Understanding jQuery Selectors: A Comprehensive Overview
jQuery is a powerful JavaScript library that simplifies the process of DOM manipulation and event handling. One of the key features that makes jQuery so popular and widely used is its ability to select and manipulate HTML elements with ease using selectors. Selectors in jQuery are a set of powerful tools that allow you to target specific elements in your web page and perform various operations on them. In this blog, we will take a deep dive into jQuery selectors, understand their syntax, and explore various types of selectors with code examples.
What are jQuery Selectors?
At its core, a jQuery selector is a function that allows you to find and select DOM elements based on their attributes, properties, classes, or relationships with other elements. Just like CSS selectors, jQuery selectors enable you to access and interact with HTML elements in a structured and efficient way.
jQuery selectors follow a similar syntax to CSS selectors, making it easy for developers familiar with CSS to quickly get started with jQuery. The primary method used for selecting elements is jQuery() or the shorthand $().
js // Using jQuery() to select an element by its ID const elementById = $("#elementId"); // Using jQuery() to select all elements with a specific class const elementsByClass = $(".elementClass"); // Using jQuery() to select all <p> elements const allParagraphs = $("p");
In the above code samples, we’ve used different jQuery selectors to select elements by their ID, class, and tag name. The result of each selection is a jQuery object containing one or more matched elements.
Different Types of jQuery Selectors
jQuery provides a wide range of selectors to accommodate various use cases. Let’s explore some of the most commonly used jQuery selectors:
1. Element Selector
The element selector selects all elements that match the specified tag name. It is denoted by the element name within the parentheses.
js // Select all <p> elements const allParagraphs = $("p");
2. ID Selector
The ID selector selects a single element with a specific ID attribute. It is denoted by a hash symbol (#) followed by the ID value.
html <!-- HTML --> <div id="myDiv">This is a div with an ID</div> js // Select the element with ID "myDiv" const myDivElement = $("#myDiv");
3. Class Selector
The class selector selects all elements that have a specific class. It is denoted by a dot (.) followed by the class name.
html <!-- HTML --> <p class="highlight">This paragraph has a class</p> js // Select all elements with class "highlight" const highlightedElements = $(".highlight");
4. Attribute Selector
The attribute selector selects elements based on their attribute values. It is denoted by square brackets ([]) containing the attribute name and an optional attribute value.
html <!-- HTML --> <input type="text" data-custom="inputField"> js // Select the input element with the attribute "data-custom" set to "inputField" const inputField = $("input[data-custom='inputField']");
5. Descendant Selector
The descendant selector selects elements that are descendants of a specified parent element. It is denoted by a space between the parent and child elements.
html <!-- HTML --> <div class="parent"> <p>This is a paragraph inside a parent div</p> </div> js // Select all <p> elements inside elements with class "parent" const paragraphsInsideParent = $(".parent p");
6. Child Selector
The child selector selects elements that are direct children of a specified parent element. It is denoted by a greater than sign (>).
html <!-- HTML --> <ul class="parent"> <li>Item 1</li> <li>Item 2</li> </ul> js // Select all <li> elements that are direct children of elements with class "parent" const listItems = $(".parent > li");
7. Sibling Selector
The sibling selector selects elements that are siblings of a specified element. It is denoted by a tilde (~).
html <!-- HTML --> <ul> <li>Item 1</li> <li>Item 2</li> </ul> js // Select all sibling <li> elements after the first <li> element const siblingItems = $("li:first-child ~ li");
8. Filtering Selectors
Filtering selectors allow you to further narrow down the set of matched elements using specific conditions.
8.1. :first and :last
The :first and :last selectors target the first and last elements in a set, respectively.
html <!-- HTML --> <ul> <li>Item 1</li> <li>Item 2</li> </ul> js // Select the first and last <li> elements const firstItem = $("li:first"); const lastItem = $("li:last");
8.2. :even and :odd
The :even and :odd selectors target even and odd elements in a set, respectively.
html <!-- HTML --> <ul> <li>Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> js // Select the even <li> elements (Item 2) const evenItems = $("li:even");
8.3. :not
The :not selector excludes elements that match the specified selector.
html <!-- HTML --> <ul> <li class="special">Special Item</li> <li>Regular Item</li> </ul> js // Select all <li> elements except those with class "special" const regularItems = $("li:not(.special)");
9. Form Selectors
jQuery also provides selectors specifically tailored for form elements.
9.1. :input
The :input selector selects all input, textarea, select, and button elements.
html <!-- HTML --> <input type="text"> <textarea></textarea> <select> <option value="1">Option 1</option> <option value="2">Option 2</option> </select> <button>Click Me</button> js // Select all input, textarea, select, and button elements const formElements = $(":input");
9.2. :text, :password, :radio, :checkbox, :submit, :reset, :button
These selectors target specific types of form elements.
html <!-- HTML --> <input type="text"> <input type="password"> <input type="radio"> <input type="checkbox"> <input type="submit"> <input type="reset"> <button>Click Me</button> js // Select specific types of form elements const textInput = $(":text"); const passwordInput = $(":password"); const radioButton = $(":radio"); // and so onβ¦
Conclusion
In this comprehensive overview, we have explored the world of jQuery selectors. You’ve learned how to use various types of selectors to target and manipulate HTML elements within your web pages. jQuery selectors are a powerful tool in a web developer’s arsenal, enabling you to perform dynamic actions and create interactive user experiences. By mastering jQuery selectors, you can take your web development skills to the next level and build impressive websites with ease.
Start experimenting with jQuery selectors in your projects, and you’ll discover how they simplify your code and enhance the way you interact with the DOM. Whether you’re a beginner or an experienced developer, having a good understanding of jQuery selectors will undoubtedly make you a more proficient web developer. Happy coding!
Remember, this is just the beginning of the exciting world of jQuery. There’s so much more to learn and explore in this incredible JavaScript library, from manipulating elements to handling events and animations. Keep learning, keep experimenting, and continue to expand your horizons as a web developer!
Table of Contents
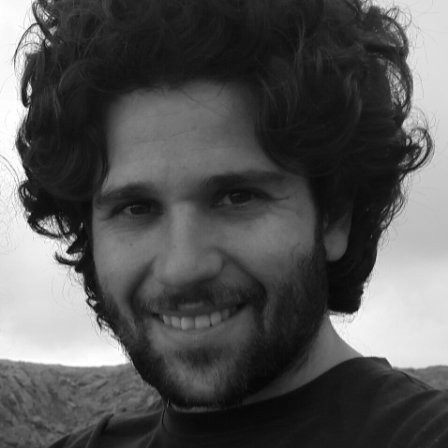
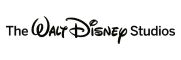