Transform User Experience in Laravel with MeiliSearch’s Instant Search Results
In the dynamic world of web applications, providing fast and relevant search results is crucial for enhancing user experience. Laravel, a popular PHP framework, offers a powerful solution for this through Laravel Scout, an engine-agnostic search solution. When combined with MeiliSearch, an open-source, lightweight search engine, Laravel Scout becomes even more potent, delivering quick, relevant search results with minimal setup. This blog post explores how Laravel Scout and MeiliSearch work together, complete with practical examples. You can hire Laravel developers for your projects to ensure greater success.
Table of Contents
1. What is Laravel Scout?
Laravel Scout provides a simple, driver-based solution for adding full-text search to your Eloquent models. By default, Scout uses Algolia; however, it’s flexible enough to be paired with other search engines like MeiliSearch. The primary benefit of using Scout is its simplicity and ease of integration with Laravel applications.
2. Key Features
– Driver-based: Easily switch between various search engine drivers.
– Model synchronization: Automatically syncs search indexes with Eloquent models.
– Simple configuration: Minimal setup required to get started.
3. MeiliSearch: A Quick Overview
MeiliSearch is a RESTful search API that is fast, easy to use, and highly customizable. It’s particularly well-suited for small to medium-sized projects, offering features like typo tolerance, filters, and a high degree of relevancy in search results.
4. Why Choose MeiliSearch with Laravel Scout?
– Speed: MeiliSearch is designed for speed, ensuring quick search responses.
– Typo Tolerance: It can handle typographical errors, providing relevant results despite mistakes in the query.
– Easy Integration: Seamlessly integrates with Laravel Scout.
5. Integrating MeiliSearch with Laravel Scout
Step 1: Installation
First, install Laravel Scout and the MeiliSearch PHP client via Composer:
```shell composer require laravel/scout meilisearch/meilisearch-php http-client ```
Next, publish the Scout configuration file:
```shell php artisan vendor:publish --provider="Laravel\Scout\ScoutServiceProvider" ```
Step 2: Configuration
Configure your `.env` file with MeiliSearch credentials:
```plaintext SCOUT_DRIVER=meilisearch MEILISEARCH_HOST=http://127.0.0.1:7700 MEILISEARCH_KEY=masterKey ```
Step 3: Model Indexing
In your Eloquent model, use the `Searchable` trait:
```php use Laravel\Scout\Searchable; class Post extends Model { use Searchable; // Model content } ```
Step 4: Searching
Perform searches on your model:
```php $posts = Post::search('query')->get(); ```
6. Practical Examples
Example 1: Basic Search
```php $results = Product::search('iPhone')->get(); ```
This simple query searches for ‘iPhone’ in the `Product` model.
Example 2: Paginated Results
```php $books = Book::search('fiction')->paginate(); ```
Pagination is essential for handling large datasets.
Example 3: Conditional Clauses
```php $users = User::search('John')->where('age', '>', 25)->get(); ```
Conditional clauses refine search results further.
7. Advanced Features
MeiliSearch offers advanced search functionalities like filters, sorting, and custom ranking rules. Laravel Scout allows developers to leverage these features with ease.
Conclusion
Combining Laravel Scout with MeiliSearch provides a robust, flexible, and efficient search solution for Laravel applications. Its ease of use, coupled with the powerful search capabilities of MeiliSearch, makes it an excellent choice for developers looking to enhance their search functionality.
Further Reading;
- MeiliSearch Documentation: For a comprehensive understanding of MeiliSearch’s features and configurations, visit the official MeiliSearch documentation at MeiliSearch Documentation.
- Laravel Scout Official Documentation: To explore the functionalities and features offered by Laravel Scout, you can refer to the Laravel Scout documentation at Laravel Scout Documentation
- Comprehensive Guide on Integrating MeiliSearch with Laravel: For step-by-step tutorials and advanced use cases on integrating MeiliSearch with Laravel, check out the guide at Integrating MeiliSearch with Laravel.
You can check out our other blog posts to learn more about Laravel. We bring you a complete guide titled Laravel Development: Best Practices and Tips for Success along with the Exploring Laravel’s Eloquent ORM: Simplify Database Operations and Laravel Caching: Boost Performance with Data Caching Techniques which will help you understand and gain more insight into the Laravel framework.
Table of Contents
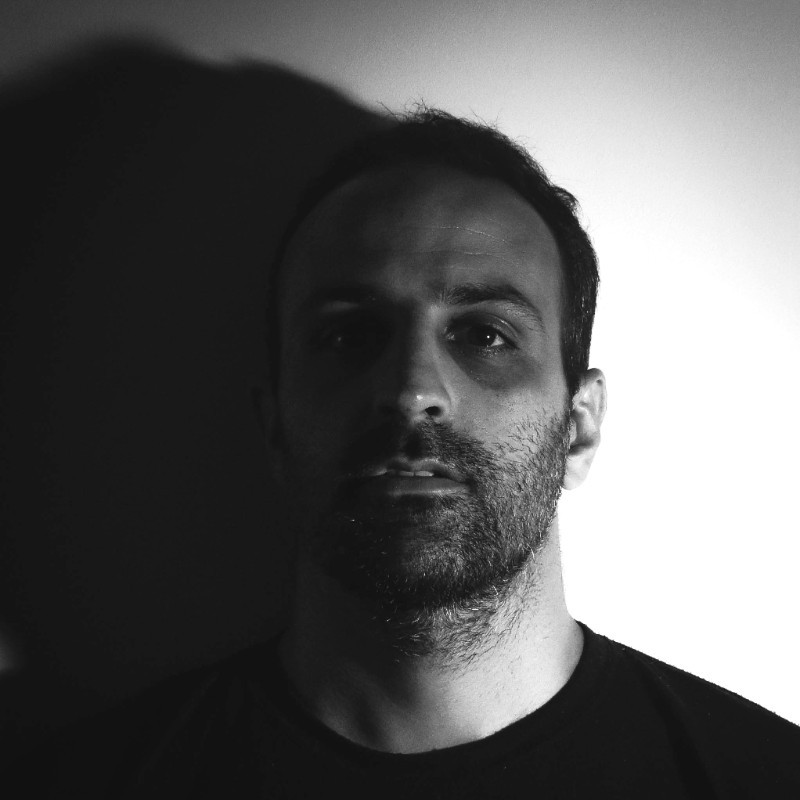
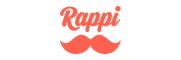