Mastering SEO: How to Build Search-Engine Friendly Websites with Next.js
Search Engine Optimization (SEO) is an essential part of any online strategy. It’s the art of making your website discoverable and readable by search engines, thus improving your organic ranking and attracting more relevant traffic. While there are various ways to achieve this, one of the most efficient methods is to use a front-end framework designed for SEO optimization. Enter Next.js.
Next.js is an open-source development framework built on Node.js, allowing for server-side rendering (SSR) and generating static sites for React-based web applications. The SEO advantage here is clear: unlike client-side rendering, where content is loaded after the user arrives on the page, server-side rendering allows for content to be preloaded. This means search engines can easily index your site.
However, mastering Next.js and its myriad features requires expertise. Therefore, for businesses seeking to harness the full potential of Next.js, hiring dedicated Next.js developers can be an invaluable investment. They possess the know-how to build and optimize your site for maximum SEO benefit, ensuring your website is easily discoverable, efficient, and user-friendly.
In the following sections, we explore how to build SEO-friendly websites with Next.js, providing practical examples. This will offer insight into the kind of sophisticated work a skilled Next.js developer can accomplish for your business.
1. Server-Side Rendering (SSR)
With SSR, you can render the initial content of your page on the server. This way, search engines can see and index the fully rendered page, rather than an empty div as in the case of client-side rendering.
Here’s a simple example of a Next.js page using SSR:
```javascript export async function getServerSideProps(context) { const res = await fetch(`https://api.example.com/data`); const data = await res.json(); if (!data) { return { notFound: true, }; } return { props: { data }, }; } function Page({ data }) { return ( <div> <h1>{data.title}</h1> <p>{data.description}</p> </div> ); } export default Page; ```
In this example, `getServerSideProps` fetches the data needed for the page before rendering it. This data is then passed as props to the `Page` component, which renders the data on the server.
2. Static Site Generation (SSG)
Next.js also provides the option for Static Site Generation (SSG). SSG pre-renders pages at build time, creating a static HTML file for each page. This can further speed up page load times, improve SEO, and give a more performant user experience.
Here’s a basic SSG example:
```javascript export async function getStaticProps() { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data, }, revalidate: 1, }; } function HomePage({ data }) { return ( <div> <h1>{data.title}</h1> <p>{data.description}</p> </div> ); } export default HomePage; ```
The `getStaticProps` function works at build time, creating an HTML page for the ‘HomePage’. The `revalidate` option means the data will be refreshed every 1 second (useful for dynamic data).
3. Dynamic Routes
With dynamic routes, you can generate multiple pages based on data fetched from an API or a database. This is especially useful for blog posts or product pages.
Here’s a basic example:
```javascript // pages/posts/[id].js export async function getStaticPaths() { const res = await fetch('https://api.example.com/posts'); const posts = await res.json(); const paths = posts.map((post) => ({ params: { id: post.id.toString() }, })); return { paths, fallback: false }; } export async function getStaticProps({ params }) { const res = await fetch(`https://api.example.com/posts/${params.id}`); const post = await res.json(); return { props: { post } }; } const Post = ({ post }) => ( <div> <h1>{post.title}</h1> <p>{post.content}</p> </div> ); export default Post; ```
In this example, `getStaticPaths` fetches all posts and generates paths for each one. `getStaticProps` then fetches data for each individual post.
4. Head Component
Next.js has a built-in `Head` component that can be used to define meta tags, which are crucial for SEO. You can dynamically set the title, description, canonical URL, and other SEO-related meta tags for each page.
Here’s an example:
```javascript import Head from 'next/head'; function HomePage() { return ( <div> <Head> <title>Your Website Title</title> <meta name="description" content="Your Website Description" /> <link rel="canonical" href="https://www.yourwebsite.com" /> </Head> <h1>Welcome to our website!</h1> </div> ); } export default HomePage; ```
In this example, the `Head` component is used to set the page’s title, description, and canonical URL.
5. Image Optimization
Next.js provides an `Image` component that automatically optimizes images in various ways: resizing, optimizing quality, and adding modern formats like WebP. Optimized images can reduce page load time and improve your website’s SEO ranking.
Here’s an example:
```javascript import Image from 'next/image'; function HomePage() { return ( <div> <h1>Welcome to our website!</h1> <Image src="/path/to/your/image.jpg" alt="Description of Image" width={500} height={300} /> </div> ); } export default HomePage; ```
In this example, the `Image` component is used to display an image that will be automatically optimized by Next.js.
Conclusion
Next.js is an exceptional framework for building SEO-friendly websites due to its capability for server-side rendering, static site generation, and various optimization features. These elements can drastically improve your website’s performance, loading time, and ultimately, your SEO ranking.
For businesses looking to leverage these advantages, hiring skilled Next.js developers can be a game-changer. They can adeptly navigate the features of this powerful framework, building a high-performance, SEO-friendly website that propels your business forward. By understanding and effectively utilizing these features, a proficient Next.js developer ensures your website is as SEO-friendly as possible, paving the way for enhanced visibility and user engagement.
Don’t miss out on the opportunity to improve your web presence. Consider hiring Next.js developers and unlock the full potential of your online platform.
Table of Contents
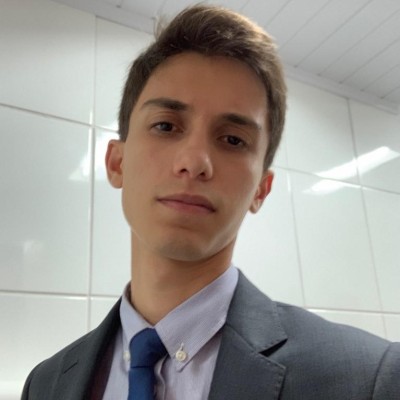
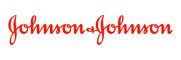