Creating Sliding Panels with jQuery UI Slider
In the world of web development, interactive and dynamic user interfaces are key to engaging user experiences. Sliding panels are a popular choice for displaying content in a visually appealing and space-efficient manner. jQuery UI Slider is a powerful tool that can help you implement sliding panels seamlessly into your web applications. In this guide, we’ll walk you through the process of creating sliding panels with jQuery UI Slider, providing step-by-step instructions, code samples, and helpful tips along the way.
Table of Contents
1. Getting Started with jQuery UI Slider
1.1. What is jQuery UI Slider?
jQuery UI is a popular JavaScript library that extends the capabilities of jQuery, making it easier to build interactive web interfaces. The jQuery UI Slider widget is a part of this library and allows you to create customizable slider controls that can be used for various purposes, including creating sliding panels.
1.2. Prerequisites
Before diving into the implementation, make sure you have the following prerequisites in place:
- Basic knowledge of HTML, CSS, and JavaScript.
- A code editor (e.g., Visual Studio Code, Sublime Text, or any of your preference).
- jQuery and jQuery UI libraries included in your project.
2. Setting Up Your HTML Structure
Start by creating the basic HTML structure for your sliding panel. Here’s a simple example:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Sliding Panel Example</title> <link rel="stylesheet" href="jquery-ui.min.css"> </head> <body> <div class="sliding-panel"> <div class="content"> <!-- Content for the sliding panel goes here --> </div> </div> <script src="jquery.min.js"></script> <script src="jquery-ui.min.js"></script> <script src="script.js"></script> </body> </html>
In this structure, we have a container div with the class “sliding-panel” that will hold the content of our sliding panel.
3. Styling Your Sliding Panel
Before adding the jQuery UI Slider functionality, let’s style the sliding panel to make it visually appealing. You can use CSS to achieve the desired look. Here’s a basic example:
css /* Add this to your CSS file */ .sliding-panel { position: fixed; top: 0; right: -300px; /* Start with the panel outside the viewport */ width: 300px; height: 100%; background-color: #f0f0f0; box-shadow: -2px 0 5px rgba(0, 0, 0, 0.2); transition: right 0.3s ease-in-out; } .content { padding: 20px; }
In this CSS snippet, we position the sliding panel on the right side of the viewport and use the “transition” property for smooth sliding animations.
4. Implementing jQuery UI Slider
Now, it’s time to add the jQuery UI Slider to our sliding panel. Create a JavaScript file (e.g., “script.js”) and include the following code:
javascript $(document).ready(function () { // Select the sliding panel and the content div var slidingPanel = $(".sliding-panel"); var content = $(".content"); // Initialize the jQuery UI Slider slidingPanel.slider({ range: "max", // Use a single handle min: 0, // Minimum value max: 300, // Maximum value (adjust as needed) value: 0, // Initial value (panel closed) slide: function (event, ui) { // Adjust the right position of the panel and opacity of the content slidingPanel.css("right", -ui.value); content.css("opacity", 1 - ui.value / 300); }, }); // Function to toggle the panel open/close function togglePanel() { var currentValue = slidingPanel.slider("value"); // If the panel is closed, open it; otherwise, close it slidingPanel.slider("value", currentValue === 0 ? 300 : 0); } // Add a click event listener to a trigger element (e.g., a button) $("#toggle-button").on("click", togglePanel); });
In this JavaScript code, we:
- Select the sliding panel and the content div using jQuery.
- Initialize the jQuery UI Slider on the sliding panel.
- Define a “slide” function to control the position of the sliding panel and the opacity of the content as the slider moves.
- Create a “togglePanel” function to open and close the panel by changing the slider’s value.
- Add a click event listener to a trigger element (with the id “toggle-button” in this example) to call the “togglePanel” function.
5. Adding a Trigger Button
To allow users to open and close the sliding panel, you can add a trigger button to your HTML file. Here’s an example:
html <button id="toggle-button">Toggle Panel</button>
This button, when clicked, will toggle the sliding panel open and closed.
6. Customizing Your Sliding Panel
6.1. Adjusting Panel Width and Animation Speed
You can customize the width of your sliding panel and the animation speed by modifying the jQuery UI Slider options. In the JavaScript code, you can adjust the “max” value to change the panel’s width and the animation speed in the “slide” function by modifying the “transition” property duration in the CSS.
6.2. Enhancing the Content
To make your sliding panel more engaging, consider enhancing the content within it. You can add images, forms, videos, or any other web elements to create an informative and interactive user experience.
html <div class="sliding-panel"> <div class="content"> <h2>Welcome to our Sliding Panel</h2> <p>Here, you can find information, images, and more.</p> <img src="image.jpg" alt="Sample Image"> <form> <!-- Your form fields go here --> </form> </div> </div>
6.3. Styling and Theming
jQuery UI Slider allows you to customize its appearance and theme to match your website’s design. You can explore different themes and apply CSS styles to change the slider’s look and feel. jQuery UI provides a theming API and a wide range of themes to choose from.
Conclusion
In this tutorial, we’ve explored how to create sliding panels with jQuery UI Slider. We began by setting up the HTML structure, styling the panel, and then implementing the slider functionality using JavaScript. With this knowledge, you can add interactive sliding panels to your web applications, providing users with a dynamic and engaging experience.
Remember that the possibilities are limitless when it comes to customizing your sliding panel. You can further enhance it by adding animations, transitions, and more complex content. Feel free to experiment and tailor the sliding panel to suit your specific project requirements.
By mastering the art of creating sliding panels with jQuery UI Slider, you’re well on your way to building more user-friendly and interactive web applications. Happy coding!
Table of Contents
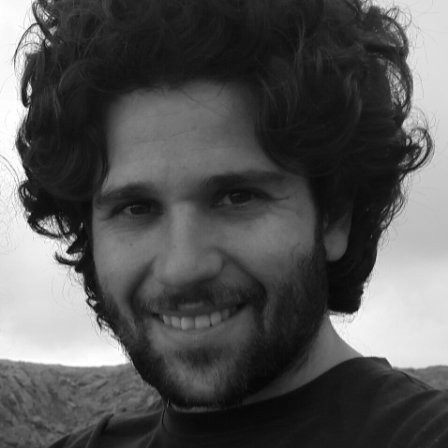
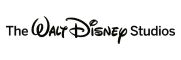