Harnessing the Power of jQuery UI Widgets
In the ever-evolving world of web development, creating dynamic and user-friendly interfaces is crucial to keeping users engaged. jQuery UI Widgets, a powerful library built on top of jQuery, provides a vast array of interactive elements to enhance the functionality and aesthetics of web applications. From sliders and accordions to date pickers and progress bars, jQuery UI widgets simplify complex UI components, making them easily accessible to developers of all levels.
Table of Contents
In this blog post, we’ll explore the benefits of jQuery UI widgets and delve into the implementation of some popular widgets through code samples. By the end, you’ll have a deeper understanding of how to harness the power of jQuery UI widgets to create engaging and feature-rich web applications.
1. The Advantages of jQuery UI Widgets:
Before we dive into the technical aspects, let’s explore the advantages of using jQuery UI widgets in your projects.
1.1. Cross-Browser Compatibility:
One of the most significant benefits of using jQuery UI widgets is their inherent cross-browser compatibility. The library has been thoroughly tested across major browsers, ensuring a consistent user experience across different platforms. This eliminates the need for time-consuming browser-specific hacks, saving developers precious time and effort.
1.2. Customizability:
jQuery UI widgets come with a plethora of customization options, allowing developers to tweak the appearance and behavior of the widgets to suit their project’s specific requirements. From setting color schemes to modifying animation effects, the customization possibilities are vast, ensuring that your UI matches your application’s overall design language.
1.3. Enhanced User Experience:
By incorporating jQuery UI widgets, you can significantly enhance the user experience of your web applications. The interactive nature of widgets, such as drag-and-drop functionality and smooth animations, makes the application feel more intuitive and responsive to user actions. This ultimately leads to higher user satisfaction and retention.
1.4. Lightweight and Efficient:
Despite their rich features, jQuery UI widgets maintain a lightweight footprint, optimizing page load times and overall performance. This is crucial for ensuring that your web application remains fast and responsive, especially for users with slower internet connections or less powerful devices.
2. Getting Started with jQuery UI:
To begin harnessing the power of jQuery UI widgets, you’ll need to include the jQuery and jQuery UI libraries in your project. You can either download the files and host them locally or include them via a CDN (Content Delivery Network) for improved loading times:
html <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include jQuery UI --> <script src="https://code.jquery.com/ui/1.13.0/jquery-ui.min.js"></script> <!-- Include the jQuery UI CSS --> <link rel="stylesheet" href="https://code.jquery.com/ui/1.13.0/themes/base/jquery-ui.css">
3. Exploring Popular jQuery UI Widgets:
Now, let’s take a closer look at some of the most commonly used jQuery UI widgets and how to implement them in your web applications.
3.1. Accordion Widget:
The Accordion widget allows you to stack multiple panels vertically, showing only one panel at a time. This is ideal for creating collapsible menus, FAQs, or any content that can be grouped into sections.
html <div id="accordion"> <h3>Section 1</h3> <div> <p>Content for section 1 goes here.</p> </div> <h3>Section 2</h3> <div> <p>Content for section 2 goes here.</p> </div> <h3>Section 3</h3> <div> <p>Content for section 3 goes here.</p> </div> </div> <script> // Initialize the Accordion widget $("#accordion").accordion(); </script>
3.2. Datepicker Widget:
The Datepicker widget simplifies the process of selecting dates from a calendar. It provides an interactive calendar interface and various date format options.
html <input type="text" id="datepicker"> <script> // Initialize the Datepicker widget $("#datepicker").datepicker(); </script>
3.3. Progressbar Widget:
The Progressbar widget visualizes the progress of a task or process, providing real-time feedback to users.
html <div id="progressbar"></div> <script> // Initialize the Progressbar widget $("#progressbar").progressbar({ value: 50 // Set the initial progress value (0 to 100) }); </script>
4. Implementing Interactivity with Widgets:
Apart from using widgets as standalone components, you can also combine them to create more complex and interactive features.
4.1. Draggable and Droppable Widgets:
The Draggable and Droppable widgets allow elements to be dragged and dropped, making it easy to implement features like sortable lists or drag-and-drop file uploads.
html <div id="draggable">Drag me</div> <div id="droppable">Drop here</div> <script> // Initialize the Draggable and Droppable widgets $("#draggable").draggable(); $("#droppable").droppable({ drop: function(event, ui) { // Code to handle the drop event } }); </script>
4.2. Resizable Widget:
The Resizable widget enables users to resize elements, such as text areas or images, by dragging the resize handle.
html <div id="resizable">Resize me</div> <script> // Initialize the Resizable widget $("#resizable").resizable(); </script>
Conclusion
jQuery UI widgets offer a powerful and flexible way to enhance your web applications with interactive and user-friendly components. Their cross-browser compatibility, customizability, and lightweight nature make them an excellent choice for developers looking to create intuitive and engaging user interfaces.
In this blog post, we explored the benefits of jQuery UI widgets, how to get started with the library, and implementation examples of some popular widgets. By leveraging the potential of jQuery UI widgets, you can take your web applications to new heights, impressing users and improving their overall experience.
So, why wait? Start harnessing the power of jQuery UI widgets today and elevate your web development projects to the next level!
Table of Contents
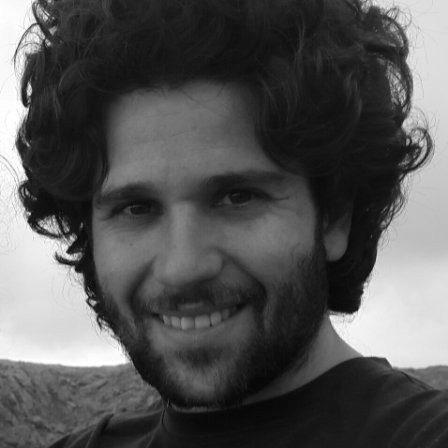
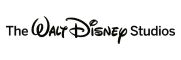