Exploring the Power of jQuery: Essential Techniques for Interactive Websites
In the world of web development, creating interactive and dynamic websites is key to engaging users and enhancing their experience. With the power of jQuery, a fast and concise JavaScript library, developers can achieve impressive results with ease. Whether you’re a seasoned developer or just starting out, understanding jQuery and its essential techniques is essential to take your web projects to the next level. In this comprehensive guide, we will explore the power of jQuery and dive into its fundamental techniques, from selectors to animations, that will enable you to create highly interactive websites.
1. Understanding jQuery: An Overview
jQuery is a JavaScript library designed to simplify the process of web development by providing a concise and powerful set of tools. With jQuery, developers can manipulate HTML documents, handle events, create animations, and much more, with just a few lines of code. It abstracts complex JavaScript operations into simple functions, making it accessible to developers of all skill levels.
2. Getting Started: Setting Up jQuery
To get started with jQuery, you need to include the jQuery library in your web project. You can either download the library and host it locally or link to a hosted version via a Content Delivery Network (CDN). Here’s an example of including jQuery using a CDN:
html <head> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> </head>
3. Selecting Elements: The Power of Selectors
One of the most powerful features of jQuery is its ability to select elements from the DOM (Document Object Model) using CSS-like selectors. This allows you to easily manipulate and interact with specific elements on your web page. Here are some commonly used selectors:
3.1 ID Selector:
javascript $('#myElement') // Selects an element with the ID "myElement"
3.2 Class Selector:
javascript $('.myClass') // Selects all elements with the class "myClass"
3.3 Element Selector:
avascript $('p') // Selects all <p> elements
4. Manipulating the DOM: Changing Content and Styles
Once you’ve selected an element, jQuery provides powerful methods to manipulate the content and style of those elements. Here are a few essential techniques:
4.1 Changing Text Content:
javascript $('#myElement').text('New text content') // Changes the text content of an element
4.2 Modifying HTML Content:
javascript $('#myElement').html('<p>New HTML content</p>') // Changes the HTML content of an element
4.3 Modifying CSS Styles:
javascript $('#myElement').css('color', 'red') // Changes the color style of an element to red
5. Handling Events: Interactivity at Your Fingertips
jQuery simplifies event handling by providing a unified API to bind event listeners and execute code when events occur. Here’s an example of handling a click event:
javascript $('#myButton').on('click', function() { alert('Button clicked!'); });
6. Animations: Bringing Your Website to Life
jQuery’s animation capabilities allow you to create dynamic and engaging effects on your web pages. Whether it’s fading elements in and out, sliding content, or creating custom animations, jQuery makes it easy. Here’s an example of a simple fade animation:
javascript $('#myElement').fadeIn(1000); // Fades in an element over 1 second
7. AJAX: Seamless Data Exchange
Asynchronous JavaScript and XML (AJAX) enables you to exchange data with a server without reloading the entire page. jQuery provides a convenient set of methods for making AJAX requests and handling responses. Here’s a basic example of making an AJAX request:
javascript $.ajax({ url: 'https://api.example.com/data', method: 'GET', success: function(response) { console.log(response); }, error: function(error) { console.error(error); } });
8. Plugins and Extensibility: Expanding jQuery’s Power
jQuery’s extensibility is one of its major strengths. There is a vast ecosystem of plugins available that provide additional functionality and make complex tasks even easier. Whether you need a carousel, a date picker, or a rich text editor, chances are there’s a jQuery plugin available to meet your needs.
Conclusion:
jQuery is a powerful and versatile JavaScript library that empowers developers to create highly interactive and engaging websites. By mastering the essential techniques covered in this guide, you’ll be equipped to build dynamic web pages, handle events, create stunning animations, and seamlessly exchange data with servers. With its simplicity and wide-ranging capabilities, jQuery remains a popular choice for developers looking to enhance the interactivity of their web projects. So go ahead, dive into the world of jQuery, and unlock the full potential of interactive web development.
Table of Contents
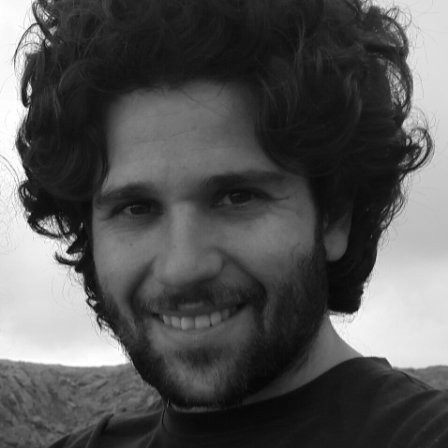
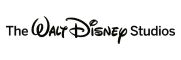