Transform Your Laravel Apps with Expert Webhook Management
Webhooks are a powerful tool for modern web applications, enabling real-time communication between different systems. In the Laravel framework, webhooks can be utilized to manage both incoming and outgoing requests efficiently. This blog post delves into the specifics of handling webhooks in Laravel, providing practical examples and external resources for further exploration. You can hire Laravel developers for your projects to ensure greater success.
Table of Contents
1. Understanding Webhooks
Before diving into Laravel’s implementation, let’s understand what webhooks are. Webhooks are user-defined HTTP callbacks that are triggered by specific events in a source application. They allow for real-time data transmission between applications, making them essential for integrations and automated workflows.
2. Key Concepts
– Event: A specific action or occurrence in an application that triggers a webhook.
– Listener: The component in the receiving application that listens for incoming webhook requests.
3. Webhooks in Action
Imagine an e-commerce platform (built with Laravel) that needs to update an external inventory management system whenever a new order is placed. A webhook can be set up to trigger on the ‘order placed’ event, sending the relevant data to the inventory system in real-time.
4. Setting Up Laravel for Webhooks
Laravel, being a robust PHP framework, offers seamless integration with webhooks. To set up webhooks in Laravel, you’ll need to follow a series of steps:
4.1. Environment Setup
Make sure your Laravel environment is ready. If you’re new to Laravel, follow the official Laravel installation guide.
4.2. Handling Incoming Webhooks
- Route Configuration: Define routes in `routes/web.php` to handle incoming webhook requests.
```php Route::post('/webhook', 'WebhookController@handle'); ```
- Creating a Controller: Generate a controller to process the incoming data.
```php php artisan make:controller WebhookController ```
- Controller Logic: Implement the logic to handle different types of webhook events.
```php public function handle(Request $request) { // Process the request } ```
4.3. Handling Outgoing Webhooks
- Event Setup: Define an event in Laravel that triggers a webhook.
```php Event::listen(OrderPlaced::class, SendOrderToInventory::class); ```
- Listener Implementation: Create a listener that sends the HTTP request to the target application.
```php public function handle(OrderPlaced $event) { // Send HTTP request to the external system } ```
5. Security Considerations
When dealing with webhooks, security is paramount. Ensure you validate incoming requests and secure outgoing requests. Laravel provides several tools for this, such as middleware for authentication and encryption methods for data security.
6. Advanced Techniques
6.1. Queueing Webhook Requests
To improve performance, especially with high-volume applications, consider queueing webhook requests. Laravel’s queue system can handle this efficiently.
6.2. Testing Webhooks
Testing is crucial for reliable webhook integration. Laravel provides testing facilities that simulate webhook events and responses.
7. Real-World Examples
Let’s look at some practical examples of handling webhooks in Laravel.
Example 1: Processing Payment Gateway Notifications
When integrating with a payment gateway like Stripe, you can set up a webhook to listen for payment success or failure notifications.
Example 2: Syncing User Data with CRM
If you have a CRM system, you can use webhooks to sync user data from your Laravel application whenever a user updates their profile.
Conclusion
Webhooks are an integral part of modern web application architecture. Laravel, with its comprehensive features, makes it easy to handle both incoming and outgoing webhook requests. By following best practices and ensuring security, you can leverage webhooks to create more dynamic and integrated applications.
Further Reading:
– Laravel Official Documentation: Webhook Handling
– Webhook Best Practices: WebhookGuide.com
– Advanced Webhook Techniques: Laravel News Blog
You can check out our other blog posts to learn more about Laravel. We bring you a complete guide titled Laravel Development: Best Practices and Tips for Success along with the Exploring Laravel’s Eloquent ORM: Simplify Database Operations and Laravel Caching: Boost Performance with Data Caching Techniques which will help you understand and gain more insight into the Laravel framework.
Table of Contents
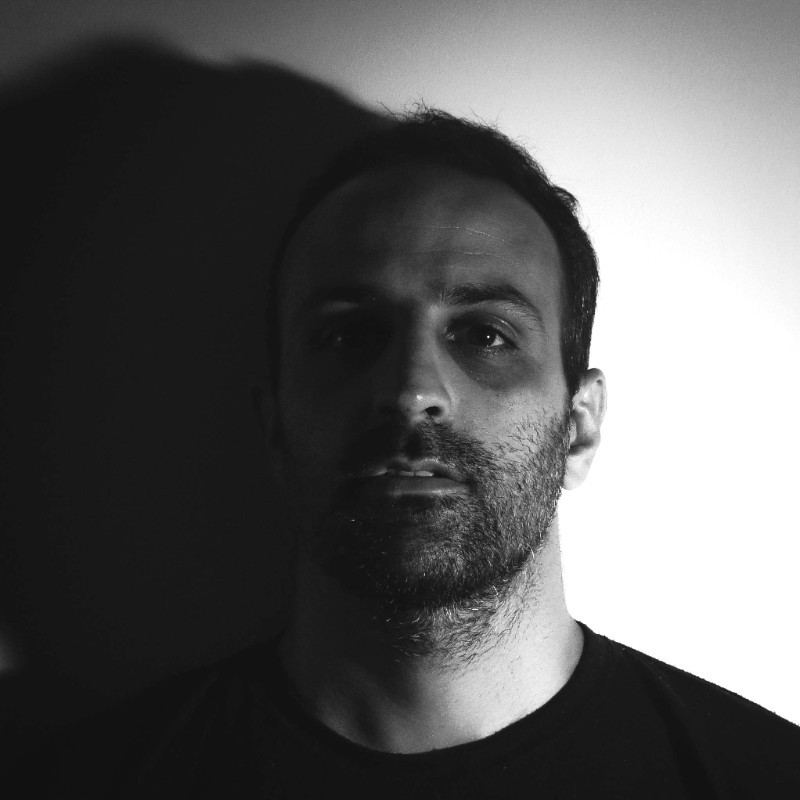
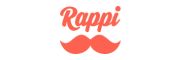