Creating Stunning UI Effects with jQuery
In today’s competitive digital landscape, it’s crucial for websites to captivate and engage users right from the moment they land on a page. User interface (UI) effects play a significant role in achieving this goal, as they can add visual interest, interactivity, and enhance the overall user experience. jQuery, a popular JavaScript library, provides developers with a powerful toolkit to create stunning UI effects with ease. In this comprehensive guide, we will explore various techniques, step-by-step instructions, and code samples to help you master the art of creating jaw-dropping UI effects with jQuery.
Getting Started with jQuery:
Before we dive into creating UI effects, let’s quickly review the basics of jQuery. If you’re new to jQuery, it is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal, event handling, and animation. To get started, you need to include the jQuery library in your HTML file. You can either download the library or include it via a Content Delivery Network (CDN). Here’s an example:
html <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
Animating Elements:
Animating elements is one of the core functionalities of jQuery. You can create eye-catching transitions, fades, slides, and more. Let’s start with a simple example that animates a div element when a button is clicked:
html <!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <style> #animated-div { width: 200px; height: 200px; background-color: red; } </style> </head> <body> <div id="animated-div"></div> <button id="animate-button">Animate</button> <script> $(document).ready(function() { $("#animate-button").click(function() { $("#animated-div").animate({ width: 'toggle', height: 'toggle' }); }); }); </script> </body> </html>
In this code sample, we create a div element with an initial width and height of 200 pixels and a red background. When the “Animate” button is clicked, the animate() function is triggered, animating the width and height of the div element.
Creating Sliders and Carousels:
Sliders and carousels are commonly used UI elements to showcase images, testimonials, or any other content in an interactive and visually appealing manner. jQuery provides plugins such as “slick” and “owl.carousel” that simplify the creation of sliders and carousels. Here’s an example using the “slick” plugin:
html <!DOCTYPE html> <html> <head> <link rel="stylesheet" type="text/css" href="https://cdn.jsdelivr.net/npm/slick-carousel@1.8.1/slick/slick.css"/> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/slick-carousel@1.8.1/slick/slick.min.js"></script> <style> .slider { width: 500px; margin: 0 auto; } .slider img { width: 100%; } </style> </head> <body> <div class="slider"> <img src="image1.jpg" alt="Image 1"> <img src="image2.jpg" alt="Image 2"> <img src="image3.jpg" alt="Image 3"> </div> <script> $(document).ready(function() { $('.slider').slick(); }); </script> </body> </html>
In this example, we include the necessary CSS and JavaScript files for the “slick” plugin. We create a div element with the class “slider” and place the images inside it. Finally, we call the slick() function on the “slider” class to initialize the slider.
Adding Parallax Effects:
Parallax effects provide a sense of depth and immersion to your website by creating an illusion of different layers moving at different speeds. jQuery makes it straightforward to implement parallax effects. Here’s an example:
html <!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <style> .parallax-section { background-image: url(background.jpg); background-size: cover; background-position: center; height: 600px; position: relative; } .parallax-content { position: absolute; top: 50%; left: 50%; transform: translate(-50%, -50%); color: white; font-size: 24px; } </style> </head> <body> <div class="parallax-section"> <div class="parallax-content"> <h1>Welcome to Our Website</h1> <p>Discover an immersive experience.</p> </div> </div> <script> $(window).scroll(function() { var scrollPosition = $(this).scrollTop(); $(".parallax-section").css("background-position", "center " + -(scrollPosition / 4) + "px"); }); </script> </body> </html>
In this code snippet, we define a section with the class “parallax-section” that has a background image. Inside this section, we have a content container with the class “parallax-content.” As the user scrolls, we adjust the background-position property of the section using the scrollTop() function to create the parallax effect.
Enhancing User Interactions:
jQuery allows you to add various interactive elements to your website, such as tooltips, modals, and dropdown menus. Let’s take a look at creating a simple dropdown menu using jQuery:
html <!DOCTYPE html> <html> <head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <style> .dropdown { position: relative; display: inline-block; } .dropdown-content { display: none; position: absolute; background-color: #f9f9f9; min-width: 160px; box-shadow: 0px 8px 16px 0px rgba(0,0,0,0.2); z-index: 1; } .dropdown:hover .dropdown-content { display: block; } </style> </head> <body> <div class="dropdown"> <button>Menu</button> <div class="dropdown-content"> <a href="#">Option 1</a> <a href="#">Option 2</a> <a href="#">Option 3</a> </div> </div> </body> </html>
In this example, we create a dropdown menu using a combination of CSS and jQuery. The dropdown content is initially hidden, and when the user hovers over the dropdown, the display property is set to “block,” revealing the menu options.
Conclusion:
In this comprehensive guide, we explored various techniques to create stunning UI effects with jQuery. From animating elements to building sliders, implementing parallax effects, and enhancing user interactions, jQuery offers a wide range of capabilities to take your website’s visuals to the next level. Remember to experiment, combine different effects, and customize them to suit your specific design requirements. With jQuery as your ally, you can create an engaging and visually appealing user interface that leaves a lasting impression on your website visitors. Happy coding!
Table of Contents
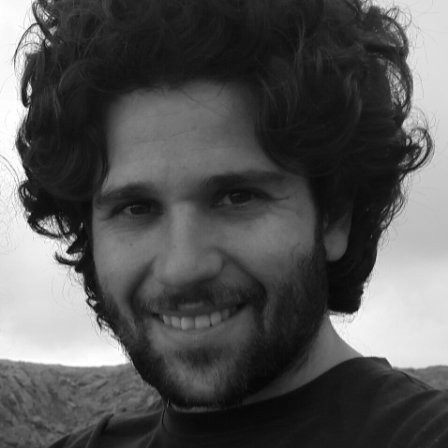
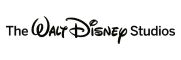