jQuery vs. Vanilla JavaScript: Which is Right for You?
When it comes to web development, JavaScript is the backbone of interactivity and dynamic functionality. Over the years, several libraries and frameworks have emerged to simplify and enhance JavaScript development. Two popular choices are jQuery and Vanilla JavaScript. In this blog post, we’ll dive into the differences between jQuery and Vanilla JavaScript, examining their strengths and weaknesses to help you make an informed decision about which one is right for your next project.
1. Understanding jQuery:
1.1 What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal, event handling, animations, and AJAX interactions. It provides a concise syntax and cross-browser compatibility, making it easier to write complex JavaScript functionalities.
1.2 Pros of jQuery:
- Simplicity: jQuery offers a simplified API, allowing developers to achieve complex tasks with less code compared to Vanilla JavaScript.
- Cross-browser compatibility: jQuery handles browser inconsistencies and provides a consistent API across different browsers.
- DOM manipulation: jQuery excels at manipulating the Document Object Model (DOM), making it easy to traverse and modify HTML elements.
- Animation and effects: jQuery provides a wide range of built-in animations and effects that can be easily applied to web elements.
- AJAX support: jQuery simplifies asynchronous data loading and communication with servers using its AJAX module.
1.3 Cons of jQuery:
- Performance: jQuery is an additional layer on top of JavaScript, which can add some overhead to the execution of code, potentially impacting performance.
- Dependency: Using jQuery requires including the library in your project, which adds to the file size and can slow down initial page loading.
- Learning curve: While jQuery is generally straightforward to use, mastering its advanced features and understanding its inner workings may require additional learning.
- Overkill for simple tasks: If your project only requires basic DOM manipulation or simple animations, the inclusion of jQuery might be unnecessary.
2. Embracing Vanilla JavaScript:
2.1 What is Vanilla JavaScript?
Vanilla JavaScript refers to using plain, unmodified JavaScript without any additional libraries or frameworks. It is the core language that powers the web and offers direct access to all browser APIs and features.
2.2 Pros of Vanilla JavaScript:
- Performance: Without the overhead of a library, Vanilla JavaScript can provide faster execution times and optimize performance.
- Lighter footprint: Using pure JavaScript means avoiding the additional file size and loading time associated with jQuery or any other library.
- Flexibility and control: Vanilla JavaScript gives you complete control over your code and allows you to tailor it precisely to your project’s needs.
- Learning JavaScript fundamentals: Working with Vanilla JavaScript helps you deepen your understanding of the language and its underlying concepts.
- Modern JavaScript features: By using Vanilla JavaScript, you can leverage the latest ECMAScript features directly without relying on library updates.
2.3 Cons of Vanilla JavaScript:
- Cross-browser compatibility: Writing Vanilla JavaScript that works consistently across different browsers can be challenging due to inconsistencies and varying levels of support for new features.
- Longer development time: Achieving certain complex tasks may require writing more code compared to using jQuery, potentially increasing development time.
- Steeper learning curve: Vanilla JavaScript can be more complex for beginners, as it requires a deeper understanding of JavaScript fundamentals and browser APIs.
3. Choosing the Right Approach:
3.1 When to use jQuery:
Legacy projects: If you’re working on a project that already utilizes jQuery extensively, it might be more efficient to stick with it rather than rewriting the entire codebase.
Simple animations and DOM manipulation: For small-scale projects that only require basic animations or DOM manipulation, jQuery’s simplicity and ease of use make it a viable choice.
Cross-browser compatibility: If you need to ensure consistent behavior across different browsers without worrying about compatibility issues, jQuery can save you time and effort.
3.2 When to use Vanilla JavaScript:
- Performance-critical projects: When every millisecond counts and performance is a top priority, using Vanilla JavaScript can provide a performance advantage over jQuery.
- Modern web development: If you want to leverage the latest JavaScript features and best practices without relying on a library, Vanilla JavaScript is the way to go.
- Learning and skill development: If you’re looking to enhance your JavaScript skills or deepen your understanding of the language, working directly with Vanilla JavaScript will be invaluable.
Conclusion:
Choosing between jQuery and Vanilla JavaScript depends on the specific requirements and goals of your project. While jQuery offers a simpler API and cross-browser compatibility, Vanilla JavaScript provides better performance, flexibility, and control. Consider the complexity of your project, performance requirements, and your personal development goals when making a decision. Ultimately, both jQuery and Vanilla JavaScript are powerful tools in their own right, and the choice should be based on what suits your project and development style best.
Remember, whichever approach you choose, the most important aspect is to write clean, maintainable, and efficient code to deliver a seamless user experience in the browser.
Code Sample:
Here’s an example of how to use jQuery to fade out a DOM element:
javascript $(document).ready(function() { $("#myElement").fadeOut(1000, function() { // Animation complete }); });
And here’s the equivalent code using Vanilla JavaScript:
javascript document.addEventListener("DOMContentLoaded", function() { var element = document.getElementById("myElement"); element.style.transition = "opacity 1s"; element.style.opacity = 0; });
Both examples achieve the same result of fading out an element with an ID of “myElement.” The jQuery version utilizes the fadeOut method, while the Vanilla JavaScript version sets the opacity property directly.
Remember to choose the approach that aligns with your project requirements and your personal preferences as a developer. Happy coding!
Table of Contents
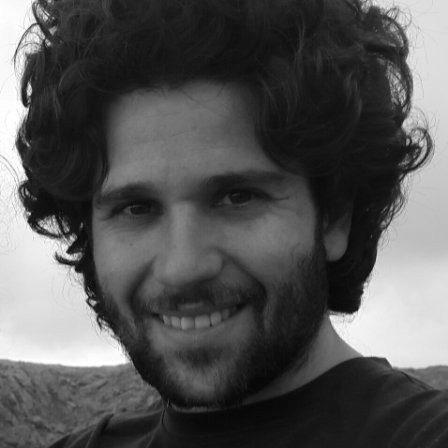
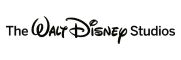