Next-Level Searching: How to Utilize Scout and Elasticsearch in Laravel
In the world of web development, the ability to efficiently search and manage data is crucial. Laravel Scout, in combination with Elasticsearch, offers a robust solution for implementing advanced search capabilities in your applications. You can hire Laravel developers for your projects to ensure greater success. In this blog post, we’ll explore how you can leverage these tools to enhance your Laravel applications.
Table of Contents
1. Understanding Laravel Scout and Elasticsearch
Laravel Scout is a powerful, full-text search package for Laravel. It provides a simple, driver-based solution for adding search functionality to Eloquent models. When coupled with Elasticsearch, a distributed, RESTful search and analytics engine, Scout becomes an even more powerful tool.
2. Getting Started
2.1. Prerequisites
– Laravel installation
– Elasticsearch server
2.2. Setting Up Laravel Scout with Elasticsearch
- Install Scout: Begin by installing Laravel Scout via Composer: `composer require laravel/scout`.
- Install Elasticsearch Driver: For Elasticsearch integration, install a compatible package like `babenkoivan/scout-elasticsearch-driver`.
- Configure Scout: Set your driver to Elasticsearch in `config/scout.php`.
3. Advanced Search Capabilities
3.1. Full-Text Search
Full-text search is the core feature of Elasticsearch. Implement it in Laravel Scout by defining searchable attributes in your model.
```php class Post extends Model { use Searchable; public function toSearchableArray() { return ['title' => $this->title, 'content' => $this->content]; } } ```
3.2. Complex Queries
Elasticsearch supports complex queries like boolean queries, range queries, and more. Laravel Scout allows you to harness these capabilities:
```php $posts = Post::search('Laravel') ->where('status', 'published') ->whereBetween('views', [100, 500]) ->get(); ```
3.3. Aggregations
Elasticsearch’s aggregation framework enables you to generate analytics based on your data. Scout simplifies the implementation:
```php use ScoutElastic\Aggregatable; class Post extends Model { use Searchable, Aggregatable; } ```
3.4. Autocomplete and Suggestions
Implement autocomplete features using Elasticsearch’s suggesters. This feature enhances user experience by providing real-time search suggestions.
3.5. Geo-Searches
Elasticsearch’s geo-search capabilities allow you to perform searches based on geographical data. This is particularly useful for location-based applications.
4. Best Practices
– Index Management: Regularly update and maintain your indices for optimal performance.
– Relevance Tuning: Adjust scoring and relevancy settings in Elasticsearch to improve search results.
– Testing: Ensure thorough testing of your search features to maintain quality and accuracy.
5. Real-World Applications
- E-Commerce Platforms: Enhance product search with filters, ranges, and autocomplete suggestions.
- Content Management Systems: Implement full-text search across articles, blogs, and other content types.
- Geo-Location Services: Offer location-based search functionalities.
For a more comprehensive guide on Laravel and Elasticsearch:
– Laravel Scout Documentation: Laravel Scout Official Docs
– Elasticsearch: Elasticsearch Official Website
– Elasticsearch PHP Client: Elasticsearch PHP Client on GitHub
Conclusion
Integrating Laravel Scout with Elasticsearch unlocks a vast array of advanced search features that can significantly enhance your Laravel applications. From full-text search to complex queries and geo-searches, the combination of these tools offers a powerful, scalable solution for managing and searching data.
Remember, the key to successful implementation lies in understanding both Laravel Scout and Elasticsearch, and carefully planning your search features to align with your application’s requirements. Happy coding!
You can check out our other blog posts to learn more about Laravel. We bring you a complete guide titled Laravel Development: Best Practices and Tips for Success along with the Exploring Laravel’s Eloquent ORM: Simplify Database Operations and Laravel Caching: Boost Performance with Data Caching Techniques which will help you understand and gain more insight into the Laravel framework.
Table of Contents
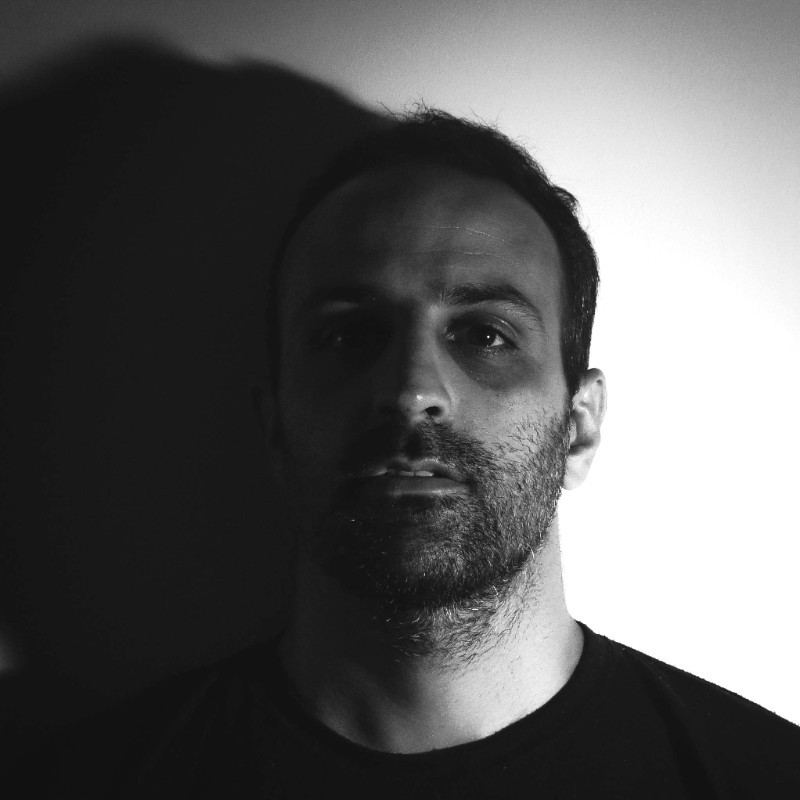
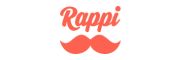