Transform Your Web Testing Approach with Advanced Laravel Dusk Insights
In the realm of web application development, testing plays a pivotal role in ensuring quality and functionality. Laravel, a robust PHP framework, offers a powerful tool for browser testing named Laravel Dusk. Laravel Dusk not only simplifies the process of writing browser tests but also opens the door to advanced testing techniques that can significantly improve the quality of your web applications. You can hire Laravel developers for your projects to ensure greater success.
Table of Contents
1. Understanding Laravel Dusk
Laravel Dusk is an expressive, easy-to-use browser automation and testing API. It provides a fluent testing API for browser-based applications and is capable of interacting with your application just like a real user would.
2. Setting Up Laravel Dusk
To get started with Laravel Dusk, you first need to install it via Composer:
```shell composer require --dev laravel/dusk ```
Once installed, you can use the `dusk:install` Artisan command to set up Dusk:
```shell php artisan dusk:install ```
3. Advanced Techniques in Laravel Dusk
3.1. Page Object Model
The Page Object Model (POM) is a design pattern that makes tests more readable and maintainable. In this pattern, each page is represented by a class. These classes encapsulate the page structure and behavior, thus separating the test logic from the UI specifics.
Example:
Here’s an example of a Page Object for a login page:
```php class LoginPage extends Page { public function url() { return '/login'; } public function login(Browser $browser, $email, $password) { $browser->visit($this->url()) ->type('email', $email) ->type('password', $password) ->press('Login'); } } ```
3.2. JavaScript and AJAX Testing
Laravel Dusk simplifies the process of testing JavaScript and AJAX operations. With Dusk, you can wait for elements to appear on the page, which is crucial for testing asynchronous operations.
Example:
Testing a button that loads data via AJAX:
```php $browser->click('#load-data-button') ->waitFor('.data-container') ->assertSee('Loaded Data'); ```
3.3. Data Seeding for Tests
Consistent data is essential for reliable tests. Laravel allows you to seed your database with test data, ensuring that your tests run against a predictable dataset.
Example:
Seeding a user table before running tests:
```php $this->artisan('db:seed', ['--class' => 'UserTableSeeder']); ```
3.4. Custom Assertions
Laravel Dusk allows you to create custom assertions to extend its capabilities. These can be tailored to your application’s specific needs.
Example:
Creating a custom assertion to check if a user is logged in:
```php Browser::macro('assertLoggedIn', function () { $this->assertAuthenticated(); }); ```
3.5. Continuous Integration and Deployment
Integrating Laravel Dusk tests into your CI/CD pipeline ensures that your application is thoroughly tested with each deployment. Tools like Jenkins, Travis CI, and GitHub Actions can be used to automate this process.
4. Debugging Dusk Tests
Debugging failing tests in Laravel Dusk can be challenging. However, Dusk provides several tools to help with this:
– Screenshots: Automatically take screenshots of your browser when a test fails.
– Console Output: Capture console output to understand JavaScript errors.
– Laravel Logs: Review application logs for insights into the application behavior during tests.
5. Best Practices
- Keep Tests Independent: Each test should be able to run independently without relying on the state created by another test.
- Use Factories for Test Data: Laravel’s model factories are a great way to create test data. This ensures your tests are working with realistic data models.
- Clean Up After Tests: Ensure that each test cleans up after itself, leaving the database and the application state as it was before.
Conclusion
Advanced browser testing with Laravel Dusk can significantly improve the reliability and quality of your web applications. By employing techniques such as the Page Object Model, JavaScript testing, custom assertions, and integrating tests into your CI/CD pipeline, you can create a robust and maintainable test suite.
Laravel Dusk stands out as a powerful tool in the Laravel ecosystem, making browser testing an accessible and integral part of the development process. With these advanced techniques, you can ensure that your Laravel applications are thoroughly tested and ready for production.
External Resources:
- Laravel Dusk Official Documentation – The official guide and reference for Laravel Dusk.
- Effective Browser Testing Strategies – An in-depth look at browser testing methodologies.
- Advanced Automated Testing Techniques – A resource for various automated testing approaches, including Laravel Dusk.
You can check out our other blog posts to learn more about Laravel. We bring you a complete guide titled Laravel Development: Best Practices and Tips for Success along with the Exploring Laravel’s Eloquent ORM: Simplify Database Operations and Laravel Caching: Boost Performance with Data Caching Techniques which will help you understand and gain more insight into the Laravel framework.
Table of Contents
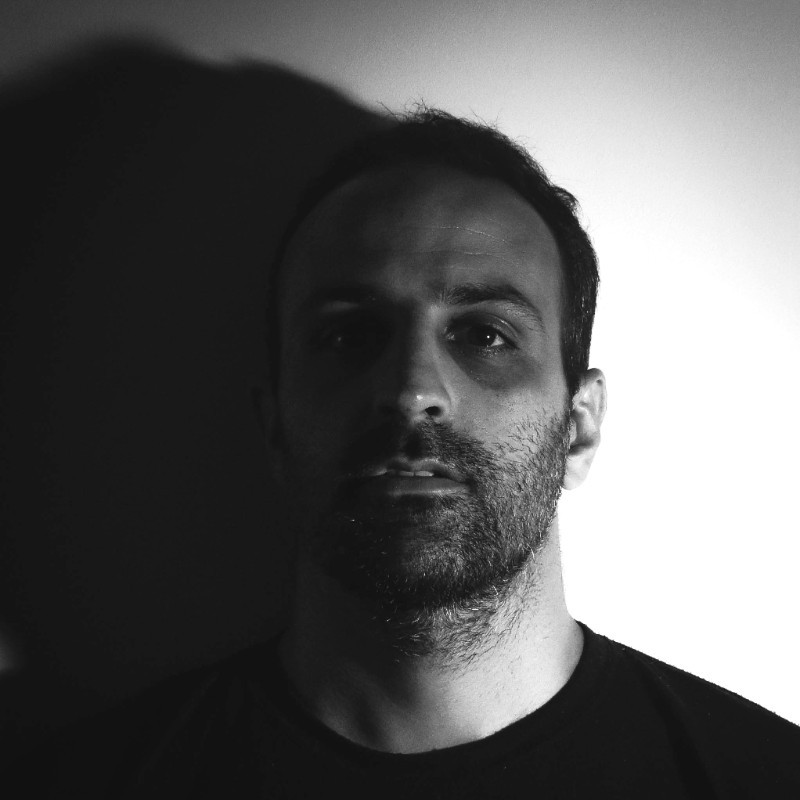
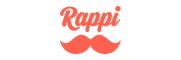