Simplifying JavaScript with jQuery: A Beginner’s Guide
JavaScript is a powerful programming language that enables dynamic and interactive web development. However, working with raw JavaScript can sometimes be complex and time-consuming, especially for beginners. That’s where jQuery comes in. jQuery is a fast, small, and feature-rich JavaScript library that simplifies the process of writing JavaScript code, making it more efficient and accessible. In this beginner’s guide, we will explore the benefits of using jQuery and provide practical examples to help you get started.
1. Why jQuery?
1.1 Simplified DOM Manipulation
One of the key advantages of jQuery is its simplified approach to DOM (Document Object Model) manipulation. The DOM is a programming interface that represents the structure of an HTML document, allowing JavaScript to interact with and modify the elements on a web page. In JavaScript, DOM manipulation can be verbose and repetitive. jQuery provides a concise syntax and a wide range of methods to easily select and manipulate HTML elements.
For example, to change the text of an element with the ID “myElement” using JavaScript, you would typically write:
javascript document.getElementById("myElement").innerHTML = "New text";
With jQuery, the same task can be accomplished with just one line of code:
javascript $("#myElement").text("New text");
1.2 Cross-browser Compatibility
Another major advantage of jQuery is its excellent cross-browser compatibility. JavaScript code often needs to work seamlessly across different web browsers, each with its own quirks and inconsistencies. jQuery takes care of these browser-specific differences behind the scenes, providing a consistent and reliable development experience. This allows developers to focus on building functionality without worrying about browser compatibility issues.
1.3 Extensive Feature Set
jQuery offers a wide range of features and utilities that can significantly simplify common JavaScript tasks. It provides built-in methods for animations, event handling, AJAX requests, and much more. Whether you need to create a smooth fade-in effect or dynamically load content from a server, jQuery has you covered. By leveraging these pre-built functionalities, developers can save time and effort, resulting in faster development cycles and cleaner code.
2. Getting Started with jQuery
2.1 Including jQuery in Your Project
To start using jQuery, you need to include the library in your HTML file. There are several ways to do this. You can download the jQuery file and host it on your own server, or you can use a content delivery network (CDN) to reference a hosted version of jQuery. The latter option is recommended for most scenarios, as it ensures that you are using the latest stable version and takes advantage of caching benefits. Here’s an example of including jQuery using a CDN:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
By including this script tag in your HTML file, you gain access to all the features and methods provided by jQuery.
2.2 Selecting Elements
One of the fundamental concepts in jQuery is selecting elements from the DOM. jQuery uses CSS-like selectors to target specific elements or groups of elements. The selected elements can then be manipulated or interacted with using jQuery methods.
For example, to select all paragraphs on a page and change their font color to red, you can use the following code:
javascript $("p").css("color", "red");
In this code snippet, $(“p”) selects all <p> elements, and the css() method is used to modify their CSS properties.
2.3 Manipulating Elements
Once you have selected an element or a group of elements, jQuery provides a variety of methods to manipulate them. You can modify their content, attributes, styles, and more.
For instance, to add a CSS class to a specific element, you can use the addClass() method:
javascript $("#myElement").addClass("highlight");
In this example, $(“#myElement”) selects the element with the ID “myElement,” and the addClass() method adds the “highlight” class to it.
3. Practical Examples
3.1 Smooth Animations
jQuery’s animation capabilities make it easy to add smooth and visually appealing effects to your web pages. You can animate properties such as width, height, opacity, and more. Here’s an example of animating the height of a <div> element:
javascript $("#myDiv").animate({ height: "200px" }, 1000);
This code animates the height of the element with the ID “myDiv” to 200 pixels over a duration of 1000 milliseconds.
3.2 AJAX Requests
jQuery simplifies the process of making AJAX requests, allowing you to fetch data from servers without reloading the entire page. The $.ajax() method provides a flexible and easy-to-use interface for performing AJAX operations. Here’s an example of making a GET request and handling the response:
javascript $.ajax({ url: "https://api.example.com/data", method: "GET", success: function(response) { // Handle the response data console.log(response); }, error: function(error) { // Handle errors console.error(error); } });
In this code, the url property specifies the endpoint to which the request is sent, the method property defines the HTTP method (GET in this case), and the success and error properties specify the callbacks to handle the response or any errors.
Conclusion
jQuery is a powerful tool that simplifies JavaScript development, particularly for beginners. Its concise syntax, cross-browser compatibility, and extensive feature set make it a popular choice for many web developers. By leveraging jQuery, you can streamline your code, enhance user experience, and save valuable development time. Whether you’re working on a small project or a large-scale application, jQuery can help simplify your JavaScript workflow and bring your ideas to life. So, why not give it a try and explore its possibilities in your next web project?
In conclusion, jQuery provides a beginner-friendly approach to JavaScript development, making it accessible to developers of all skill levels. Its simplified DOM manipulation, cross-browser compatibility, and extensive feature set make it a valuable tool in any web developer’s toolkit. By utilizing jQuery, you can streamline your JavaScript code, enhance your web projects, and bring your ideas to life more efficiently. So don’t hesitate to dive into the world of jQuery and experience its benefits firsthand. Happy coding!
Table of Contents
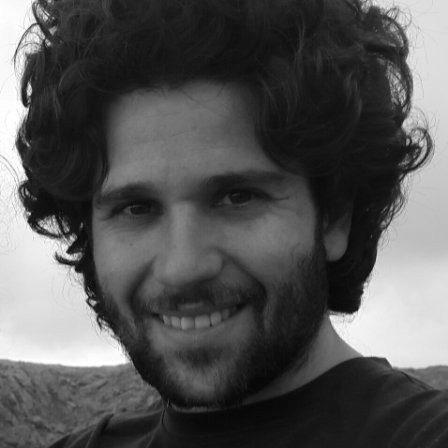
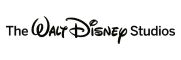