Streamline Your Laravel Apps with Beanstalkd: A Step-by-Step Guide
In the world of web development, efficiency and speed are paramount. Laravel, a robust PHP framework, and Beanstalkd, a simple yet powerful queueing system, combine to provide an elegant solution for handling background job processing. This blog post will dive deep into how Laravel queues work with Beanstalkd to enhance your application’s performance. You can hire Laravel developers for your projects to ensure greater success.
Table of Contents
1. What is Laravel Queues?
Laravel Queues is a feature within the Laravel framework that allows you to defer the processing of a time-consuming task, such as sending emails, until a later time. This non-blocking approach significantly improves web application performance.
2. Why Beanstalkd?
Beanstalkd is a lightweight and easy-to-use queueing system. Its simplicity in design and protocol translates to lower overheads and efficient job handling, making it a popular choice for Laravel developers. Learn more about Beanstalkd [here](https://beanstalkd.github.io/).
3. Setting Up Laravel with Beanstalkd
To begin using Laravel Queues with Beanstalkd, you first need to set up Beanstalkd on your server. Installation instructions can be found [here](https://beanstalkd.github.io/download.html). Once installed, configure your `.env` file in Laravel to use Beanstalkd as the queue driver:
```env QUEUE_CONNECTION=beanstalkd ```
3.1. Creating Jobs
In Laravel, jobs are simple PHP classes where the actual work is defined. You can generate a new job class using the Artisan CLI:
```bash php artisan make:job ProcessOrder ```
This command will create a new job class in the `app/Jobs` directory.
3.2. Writing a Job Class
Here’s an example of a basic job class:
```php namespace App\Jobs; use Illuminate\Bus\Queueable; use Illuminate\Contracts\Queue\ShouldQueue; use Illuminate\Foundation\Bus\Dispatchable; use Illuminate\Queue\InteractsWithQueue; use Illuminate\Queue\SerializesModels; class ProcessOrder implements ShouldQueue { use Dispatchable, InteractsWithQueue, Queueable, SerializesModels; protected $order; public function __construct(Order $order) { $this->order = $order; } public function handle() { // Process the order... } } ```
3.3. Dispatching Jobs
You can dispatch a job using the `dispatch` method:
```php ProcessOrder::dispatch($order); ```
3.4. Processing Jobs
Run the queue worker to start processing jobs:
```bash php artisan queue:work beanstalkd ```
4. Advantages of Using Laravel Queues with Beanstalkd
- Asynchronous Processing: Allows for handling tasks such as email notifications in the background, improving user experience.
- Scalability: Easy to scale and manage large numbers of background jobs.
- Reliability: Beanstalkd ensures that jobs are processed at least once.
5. Monitoring and Troubleshooting
For monitoring and managing Beanstalkd queues, tools like Beanstalkd Console are quite handy.
Conclusion
Laravel Queues combined with Beanstalkd offers a robust solution for managing background job processing in your applications. This setup not only improves the performance of your applications but also provides scalability and reliability.
– For a more comprehensive guide on Laravel Queues, visit the official Laravel documentation
– To deep dive into Beanstalkd and its features, check out this comprehensive guide.
– For real-world implementations and case studies, explore Laravel News, a site dedicated to news and tutorials related to Laravel.
Implementing Laravel Queues with Beanstalkd in your projects can significantly enhance your application’s efficiency. The combination of Laravel’s elegant syntax and Beanstalkd’s simplicity makes background job processing a breeze. Whether you’re sending batch emails, generating reports, or handling data synchronization, this duo will ensure your tasks are handled smoothly in the background.
You can check out our other blog posts to learn more about Laravel. We bring you a complete guide titled Laravel Development: Best Practices and Tips for Success along with the Exploring Laravel’s Eloquent ORM: Simplify Database Operations and Laravel Caching: Boost Performance with Data Caching Techniques which will help you understand and gain more insight into the Laravel framework.
Table of Contents
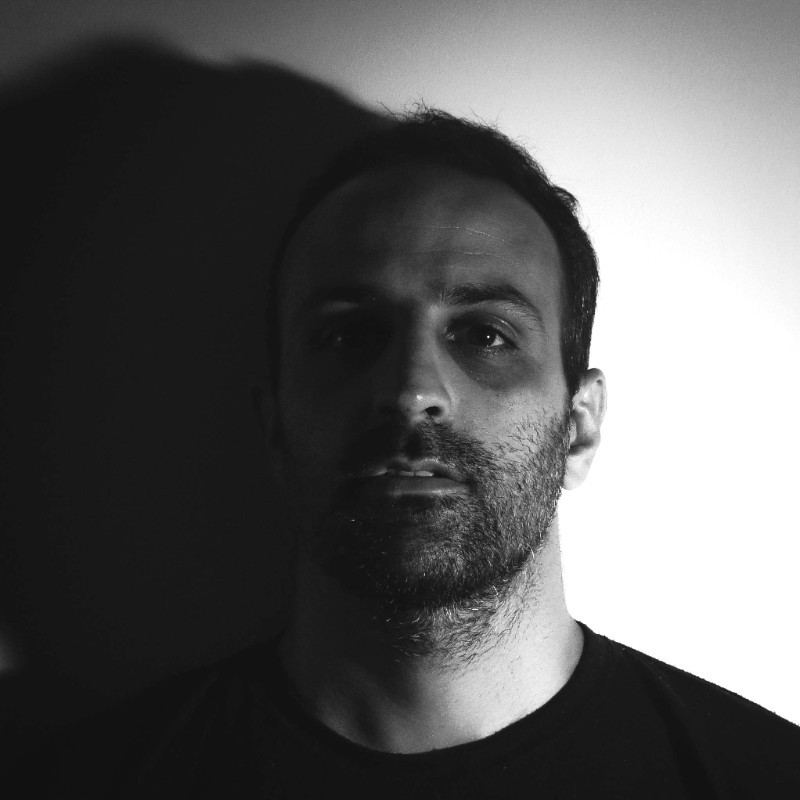
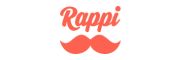