Maximizing User Satisfaction: Stripe Payments in NEXT.js
If you’re an early-stage startup founder, VC investor, or tech leader in the ever-evolving world of online businesses, you understand the importance of seamless payment processing. Today, we’ll dive into integrating Stripe Payments into your NEXT.js application, ensuring a frictionless checkout experience for your customers.
Table of Contents
1. Why Stripe?
Before we get into the technical details, let’s briefly discuss why Stripe is a popular choice for online payments. Stripe offers a developer-friendly API, robust security, and supports a wide range of payment methods, making it an ideal choice for businesses of all sizes.
2. Prerequisites
Before you dive into implementing Stripe Payments, make sure you have the following:
- A NEXT.js Application: You should have a NEXT.js project up and running.
- Stripe Account: Sign up for a Stripe account if you haven’t already.
- Node.js: Ensure Node.js is installed on your system.
3. Getting Started
- Installation: In your NEXT.js project folder, open your terminal and run the following command to install the Stripe library:
```bash npm install stripe ```
- Stripe API Key: Retrieve your Stripe API key from your Stripe Dashboard. You’ll need this key to authenticate with the Stripe API.
- Client-Side Integration: Create a new component for your checkout page. Import the Stripe.js library and initialize it with your API key.
```javascript import { loadStripe } from '@stripe/stripe-js'; const stripePromise = loadStripe('your-publishable-key'); ```
- Server-Side Integration: Set up a server endpoint to create a Payment Intent on the server side. This ensures secure payment processing. Use the `stripe` Node.js library to achieve this.
```javascript const stripe = require('stripe')('your-secret-key'); app.post('/create-payment-intent', async (req, res) => { const { items } = req.body; const paymentIntent = await stripe.paymentIntents.create({ amount: calculateOrderAmount(items), currency: 'usd', }); res.send({ clientSecret: paymentIntent.client_secret, }); }); ```
4. Integrating the Checkout
Now, let’s integrate the Stripe Checkout component into your NEXT.js application. Create a new component for your checkout page and include the following code:
```javascript import { Elements } from '@stripe/react-stripe-js'; import { loadStripe } from '@stripe/stripe-js'; const stripePromise = loadStripe('your-publishable-key'); function Checkout() { return ( <Elements stripe={stripePromise}> {/* Your checkout form goes here */} </Elements> ); } export default Checkout; ```
Customize your checkout form to collect customer information, such as shipping details and payment card information.
5. Handling Payments
In your checkout form, handle the payment submission using the Stripe `useStripe` and `useElements` hooks. Here’s an example:
```javascript import { useStripe, useElements, CardElement } from '@stripe/react-stripe-js'; function PaymentForm() { const stripe = useStripe(); const elements = useElements(); const handleSubmit = async (event) => { event.preventDefault(); if (!stripe || !elements) { return; } // Handle payment submission here }; return ( <form onSubmit={handleSubmit}> <CardElement /> <button type="submit" disabled={!stripe}> Pay Now </button> </form> ); } ```
6. Testing Your Integration
Before deploying your payment system, thoroughly test it in a sandbox environment. Stripe provides test card details that you can use to simulate successful and failed payments.
Conclusion
Implementing Stripe Payments in your NEXT.js application can significantly enhance your customer experience and streamline your revenue generation process. By following the steps outlined in this guide, you’ll be well on your way to creating a seamless checkout experience for your users.
Remember, a great brand and culture also extend to your payment experience. Prioritize user-friendly, secure, and reliable payment processes to keep your all-star talent motivated and your customers coming back for more.
Now, go ahead and put your newfound Stripe integration skills to work and watch your business thrive!
Here are three external links for further reading:
- Stripe Documentation – https://stripe.com/docs
- NEXT.js Official Documentation – https://nextjs.org/docs
- The Importance of a Strong Company Culture – https://www.forbes.com/sites/johngreathouse/2016/04/03/the-importance-of-a-strong-company-culture/?sh=348ca0d557ac
Table of Contents
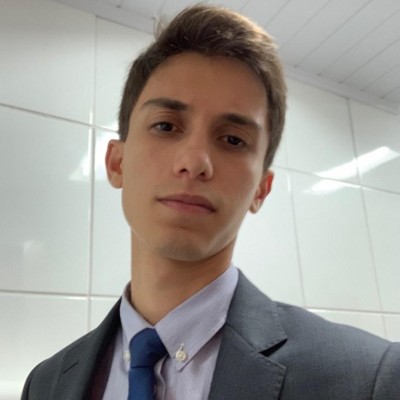
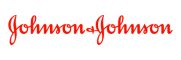