Laravel Queues Meets Amazon SQS: Transform Your Application’s Efficiency
In the fast-paced world of web development, efficiently handling background tasks is crucial for application performance. Laravel, a robust PHP framework, offers an elegant queuing system that simplifies asynchronous task execution. When combined with Amazon Simple Queue Service (SQS), a fully managed message queuing service, Laravel’s queuing capabilities become significantly more scalable and reliable. This synergy is essential for developers looking to enhance their application’s performance and scalability. You can hire Laravel developers for your projects to ensure greater success.
Table of Contents
1. Understanding Laravel Queues
Laravel’s queue system provides a unified API across a variety of different queue backends. Queues allow you to defer the processing of a time-consuming task, such as sending an email, until a later time, thereby speeding up web requests to your application.
2. Overview of Amazon SQS
Amazon SQS is a scalable, fully managed message queuing service offered by Amazon Web Services (AWS). It enables the decoupling and scaling of microservices, distributed systems, and serverless applications. With SQS, you can send, store, and receive messages between software components without losing messages and without requiring other services to be available.
3. Integrating Laravel Queues with Amazon SQS
To integrate Laravel Queues with Amazon SQS, you need to follow these steps:
- Set up an AWS account and create an SQS queue: First, sign up for an AWS account and create a new SQS queue. Note down the queue URL and access credentials.
- Configure Laravel: In your Laravel application, update the `config/queue.php` file to set SQS as the default queue driver and input your SQS credentials and queue URL.
4. Real-World Examples
Imagine you’re developing an e-commerce application where you need to process orders. You can use Laravel Queues with Amazon SQS to handle order processing in the background. Here’s a simple code snippet to demonstrate this:
```php // In your OrderController public function processOrder(Request $request) { // Validate and create order ... // Dispatch order processing job to queue ProcessOrderJob::dispatch($order); } ```
In this example, `ProcessOrderJob` would be a job class that handles the logic of order processing.
5. Best Practices and Advanced Tips
– Batch Processing: Utilize batch processing for bulk operations to reduce the number of API calls.
– Error Handling: Implement robust error handling in your job classes to manage failed jobs effectively.
6. Comparative Analysis
While other queue systems like Redis or database queues are suitable for certain scenarios, Amazon SQS stands out for its scalability, reliability, and ease of use in distributed environments.
Conclusion
Integrating Laravel Queues with Amazon SQS is a powerful approach to handling background tasks in a scalable and efficient manner. By leveraging these technologies, developers can significantly enhance the performance and reliability of their web applications.
References and Further Reading:
- Laravel Documentation on Queues – Official Laravel documentation providing in-depth insights into Laravel Queues.
- Amazon SQS Developer Guide – Comprehensive guide on Amazon SQS, including best practices and setup instructions.
- Scalable Laravel: Using Queues with Amazon SQS – An insightful blog post
You can check out our other blog posts to learn more about Laravel. We bring you a complete guide titled Laravel Development: Best Practices and Tips for Success along with the Exploring Laravel’s Eloquent ORM: Simplify Database Operations and Laravel Caching: Boost Performance with Data Caching Techniques which will help you understand and gain more insight into the Laravel framework.
Table of Contents
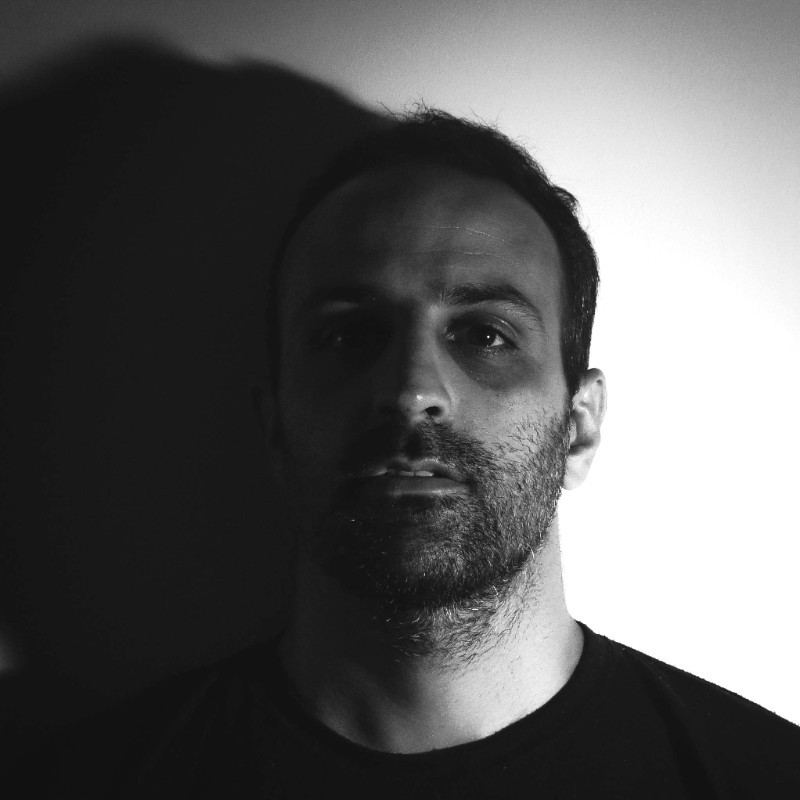
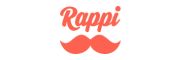