jQuery 101: Getting Started with the Ultimate Web Development Tool
In today’s rapidly evolving web development landscape, having a versatile and efficient toolkit is crucial. jQuery, an open-source JavaScript library, has been a staple in web development for over a decade. With its simplified syntax, powerful features, and extensive community support, jQuery remains the go-to choice for developers worldwide. If you’re new to jQuery or looking to brush up on your skills, this comprehensive guide will walk you through the basics and empower you to harness jQuery’s full potential.
1. What is jQuery?
jQuery is a fast, concise, and feature-rich JavaScript library that simplifies HTML document traversing, event handling, and animating. It was created by John Resig in 2006 and has since become immensely popular due to its ability to streamline web development tasks and enhance user experience. jQuery provides a high-level abstraction layer over JavaScript, making it easier to perform common operations on HTML documents.
2. Why Should You Use jQuery?
There are several compelling reasons why jQuery has stood the test of time and remains an invaluable tool for web developers:
- Cross-browser Compatibility: jQuery takes care of the nuances and inconsistencies across different web browsers, allowing developers to write code that works seamlessly across various platforms.
- Simplified Syntax: jQuery’s concise syntax makes it easier to write and read code. With jQuery, you can accomplish complex tasks with fewer lines of code compared to pure JavaScript.
- Extensive Plugin Ecosystem: jQuery boasts a vast ecosystem of plugins that can be easily integrated into your projects. These plugins offer additional functionality, such as image sliders, form validations, and rich UI components, saving you time and effort.
- Animation and Effects: jQuery simplifies the process of creating engaging animations and effects, bringing life to your web pages and enhancing user interactions.
- AJAX Support: jQuery provides powerful AJAX capabilities, enabling you to make asynchronous requests to the server and update your web pages dynamically without a full page reload.
3. Getting Started with jQuery
3.1 Installing jQuery:
To start using jQuery, you first need to download the library or include it from a content delivery network (CDN). The easiest way to get started is by using a CDN, as it provides several advantages, such as faster load times and increased chances of the library being cached by the user’s browser. Here’s an example of including jQuery from the Google CDN:
html <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
3.2 Including jQuery in Your Web Page:
After including the jQuery library in your HTML file, you can start utilizing its features. The most common approach is to enclose your jQuery code within the $(document).ready() function, ensuring that your code executes once the HTML document is fully loaded. Here’s an example:
javascript $(document).ready(function() { // Your jQuery code goes here });
4. Selectors and DOM Manipulation
4.1 Understanding Selectors:
One of jQuery’s greatest strengths lies in its ability to select and manipulate elements in the Document Object Model (DOM) easily. jQuery provides a wide range of selectors, allowing you to target elements based on their tag name, class, ID, attributes, and more. Here are some examples of commonly used selectors:
Selecting elements by tag name:
javascript $('p') // Selects all <p> elements
Selecting elements by class name:
javascript $('.classname') // Selects all elements with the class name 'classname'
Selecting elements by ID:
javascript $('#elementID') // Selects the element with the ID 'elementID'
4.2 Manipulating the DOM:
Once you’ve selected elements using jQuery’s selectors, you can manipulate them in various ways. jQuery provides numerous methods to modify element attributes, styles, content, and structure. Here’s an example that changes the text and background color of a button when it’s clicked:
javascript $(document).ready(function() { $('button').click(function() { $(this).text('Clicked!'); $(this).css('background-color', 'red'); }); });
In the example above, the click() function attaches a click event handler to all <button> elements. When a button is clicked, its text is changed to “Clicked!” and its background color is set to red.
5. Event Handling:
Event handling is a fundamental aspect of web development, and jQuery simplifies the process of attaching event handlers to elements. With jQuery, you can easily listen for user interactions, such as clicks, keypresses, and mouse movements. Here’s an example of binding a click event handler to a button:
javascript $(document).ready(function() { $('button').click(function() { // Your code to handle the button click goes here }); });
In the example above, the click() function is used to bind a click event handler to all <button> elements. When a button is clicked, the code inside the event handler function will be executed.
6. Animations and Effects:
Adding animations and effects to your web pages can greatly enhance the user experience. jQuery provides a range of methods to create smooth animations and apply effects to elements. Whether you want to fade elements in and out, slide them up and down, or create custom animations, jQuery has you covered. Here’s an example of fading in a <div> element when a button is clicked:
javascript $(document).ready(function() { $('button').click(function() { $('div').fadeIn(); }); });
In the example above, the fadeIn() function is used to gradually fade in a <div> element when the button is clicked. jQuery provides a suite of animation methods like fadeOut(), slideUp(), and animate() to bring your web pages to life.
7. AJAX and Asynchronous Operations:
Asynchronous JavaScript and XML (AJAX) enables you to update parts of your web page without refreshing the entire page. jQuery simplifies AJAX requests by providing a range of methods to handle asynchronous operations. You can fetch data from a server, send data to a server, and update your web page dynamically. Here’s an example of making an AJAX request and updating a <div> element with the response:
javascript $(document).ready(function() { $('button').click(function() { $.ajax({ url: 'https://api.example.com/data', method: 'GET', success: function(response) { $('div').text(response); } }); }); });
In the example above, when the button is clicked, an AJAX GET request is made to the specified URL. Upon receiving a successful response, the <div> element’s content is updated with the response text.
8. Plugins and Extensibility:
jQuery’s extensibility is a significant advantage. The library’s plugin ecosystem offers a wide range of ready-to-use solutions for common web development tasks. These plugins can add functionality like image sliders, date pickers, form validations, and much more to your web pages. By leveraging the power of plugins, you can save development time and enhance your projects. Integrating a jQuery plugin into your code is typically as simple as including the plugin script and initializing it with a few lines of code.
9. Best Practices for Using jQuery:
To make the most of jQuery, it’s essential to follow some best practices:
- Minify and Concatenate: Minify your jQuery code to reduce file size and improve page load times. Concatenating multiple JavaScript files into one also reduces HTTP requests.
- Use Event Delegation: Instead of attaching event handlers to individual elements, use event delegation to attach them to a parent element. This improves performance, especially when dealing with dynamically created elements.
- Optimize DOM Manipulation: Minimize DOM manipulation by chaining jQuery methods and performing multiple changes in a single operation. This reduces browser reflows and improves performance.
- Update to the Latest Version: Stay up to date with the latest jQuery version to benefit from bug fixes, performance improvements, and new features.
Conclusion:
jQuery remains an indispensable tool for web developers, offering a wealth of features and simplifying complex tasks. With its extensive documentation, active community, and vast plugin ecosystem, jQuery provides a solid foundation for building modern and interactive web applications. By mastering the basics covered in this guide and exploring advanced techniques, you’ll unlock the full potential of jQuery and elevate your web development skills to new heights.
Whether you’re a beginner or an experienced developer, jQuery’s versatility and ease of use make it a valuable asset in your web development toolkit. Start exploring the world of jQuery today and take your web development projects to the next level. Happy coding!
Table of Contents
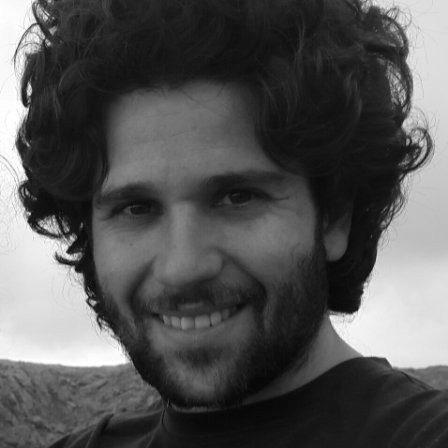
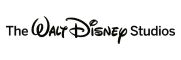