Simplifying Form Validation with jQuery Validation Plugin
In the world of web development, creating user-friendly and error-free forms is crucial for delivering a seamless user experience. A significant aspect of achieving this goal is implementing robust form validation. Enter the jQuery Validation Plugin – a powerful tool that simplifies the process of validating user input in forms. In this article, we’ll delve into the benefits of using the jQuery Validation Plugin, explore its features, and provide you with step-by-step guidance accompanied by code samples to streamline your form validation process.
Table of Contents
1. Why Use the jQuery Validation Plugin?
Form validation is the process of ensuring that user inputs are accurate and adhere to a predefined set of rules before they are submitted to the server. This not only enhances the user experience but also prevents erroneous data from reaching the server, leading to more reliable data storage and processing.
jQuery Validation Plugin offers several compelling reasons to use it for form validation:
- Ease of Use: Integrating the jQuery Validation Plugin into your web application is straightforward. With minimal effort, you can add validation rules to your form fields and customize error messages.
- Client-Side Validation: The plugin performs validation on the client side, minimizing the need for round-trips to the server for error checking. This leads to faster response times and reduces server load.
- Customization: You can define your own validation rules and error messages, tailoring them to your application’s specific requirements.
- Real-Time Feedback: As users interact with the form, the plugin provides real-time feedback on the validity of their input. This immediate feedback enhances user engagement and prevents frustration.
- Compatibility: The jQuery Validation Plugin is compatible with various browsers, ensuring a consistent experience for users regardless of their browser choice.
2. Getting Started with jQuery Validation Plugin
Let’s walk through the steps of integrating the jQuery Validation Plugin into your web application.
Step 1: Include jQuery and jQuery Validation Plugin
First, make sure you have jQuery included in your project. You can either download jQuery and host it locally or include it via a Content Delivery Network (CDN). Additionally, download the jQuery Validation Plugin from the official website or include it through a CDN.
html <!DOCTYPE html> <html> <head> <title>Form Validation with jQuery Validation Plugin</title> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="https://cdn.jsdelivr.net/jquery.validation/1.19.3/jquery.validate.min.js"></script> </head> <body> <!-- Your form goes here --> </body> </html>
Step 2: Create a Form
Next, create the HTML form that you want to validate. Assign unique IDs to each input element you want to validate. For instance:
html <form id="myForm"> <input type="text" id="username" name="username" placeholder="Username"> <input type="email" id="email" name="email" placeholder="Email"> <button type="submit">Submit</button> </form>
Step 3: Add Validation Rules
In your JavaScript code, set up the validation rules for each form field using the .validate() method provided by the jQuery Validation Plugin. Here’s an example of adding rules for the username and email fields:
javascript $(document).ready(function() { $("#myForm").validate({ rules: { username: { required: true, minlength: 3 }, email: { required: true, email: true } }, messages: { username: { required: "Please enter a username", minlength: "Username must be at least 3 characters long" }, email: { required: "Please enter an email address", email: "Please enter a valid email address" } } }); });
In this code snippet, we’ve defined validation rules for the “username” and “email” fields. The required rule ensures that the fields are not left empty, while the minlength and email rules enforce specific formatting requirements.
Step 4: Provide Error Messages
Customize the error messages that users will see when they fail to meet the validation criteria. In the previous code snippet, the messages property contains the customized error messages for each field.
3. Advanced Validation Techniques
While the basic setup covers most validation scenarios, the jQuery Validation Plugin offers more advanced techniques to handle complex requirements.
4. Conditional Validation
You can apply validation based on certain conditions. For example, you might want to validate a secondary email field only if the user provides an email in the primary field:
javascript $(document).ready(function() { $("#myForm").validate({ rules: { primaryEmail: { required: true, email: true }, secondaryEmail: { email: { depends: function(element) { return $("#primaryEmail").val() !== ""; } } } }, messages: { primaryEmail: { required: "Please enter a primary email", email: "Please enter a valid email address" }, secondaryEmail: { email: "Please enter a valid email address for the secondary email" } } }); });
In this example, the “secondaryEmail” field is validated only if the “primaryEmail” field has a value.
5. Remote Validation
Sometimes, you might need to validate input against a remote data source, like checking if a username is already taken. The jQuery Validation Plugin supports remote validation:
javascript $(document).ready(function() { $("#myForm").validate({ rules: { username: { required: true, remote: { url: "check-username-availability.php", type: "post" } } }, messages: { username: { required: "Please enter a username", remote: "This username is already taken" } } }); });
In this example, the plugin sends a request to the server to check if the chosen username is available.
Conclusion
The jQuery Validation Plugin significantly simplifies the process of form validation by providing an intuitive way to enforce rules on user inputs. Its ease of use, real-time feedback, and customization options make it a valuable tool for enhancing user experiences. By following the steps outlined in this article and exploring advanced validation techniques, you can seamlessly integrate this plugin into your web applications and ensure error-free form submissions. Remember, a well-validated form is not only a testament to your development skills but also a key component of a successful user-centric web application.
Incorporating the jQuery Validation Plugin into your projects is a worthwhile endeavor. Its straightforward implementation, real-time feedback, and customization capabilities will elevate your form validation process. Whether you’re building a simple contact form or a complex registration system, this plugin empowers you to create a seamless user experience while ensuring data accuracy. So, dive into the world of jQuery Validation and unlock the potential of efficient and effective form validation.
Remember, an optimized user experience starts with accurate data input, and the jQuery Validation Plugin is your partner in achieving that goal. Happy coding!
Table of Contents
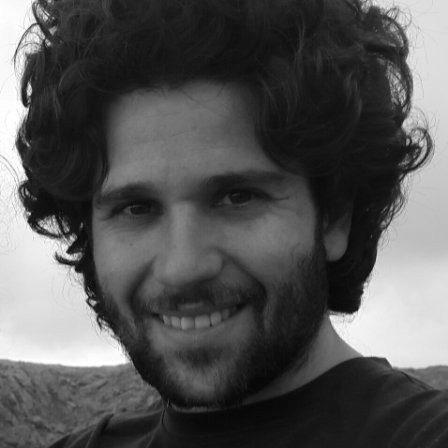
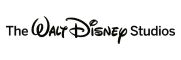