React Native and Android Permissions: Handling User Permissions
In today’s mobile app development landscape, user privacy and security are paramount. When building React Native apps for Android, it’s crucial to understand how to handle user permissions gracefully. In this blog, we’ll dive into the world of Android permissions and explore how to manage them in your React Native applications. From understanding permission types to implementing best practices and code samples, we’ve got you covered.
Table of Contents
1. Understanding Android Permissions
Before we dive into the code, it’s essential to understand the basics of Android permissions. Android permissions are safeguards that protect user privacy by controlling access to sensitive device resources or data. There are various types of permissions, permission groups, and different rules governing their usage.
1.1. Types of Permissions
Android permissions can be broadly categorized into two types:
- Normal Permissions: These permissions are granted automatically when your app is installed. They pose minimal risk to user privacy, as users are not explicitly asked for their consent during installation. Examples of normal permissions include accessing the internet or setting the device’s time zone.
- Dangerous Permissions: Dangerous permissions, on the other hand, are considered sensitive and require user consent. These permissions include accessing the device’s camera, microphone, location, and contacts. If your app attempts to use dangerous permissions, it must request them at runtime.
1.2. Permission Groups
Permissions are organized into permission groups, making it easier to manage and request permissions with similar purposes. For instance, the “Location” permission group includes permissions related to accessing the device’s GPS or network-based location.
1.3. Runtime vs. Normal Permissions
As mentioned earlier, normal permissions are automatically granted during installation, while dangerous permissions must be requested at runtime. This runtime permission model provides users with more control over their data and privacy. Users can grant or deny permissions on a per-use basis.
2. Requesting Permissions
Now that we have a good understanding of Android permissions, let’s dive into the process of requesting permissions in React Native.
2.1. Checking and Requesting Permissions
Before you can use a dangerous permission, you need to check if it’s already granted and, if not, request it. React Native provides a module called PermissionsAndroid to handle permissions.
Here’s a code snippet demonstrating how to check and request a single permission:
javascript import { PermissionsAndroid } from 'react-native'; async function checkAndRequestCameraPermission() { try { const granted = await PermissionsAndroid.request( PermissionsAndroid.PERMISSIONS.CAMERA, { title: 'Camera Permission', message: 'This app needs access to your camera to take photos.', buttonPositive: 'OK', buttonNegative: 'Cancel', } ); if (granted === PermissionsAndroid.RESULTS.GRANTED) { console.log('Camera permission granted'); // You can now use the camera } else { console.log('Camera permission denied'); // Handle the denial gracefully } } catch (err) { console.warn(err); } }
In this example, we first import the PermissionsAndroid module and create an asynchronous function checkAndRequestCameraPermission(). Inside the function, we use PermissionsAndroid.request to request the camera permission. We provide a title, message, and buttons for the permission dialog.
2.2. Handling Permission Responses
When the user responds to the permission request, the PermissionsAndroid.request function returns a result, which can be one of the following constants:
- PermissionsAndroid.RESULTS.GRANTED: The user granted the permission.
- PermissionsAndroid.RESULTS.DENIED: The user denied the permission.
- PermissionsAndroid.RESULTS.NEVER_ASK_AGAIN: The user denied the permission and selected the “Never ask again” option.
It’s crucial to handle these responses gracefully to provide a good user experience. In the code sample above, we log whether the permission was granted or denied.
3. Best Practices for Handling Permissions
Now that we’ve covered the basics of requesting permissions, let’s discuss some best practices to ensure a seamless user experience in your React Native app.
3.1. Explain Why You Need Permissions
When you request dangerous permissions, it’s essential to explain why your app needs them. Clearly communicate to the user why granting the permission is necessary for the app’s functionality. This increases the likelihood of the user granting the permission.
3.2. Handle Permission Denials Gracefully
Not all users will grant the permissions you request. Some may deny them, and that’s okay. Your app should gracefully handle permission denials and provide an alternative user experience when certain permissions are not available.
3.3. Checking and Requesting Multiple Permissions
In some cases, your app may require multiple permissions to function correctly. You can check and request multiple permissions using the PermissionsAndroid.requestMultiple method. Here’s a code snippet that demonstrates how to do this:
javascript import { PermissionsAndroid } from 'react-native'; async function checkAndRequestPermissions() { try { const permissions = await PermissionsAndroid.requestMultiple([ PermissionsAndroid.PERMISSIONS.CAMERA, PermissionsAndroid.PERMISSIONS.READ_CONTACTS, ]); if ( permissions[PermissionsAndroid.PERMISSIONS.CAMERA] === PermissionsAndroid.RESULTS.GRANTED && permissions[PermissionsAndroid.PERMISSIONS.READ_CONTACTS] === PermissionsAndroid.RESULTS.GRANTED ) { console.log('All permissions granted'); // Your app can now use the requested permissions } else { console.log('Some permissions were denied'); // Handle the denial of permissions } } catch (err) { console.warn(err); } }
In this example, we use PermissionsAndroid.requestMultiple to request both camera and contacts permissions. We then check if all the requested permissions were granted or if some were denied.
4. Code Samples
Let’s wrap things up with a couple of code samples that illustrate how to request permissions in React Native.
4.1. Requesting a Single Permission
Here’s a summarized version of the earlier code snippet for requesting the camera permission:
javascript import { PermissionsAndroid } from 'react-native'; async function checkAndRequestCameraPermission() { try { const granted = await PermissionsAndroid.request( PermissionsAndroid.PERMISSIONS.CAMERA, { title: 'Camera Permission', message: 'This app needs access to your camera to take photos.', buttonPositive: 'OK', buttonNegative: 'Cancel', } ); if (granted === PermissionsAndroid.RESULTS.GRANTED) { console.log('Camera permission granted'); // You can now use the camera } else { console.log('Camera permission denied'); // Handle the denial gracefully } } catch (err) { console.warn(err); } }
4.2. Requesting Multiple Permissions
And here’s how you can request multiple permissions at once:
javascript import { PermissionsAndroid } from 'react-native'; async function checkAndRequestPermissions() { try { const permissions = await PermissionsAndroid.requestMultiple([ PermissionsAndroid.PERMISSIONS.CAMERA, PermissionsAndroid.PERMISSIONS.READ_CONTACTS, ]); if ( permissions[PermissionsAndroid.PERMISSIONS.CAMERA] === PermissionsAndroid.RESULTS.GRANTED && permissions[PermissionsAndroid.PERMISSIONS.READ_CONTACTS] === PermissionsAndroid.RESULTS.GRANTED ) { console.log('All permissions granted'); // Your app can now use the requested permissions } else { console.log('Some permissions were denied'); // Handle the denial of permissions } } catch (err) { console.warn(err); } }
Feel free to adapt these code samples to your specific app’s needs.
Conclusion
Handling Android permissions in React Native is an essential aspect of building secure and user-friendly mobile applications. By understanding the types of permissions, permission groups, and best practices for requesting and handling permissions, you can ensure a smooth user experience while respecting user privacy. Remember to communicate the necessity of permissions, handle denials gracefully, and request multiple permissions when required. Incorporate these practices into your React Native app development workflow to create trustworthy and reliable applications.
Table of Contents
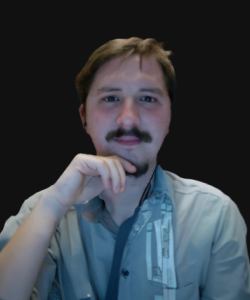
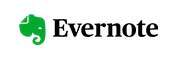