jQuery and Web Accessibility: A Complete Guide
Web accessibility is a fundamental aspect of modern web development. It ensures that websites and web applications are usable by people with disabilities, such as those with visual, auditory, or motor impairments. Building accessible web experiences not only aligns with ethical principles but also ensures compliance with legal requirements in many regions.
Table of Contents
jQuery, a popular JavaScript library, can be a powerful tool in enhancing web accessibility. In this comprehensive guide, we’ll explore the intersection of jQuery and web accessibility. We’ll delve into techniques, best practices, and provide code examples to help you create inclusive web applications.
1. Understanding Web Accessibility
Before diving into jQuery and its role in web accessibility, let’s understand the key concepts of web accessibility.
1.1. What is Web Accessibility?
Web accessibility, often abbreviated as “a11y,” refers to the practice of designing and developing websites and web applications that can be used by people with disabilities. This includes considerations for individuals with visual, auditory, motor, and cognitive impairments.
1.2. Why is Web Accessibility Important?
Web accessibility is crucial for several reasons:
- Inclusivity: It ensures that everyone, regardless of their abilities, can access and use your website or application.
- Legal Compliance: Many countries have laws and regulations that require websites to be accessible. Non-compliance can result in legal consequences.
- Improved User Experience: Accessible websites are often more user-friendly for all users, not just those with disabilities.
1.3. Web Content Accessibility Guidelines (WCAG)
The Web Content Accessibility Guidelines (WCAG) are a set of internationally recognized guidelines for making web content more accessible. These guidelines are organized into four key principles:
- Perceivable: Information and user interface components must be presented in ways that users can perceive.
- Operable: User interface components and navigation must be operable.
- Understandable: Information and operation of the user interface must be understandable.
- Robust: Content must be robust enough to work with current and future technologies.
2. jQuery: An Introduction
jQuery is a fast, small, and feature-rich JavaScript library. It simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. jQuery has been widely adopted by developers for its ease of use and cross-browser compatibility.
2.1. Enhancing Web Accessibility with jQuery
Now that we have a foundational understanding of web accessibility and jQuery, let’s explore how jQuery can be used to enhance web accessibility.
2.1.1. Improving Keyboard Navigation
Keyboard navigation is essential for users who rely on screen readers or have motor impairments. jQuery can help ensure that all interactive elements on your website are keyboard-accessible.
Example: Making a Button Keyboard Accessible
html <button id="myButton" tabindex="0">Click me</button> javascript Copy code $('#myButton').on('keydown', function(e) { if (e.which === 13 || e.which === 32) { // Enter or Space key e.preventDefault(); // Perform the button's action here } });
In this example, we add a keydown event listener to a button element with the ID myButton. When the Enter or Space key is pressed, it triggers the button’s action.
2.1.2. Providing ARIA Roles and Attributes
Accessible Rich Internet Applications (ARIA) is a set of attributes that can be added to HTML elements to improve their accessibility. jQuery can be used to dynamically add ARIA roles and attributes to elements as needed.
Example: Adding ARIA Roles
html <div class="alert" role="alert">This is an important message.</div> javascript $('.alert').attr('aria-live', 'assertive');
In this example, we use jQuery to add the aria-live attribute with the value assertive to a div with the class alert. This indicates that the content inside the div should be announced immediately to screen reader users.
2.1.3. Managing Focus
Properly managing focus is crucial for users who navigate websites using assistive technologies. jQuery can assist in managing focus when elements are shown or hidden dynamically.
Example: Managing Focus when a Modal Opens
html <div id="myModal" class="modal"> <p>Modal content goes here.</p> <button id="closeModal">Close</button> </div> javascript $('#myModal').on('shown.bs.modal', function () { $('#closeModal').focus(); });
In this example, we use jQuery to set focus on the “Close” button when a modal dialog is opened. This ensures that keyboard users are directed to the appropriate element.
2.1.4. Validating Forms Accessibly
Form validation is a critical aspect of web applications. jQuery can help validate forms while ensuring that error messages are accessible to all users.
Example: Accessible Form Validation
html <form id="myForm"> <label for="email">Email:</label> <input type="email" id="email" required> <div id="emailError" class="error" role="alert"></div> <button type="submit">Submit</button> </form> javascript $('#myForm').on('submit', function (e) { e.preventDefault(); const email = $('#email').val(); if (!isValidEmail(email)) { $('#emailError').text('Please enter a valid email address.'); $('#emailError').attr('aria-hidden', 'false'); } else { $('#emailError').text(''); $('#emailError').attr('aria-hidden', 'true'); // Submit the form } });
In this example, jQuery is used to validate the email input field and display an error message when necessary. The aria-hidden attribute is dynamically set to ensure screen readers announce or ignore the error message appropriately.
2.1.5. Handling Dynamic Content
Modern web applications often load content dynamically. When doing so, it’s essential to ensure that this content is accessible and that screen readers can detect changes.
Example: Adding ARIA Live Regions for Dynamic Content
html <div id="dynamicContent" aria-live="polite"></div> <button id="loadContent">Load Content</button> javascript $('#loadContent').on('click', function () { // Load dynamic content into #dynamicContent $('#dynamicContent').html('New content loaded.'); });
In this example, we use the aria-live attribute with the value polite to indicate that changes to the dynamicContent div should be announced by screen readers in a non-intrusive manner.
3. Testing for Accessibility
Enhancing web accessibility with jQuery is a step in the right direction, but it’s equally important to test your website thoroughly to ensure its accessibility. Several tools and techniques can help with accessibility testing.
3.1. Automated Testing Tools
There are various automated accessibility testing tools available that can scan your website for common accessibility issues. Some popular options include:
- axe-core: A JavaScript library that can be integrated into your testing framework to automate accessibility testing.
- WAVE: A browser extension that provides instant accessibility evaluation of web content.
- Lighthouse: A tool built into Google Chrome’s DevTools that offers accessibility audits and recommendations.
3.2. Manual Testing
Automated tools can catch many accessibility issues, but manual testing is essential for uncovering more nuanced problems. Here are some manual testing techniques:
- Keyboard Testing: Navigate your website using only the keyboard to ensure all interactive elements are accessible.
- Screen Reader Testing: Use screen reader software, such as JAWS or NVDA, to listen to how your website is experienced by users with visual impairments.
- Color Contrast Testing: Verify that text and background colors have sufficient contrast for readability.
3.3. User Testing
User testing involves real people with disabilities using your website and providing feedback. This can uncover issues that automated and manual testing might miss.
Conclusion
Web accessibility is a vital aspect of web development, and jQuery can play a significant role in enhancing it. By following best practices, implementing ARIA attributes, managing focus, and testing rigorously, you can ensure that your web applications are inclusive and accessible to all users.
Remember that web accessibility is an ongoing commitment. As technologies evolve and guidelines are updated, it’s essential to stay informed and continue improving the accessibility of your web projects. jQuery can be a valuable tool in this journey, helping you create web experiences that are truly for everyone.
Incorporating web accessibility into your development process not only makes your website compliant with legal standards but also opens your content to a wider audience, making the web a more inclusive place for all.
So, embrace the power of jQuery in creating accessible web applications, and contribute to a more inclusive online world.
Table of Contents
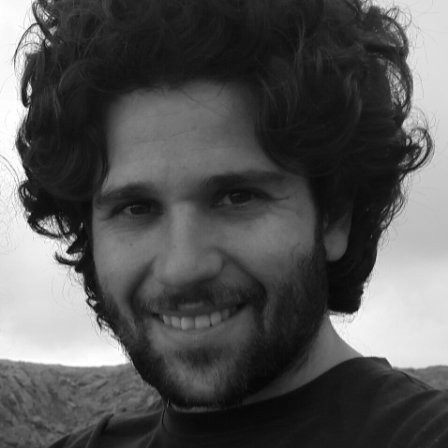
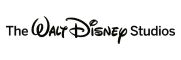