Pusher Powers Laravel: Create Dynamic, Real-Time Web Applications
In the modern web development landscape, real-time data broadcasting is no longer a luxury—it’s a necessity. Laravel, a popular PHP framework, simplifies the implementation of real-time events through its broadcasting feature. Pair this with Pusher Channels, and you have a robust solution for delivering real-time updates to your users. This blog post explores how to leverage Laravel Broadcasting with Pusher Channels to create dynamic, real-time web applications.You can hire Laravel developers for your projects to ensure greater success.
Table of Contents
1. What is Laravel Broadcasting?
Laravel Broadcasting allows you to broadcast your server-side Laravel events to your client-side web application. It makes use of WebSockets to establish a connection between your server and the client, enabling real-time data transmission without the need for polling.
2. Pusher Channels: A Brief Overview
Pusher Channels is a real-time messaging service that allows you to add real-time features to your web and mobile applications. It manages and scales WebSocket connections, ensuring reliable and secure communication between the client and server.
3. Setting Up Laravel with Pusher Channels
To get started, you first need to set up Pusher Channels in your Laravel project.
- Install Pusher PHP Server SDK:
```bash composer require pusher/pusher-php-server ```
- Configure `.env` File:
Add your Pusher credentials to your `.env` file:
``` PUSHER_APP_ID=your-app-id PUSHER_APP_KEY=your-app-key PUSHER_APP_SECRET=your-app-secret PUSHER_APP_CLUSTER=your-app-cluster ```
- Configure `broadcasting.php`:
In `config/broadcasting.php`, set the default broadcaster to Pusher:
```php 'default' => env('BROADCAST_DRIVER', 'pusher'), ```
4. Creating and Broadcasting Events
In Laravel, each broadcast event is represented by a class. Let’s create a simple event.
- Create an Event:
```bash php artisan make:event OrderStatusUpdated ```
- Implement `ShouldBroadcast`:
Update the event class to implement `ShouldBroadcast` and define the data to broadcast:
```php use Illuminate\Contracts\Broadcasting\ShouldBroadcast; class OrderStatusUpdated implements ShouldBroadcast { public $orderId; public $status; public function __construct($orderId, $status) { $this->orderId = $orderId; $this->status = $status; } public function broadcastOn() { return new Channel('orders'); } } ```
5. Frontend Integration
On the frontend, you need to listen for the event:
- Install Pusher JS Library:
```html <script src="https://js.pusher.com/7.0/pusher.min.js"></script> ```
- Subscribe to the Channel:
```javascript var pusher = new Pusher('your-app-key', { cluster: 'your-app-cluster' }); var channel = pusher.subscribe('orders'); channel.bind('OrderStatusUpdated', function(data) { alert('Order ' + data.orderId + ' status updated to ' + data.status); }); ```
6. Broadcasting an Event
To broadcast an event, you simply dispatch it from your Laravel application:
```php event(new OrderStatusUpdated($orderId, 'shipped')); ```
7. Additional Resources
- Laravel Official Documentation on Broadcasting:
The Laravel documentation provides a thorough overview of broadcasting, including its concepts, setup, and usage. It’s a valuable resource for understanding technical details and best practices directly from the official source. Laravel Broadcasting Documentation
- Pusher Channels Official Documentation:
Pusher’s documentation is crucial for comprehending how to implement Pusher Channels in different scenarios, including with Laravel. It includes detailed API references, setup guides, and best practices. Pusher Channels Documentation
- Tutorial on Laravel and Pusher Integration:
DigitalOcean provides a practical tutorial on creating a web-based notification system using Laravel and Pusher. This tutorial is great for readers seeking hands-on, applied knowledge and examples. How To Create Web Notifications Using Laravel and Pusher Channels
Conclusion
Integrating Laravel Broadcasting with Pusher Channels is a powerful way to enhance your web applications with real-time features. Whether it’s updating order statuses, chat messages, or live notifications, the combination of Laravel and Pusher provides a seamless and scalable solution.
You can check out our other blog posts to learn more about Laravel. We bring you a complete guide titled Laravel Development: Best Practices and Tips for Success along with the Exploring Laravel’s Eloquent ORM: Simplify Database Operations and Laravel Caching: Boost Performance with Data Caching Techniques which will help you understand and gain more insight into the Laravel framework.
Table of Contents
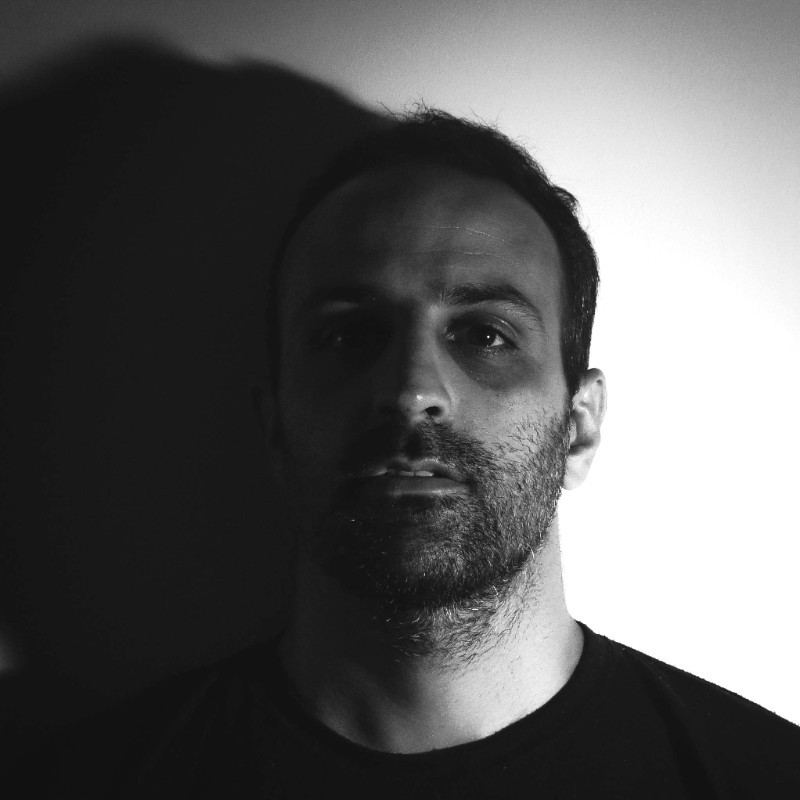
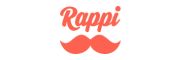