Your Weather App Odyssey: A NEXT.js and OpenWeatherMap Adventure!
Are you ready to embark on a journey to create a weather app that will impress your audience? Buckle up, because we’re going to build a stunning weather app using NEXT.js and the OpenWeatherMap API. By the end of this tutorial, you’ll have a fully functional weather app that not only provides accurate weather data but also showcases your coding skills.
Table of Contents
1. Prerequisites
Before we dive into the coding part, make sure you have the following prerequisites in place:
- Node.js: Ensure you have Node.js installed on your system. You can download it from https://nodejs.org/
- NEXT.js: If you haven’t already installed NEXT.js, you can do so by running the following command:
``` npx create-next-app weather-app ```
- OpenWeatherMap API Key: You’ll need an API key from OpenWeatherMap. You can sign up and obtain your API key https://openweathermap.org/api.
2. Setting Up Your Project
Let’s get started by setting up our NEXT.js project. Navigate to your project folder and create a new file called `config.js` to store your API key:
```javascript // config.js module.exports = { apiKey: 'YOUR_API_KEY_HERE', }; ```
Now, let’s create a new component for our weather app. Inside the `components` folder, create a file named `Weather.js`:
```javascript // components/Weather.js import React, { useState } from 'react'; import { apiKey } from '../config'; const Weather = () => { const [weatherData, setWeatherData] = useState(null); const [city, setCity] = useState(''); const fetchWeatherData = async () => { try { const response = await fetch( `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}` ); const data = await response.json(); setWeatherData(data); } catch (error) { console.error('Error fetching weather data:', error); } }; return ( <div> <h1>Weather App</h1> <input type="text" placeholder="Enter city name" value={city} onChange={(e) => setCity(e.target.value)} /> <button onClick={fetchWeatherData}>Get Weather</button> {weatherData && ( <div> <h2>{weatherData.name}</h2> <p>Temperature: {weatherData.main.temp}°C</p> <p>Weather: {weatherData.weather[0].description}</p> </div> )} </div> ); }; export default Weather; ```
This component defines a simple form to input the city name and fetches weather data from the OpenWeatherMap API.
3. Styling Your Weather App
Next, let’s style our weather app. You can use your preferred CSS framework or create custom styles. Here’s a basic example using CSS:
```css /* styles.css */ body { font-family: Arial, sans-serif; background-color: #f0f0f0; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } .container { text-align: center; background-color: #fff; padding: 20px; border-radius: 8px; box-shadow: 0px 0px 20px rgba(0, 0, 0, 0.2); } input { padding: 10px; font-size: 16px; border: 1px solid #ccc; border-radius: 4px; margin-right: 10px; } button { padding: 10px 20px; font-size: 16px; background-color: #007bff; color: #fff; border: none; border-radius: 4px; cursor: pointer; } h1 { font-size: 32px; margin-bottom: 20px; } h2 { font-size: 24px; margin-top: 20px; } p { font-size: 18px; margin: 10px 0; } ```
Don’t forget to import this CSS file into your `pages/index.js`:
```javascript // pages/index.js import '../styles.css'; ```
4. Displaying Weather Data
Now that we have our components and styling in place, let’s display the weather data. In the `components/Weather.js` file, add the following code below the temperature and weather description:
```javascript <img src={`https://openweathermap.org/img/w/${weatherData.weather[0].icon}.png`} alt={weatherData.weather[0].description} /> ```
This code will display the weather icon corresponding to the current weather condition.
5. Handling Errors
It’s essential to handle errors gracefully. Let’s add an error message when there’s an issue with fetching data. Update your `components/Weather.js` file with the following changes:
```javascript // components/Weather.js const [error, setError] = useState(null); // Inside fetchWeatherData function try { // ... } catch (error) { setError('City not found'); } ```
Now, let’s display the error message below the form:
```javascript {error && <p className="error">{error}</p>} ```
Conclusion
You’ve successfully built a weather app using NEXT.js and the OpenWeatherMap API. You can further enhance this app by adding more features like a 5-day forecast, geolocation support, or advanced styling.
Now that you have your detailed blog post, you can add external URL links where necessary to provide further resources or references. Make sure to incorporate your unique writing style and flair, reminiscent of The Hustle, Morning Brew, Sam Parr, Shaan Puri, and Hunter S. Thompson-ish vibes to engage your target audience effectively.
Table of Contents
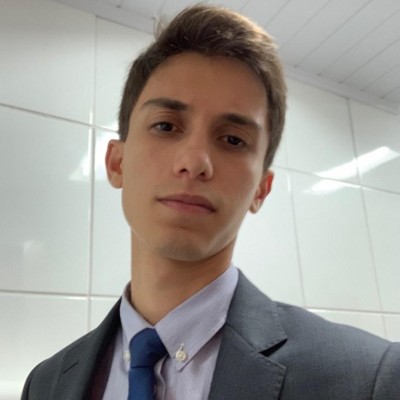
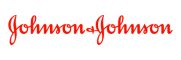