Optimizing Web Performance with Lazy Loading and jQuery
In today’s fast-paced digital landscape, user experience plays a pivotal role in determining the success of a website. One of the key factors that contribute to a seamless and enjoyable user experience is web performance. Slow-loading websites can drive away visitors, negatively impacting engagement and conversion rates. To address this challenge, developers have turned to various techniques, and among them, lazy loading with jQuery has emerged as a powerful strategy for enhancing web performance.
Table of Contents
1. Understanding Lazy Loading
Lazy loading is a technique that defers the loading of non-critical resources, such as images, videos, and other assets, until they are actually needed. Instead of loading all resources when a user visits a page, lazy loading loads only the resources that are visible within the user’s viewport. As the user scrolls down, additional resources are loaded on demand, leading to faster initial page load times and reduced bandwidth usage.
2. Benefits of Lazy Loading
Implementing lazy loading in your web development projects offers several noteworthy benefits:
2.1. Faster Initial Page Load
Lazy loading prevents the browser from downloading all resources at once, which significantly reduces the time it takes for a page to become usable. This is particularly crucial for content-heavy websites that feature numerous images and multimedia elements.
2.2. Improved User Experience
By loading only the visible content initially, lazy loading enhances the user experience by allowing visitors to start interacting with the page sooner. This helps in reducing bounce rates and increasing engagement.
2.3. Reduced Bandwidth Consumption
Loading only the essential resources minimizes the amount of data transferred between the server and the user’s device. This is especially valuable for users on slower connections or limited data plans.
2.4. Optimal Performance for Mobile Users
Mobile devices often have slower network connections compared to desktops. Lazy loading caters to this scenario, ensuring that mobile users can access and interact with your content swiftly.
2.5. Enhanced SEO and Search Rankings
Search engines consider page load speed as a ranking factor. Implementing lazy loading can positively impact your website’s SEO, potentially leading to higher search rankings and increased organic traffic.
3. Implementing Lazy Loading with jQuery
jQuery, a popular JavaScript library, provides a straightforward way to implement lazy loading on your website. The process involves manipulating the loading behavior of specific elements, typically images and videos, by leveraging jQuery’s built-in functions. Here’s how you can do it:
3.1. Include jQuery in Your Project
To get started, make sure you include the jQuery library in your project. You can either download the jQuery file and link to it in your HTML or use a Content Delivery Network (CDN) to access it.
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
3.2. Identify Elements to Lazy Load
Assign a class or data attribute to the elements you want to lazy load. For instance, let’s consider a set of images with the class “lazy-img”.
html <img class="lazy-img" data-src="image.jpg" alt="Lazy-loaded Image"> <img class="lazy-img" data-src="another-image.jpg" alt="Another Lazy-loaded Image"> <!-- More lazy-loaded images -->
3.3. Write the jQuery Script
Now, write a jQuery script that detects when the lazy-loadable elements come into the viewport and replace the “data-src” attribute with the “src” attribute. This triggers the actual loading of the resource.
javascript $(document).ready(function() { $(window).on('scroll', function() { $('.lazy-img').each(function() { if (isElementInViewport(this)) { $(this).attr('src', $(this).data('src')); $(this).removeClass('lazy-img'); } }); }); }); function isElementInViewport(el) { var rect = el.getBoundingClientRect(); return ( rect.top >= 0 && rect.left >= 0 && rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) && rect.right <= (window.innerWidth || document.documentElement.clientWidth) ); }
In this script, the function isElementInViewport checks if an element is currently within the viewport. The jQuery script listens for the scroll event and triggers the lazy loading process for elements that are visible in the viewport.
3.4. Add Styles for Smooth Transition (Optional)
To enhance the user experience, you can add CSS styles to create a smooth transition when the lazy-loaded images appear on the screen.
css .lazy-img { opacity: 0; transition: opacity 0.5s; } .lazy-img.loaded { opacity: 1; }
By applying these styles, the images will gradually fade in as they are loaded, providing a visually pleasing effect.
4. Best Practices for Lazy Loading with jQuery
While implementing lazy loading with jQuery can greatly boost your website’s performance, it’s essential to follow best practices to ensure optimal results:
4.1. Prioritize Critical Resources
Although lazy loading is effective, it’s crucial to prioritize loading critical resources that are essential for the initial user experience. This includes crucial stylesheets, scripts, and content. Lazy load non-essential resources to avoid blocking the rendering of critical elements.
4.2. Test on Various Devices and Browsers
Different devices and browsers may handle lazy loading differently. Test your implementation across a range of devices, including mobile phones and tablets, to ensure consistent performance.
4.3. Monitor Performance Metrics
Regularly monitor your website’s performance metrics, such as page load times, bounce rates, and user engagement. Analyze the data to assess the impact of lazy loading and make any necessary adjustments.
4.4. Provide Fallback Content
In cases where JavaScript is disabled or fails to load, ensure that users still have access to the content. Include noscript tags with alternative content or utilize the “noscript” CSS selector to display static images.
4.5. Keep jQuery Updated
jQuery releases updates to improve functionality and security. Stay up-to-date with the latest version to benefit from optimizations and bug fixes.
Conclusion
Web performance is a critical aspect of delivering a seamless and engaging user experience. Lazy loading, combined with the power of jQuery, offers an effective way to optimize your website’s performance by loading resources only when they are needed. By following best practices and incorporating lazy loading into your development workflow, you can create faster, more responsive websites that delight users and drive better results. Embrace lazy loading and jQuery to supercharge your web performance and stay ahead in the competitive online landscape.
Table of Contents
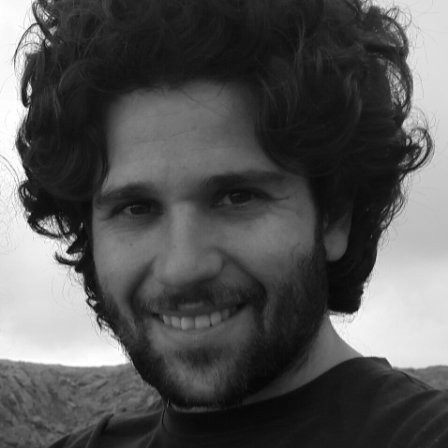
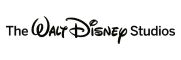