jQuery Templating: Building Reusable UI Components
In the ever-evolving landscape of web development, creating dynamic and interactive user interfaces is of paramount importance. One of the key challenges developers face is efficiently building and maintaining UI components that can be reused across various parts of a website or application. This is where jQuery templating comes into play. In this guide, we’ll delve into the world of jQuery templating, exploring its benefits, techniques, and providing insightful code samples.
Table of Contents
1. Understanding the Need for Reusable UI Components
As web applications grow in complexity, maintaining a consistent and user-friendly experience becomes crucial. Imagine having to recreate similar UI elements like navigation bars, product listings, or comments sections across multiple pages. This not only wastes development time but also increases the chances of inconsistencies and errors. Reusable UI components solve this problem by allowing developers to create modular pieces of UI that can be easily integrated into various parts of the application.
2. The Power of jQuery Templating
jQuery templating is a technique that leverages the power of jQuery, a popular JavaScript library, to generate dynamic HTML content based on data. It provides a structured approach to creating reusable UI components by separating the HTML structure from the data that populates it. This separation promotes a cleaner and more maintainable codebase, making it easier to update UI elements without affecting the underlying functionality.
3. Benefits of jQuery Templating
- Code Reusability: With templating, you can create UI components once and reuse them across multiple parts of your application, reducing code duplication and enhancing consistency.
- Data-Driven: Templating allows you to dynamically generate UI components based on data, making it ideal for scenarios where content needs to be updated frequently.
- Maintenance: Separating the UI structure from the data simplifies maintenance. You can update the template without altering the JavaScript logic, and vice versa.
- Performance: jQuery templating often leads to better performance compared to manually manipulating the DOM, as the library handles rendering efficiently.
4. Common jQuery Templating Libraries
Several jQuery templating libraries have emerged over the years, each offering its own set of features and syntax. Let’s take a look at a few popular ones:
4.1. jQuery.tmpl
jQuery.tmpl is a simple and lightweight templating engine that follows a concise syntax. Here’s a basic example of how it works:
javascript <script id="template" type="text/x-jquery-tmpl"> <div class="user"> <h2>${name}</h2> <p>${email}</p> </div> </script> <div id="users-container"></div> <script> var users = [ { name: "Alice", email: "alice@example.com" }, { name: "Bob", email: "bob@example.com" } ]; var template = $("#template").tmpl(users); $("#users-container").append(template); </script>
In this example, the #template script tag contains the HTML structure of the user component, and the ${} placeholders are used to insert data from the users array.
4.2. Handlebars.js
Handlebars.js is a popular templating library that extends the basic concept of templating with more advanced features like helpers and partials. Here’s a snippet illustrating its usage:
javascript <script id="template" type="text/x-handlebars-template"> <div class="product"> <h2>{{name}}</h2> <p>{{description}}</p> <span>${{price}}</span> </div> </script> <div id="products-container"></div> <script> var products = [ { name: "Product 1", description: "Description 1", price: 29.99 }, { name: "Product 2", description: "Description 2", price: 39.99 } ]; var template = Handlebars.compile($("#template").html()); $("#products-container").html(template(products)); </script>
Handlebars.js uses double curly braces {{}} to denote placeholders. It compiles the template using Handlebars.compile() and then inserts data into the template.
5. Best Practices for jQuery Templating
While jQuery templating can greatly improve development efficiency, there are some best practices to keep in mind:
- Separation of Concerns: Maintain a clear separation between HTML templates and JavaScript logic. This makes your codebase more organized and easier to understand.
- Use Helpers: If your chosen templating library supports helpers or filters, utilize them to perform complex operations within the template itself. This can help reduce the complexity of your JavaScript code.
- Avoid Inline Scripts: Instead of embedding JavaScript directly within your template, keep your JavaScript code in separate script files. This enhances code readability and maintainability.
- Caching Templates: If you’re using a template multiple times, cache it for better performance. This prevents unnecessary recompilation of the same template.
Conclusion
jQuery templating is a powerful tool for building reusable UI components that enhance the efficiency and maintainability of your web applications. By separating UI structure from data, these templates enable developers to create modular pieces of code that can be easily integrated across different parts of the application. Whether you choose a lightweight library like jQuery.tmpl or a feature-rich one like Handlebars.js, mastering the art of templating will undoubtedly elevate your web development skills. So, embrace the power of jQuery templating and unlock a world of dynamic and reusable UI components. Your users and fellow developers will thank you for it.
Table of Contents
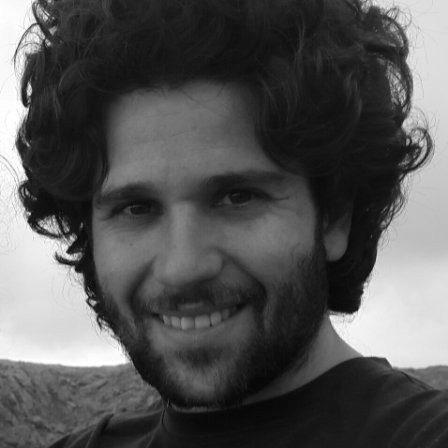
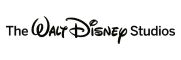