script src="https://code.highcharts.com/highcharts.js"></script> </head> <body> <div id="container" style="width: 400px; height: 200px;"></div> <script> Highcharts.chart('container', { chart: { type: 'line' }, title: { text: 'Monthly Sales Data' }, xAxis: { categories: ['January', 'February', 'March', 'April', 'May'] }, yAxis: { title: { text: 'Sales' } }, series: [{ name: 'Sales', data: [12, 19, 3, 5, 2] }] }); </script> </body> </html>
2.3. FusionCharts:
FusionCharts is a comprehensive library that goes beyond traditional charting. It supports a vast array of chart types, including funnel charts, pyramid charts, and bubble charts. FusionCharts also allows integration with various platforms like React, Angular, and Vue, providing flexibility and scalability.
Code Sample:
html <!DOCTYPE html> <html> <head> <title>FusionCharts Example</title> <script src="https://cdn.fusioncharts.com/fusioncharts/latest/fusioncharts.js"></script> <script src="https://cdn.fusioncharts.com/fusioncharts/latest/themes/fusioncharts.theme.fusion.js"></script> </head> <body> <div id="chart-container" style="width: 400px; height: 200px;"></div> <script> FusionCharts.ready(function () { var fusioncharts = new FusionCharts({ type: 'pie2d', renderAt: 'chart-container', width: '100%', height: '100%', dataFormat: 'json', dataSource: { "chart": { "caption": "Monthly Sales", "theme": "fusion", "showValues": "1", "showPercentInTooltip": "0" }, "data": [ { "label": "January", "value": "12" }, { "label": "February", "value": "19" }, { "label": "March", "value": "3" }, { "label": "April", "value": "5" }, { "label": "May", "value": "2" } ] } }).render(); }); </script> </body> </html>
3. Getting Started with jQuery Charting Libraries
Now that we’ve explored some popular jQuery Charting Libraries, let’s dive into a step-by-step guide to getting started with them.
Step 1: Include the Library:
Start by including the required jQuery Charting Library in your project. You can either download the library files or include them via Content Delivery Networks (CDNs). Remember to add the library’s JavaScript and CSS files to your HTML page.
Step 2: Set Up the Data:
Prepare the data you want to visualize. Depending on the library, data can be in various formats such as arrays, JSON objects, or CSV files. Ensure the data is structured correctly to match the chosen chart type.
Step 3: Create the Container:
Create a container, such as a div element, to hold the chart. Assign a unique ID to the container for easy reference in your JavaScript code.
Step 4: Initialize the Chart:
Use JavaScript to initialize the chart by providing the chart type, data source, and any necessary configuration options.
Step 5: Render the Chart:
Finally, render the chart within the designated container by calling the appropriate method from the library’s API.
Conclusion
Data visualization is an essential aspect of modern web development, and jQuery Charting Libraries make the process of creating interactive and visually appealing charts a breeze. In this blog, we explored the benefits of using these libraries, highlighted popular options like Chart.js, Highcharts, and FusionCharts, and provided step-by-step guides along with code samples to help you get started.
Experiment with different chart types, styles, and interactive features to create stunning visualizations that effectively communicate your data’s insights. jQuery Charting Libraries are a powerful addition to any developer’s toolkit, and mastering them will undoubtedly elevate your data visualization game. Happy charting!
Remember, the best way to learn is through practice. So, roll up your sleeves, try out the code samples, and explore the vast possibilities that jQuery Charting Libraries offer. Happy coding!
Table of Contents
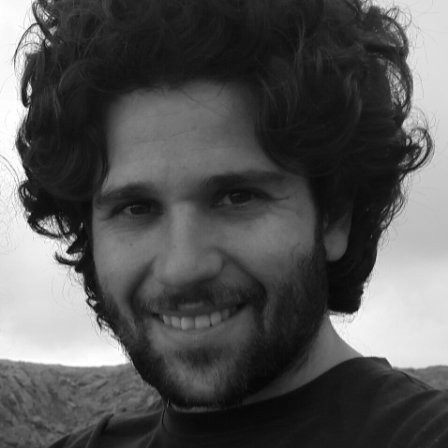
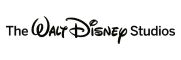