Ionic and Blockchain: Exploring Decentralized App Development
In today’s digital age, decentralized applications (DApps) are gaining significant traction due to their potential to revolutionize various industries. These applications leverage blockchain technology to enable secure, transparent, and tamper-proof transactions and interactions. If you’re a developer looking to dive into the world of DApp development, this blog is your guide. We will explore how to combine the robustness of Ionic with the power of blockchain to create decentralized apps that are both user-friendly and secure.
Table of Contents
1. Introduction to Decentralized Apps
1.1. Understanding Decentralization
Decentralization, at its core, refers to the distribution of power and control away from a central authority. In the context of applications, decentralization means that there is no single entity or central server that manages all the data and transactions. Instead, data and transactions are spread across a network of nodes, making it more resilient to censorship and single points of failure.
1.2. Why Choose Blockchain for DApps?
Blockchain technology is the backbone of decentralized applications. It is a distributed ledger that records all transactions across a network of computers, ensuring transparency, security, and immutability. Here are some compelling reasons to choose blockchain for DApp development:
- Security: Blockchain’s cryptographic techniques make it highly secure, minimizing the risk of data breaches and fraud.
- Transparency: All transactions on the blockchain are publicly recorded and can be audited, ensuring transparency and trust.
- Immutability: Once data is added to the blockchain, it cannot be altered or deleted, making it ideal for applications where data integrity is critical.
- Decentralization: Blockchain eliminates the need for a central authority, reducing the risk of censorship and single points of failure.
Now that you understand the basics of decentralization and blockchain, let’s dive into the practical aspects of creating a decentralized app.
2. Getting Started with Ionic
2.1. What is Ionic?
Ionic is an open-source framework for building cross-platform mobile and web applications. It offers a rich set of UI components and tools for creating interactive and visually appealing apps. One of the key advantages of Ionic is its ability to use web technologies such as HTML, CSS, and JavaScript to build apps that work on multiple platforms, including iOS, Android, and the web.
2.2. Setting Up Your Development Environment
Before you begin developing your decentralized app with Ionic, you need to set up your development environment. Here are the essential steps:
- Install Node.js: Ionic relies on Node.js, so make sure you have it installed on your machine. You can download it from the official website.
- Install Ionic CLI: The Ionic Command Line Interface (CLI) is used for creating and managing Ionic projects. You can install it globally using npm (Node Package Manager) by running the following command:
bash npm install -g @ionic/cli
- Create Your First Ionic App: Once the Ionic CLI is installed, you can create your first app by running the following command:
bash ionic start my-dapp tabs
This command creates a new Ionic app with a tabbed interface.
- Navigate to Your App Directory: Change your working directory to the newly created app directory:
bash cd my-dapp
- Run Your App: You can now run your app in a development server by running:
bash ionic serve
This will open your app in a web browser, allowing you to preview and test it.
With your Ionic development environment set up, you’re ready to start building your DApp.
3. Blockchain Basics
3.1. What is Blockchain?
Blockchain is a distributed ledger technology that consists of a chain of blocks, where each block contains a set of transactions. These transactions are cryptographically linked, forming a secure and immutable chain. Here are some key blockchain concepts:
- Decentralization: The blockchain network is distributed across multiple nodes (computers), making it decentralized and resistant to central control.
- Consensus Mechanisms: Blockchain uses consensus mechanisms like Proof of Work (PoW) or Proof of Stake (PoS) to validate and add new transactions to the blockchain.
- Smart Contracts: Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They automate and enforce agreements without the need for intermediaries.
3.2. Ethereum: A Leading Blockchain Platform for DApps
Ethereum is one of the most popular blockchain platforms for developing decentralized applications. It introduced the concept of smart contracts, which are Turing complete and allow developers to build complex decentralized applications. Ethereum’s native cryptocurrency, Ether (ETH), is used for transactions and as a fuel to execute smart contracts.
Now that you have a basic understanding of blockchain, let’s explore how to integrate it with Ionic to create a DApp.
4. Integrating Ionic and Blockchain
4.1. Building a DApp Frontend with Ionic
The frontend of your DApp is what users interact with, and Ionic provides a robust framework for creating visually appealing and user-friendly interfaces. You can design your DApp’s frontend using Ionic’s UI components and customize them to fit your application’s needs.
Here’s a simple example of an Ionic interface for a decentralized voting application:
html <!-- Ionic HTML template for a voting DApp --> <ion-header> <ion-toolbar> <ion-title> Decentralized Voting </ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-list> <ion-item> <ion-label>Proposal 1</ion-label> <ion-radio slot="end"></ion-radio> </ion-item> <ion-item> <ion-label>Proposal 2</ion-label> <ion-radio slot="end"></ion-radio> </ion-item> </ion-list> <ion-button expand="full" (click)="vote()">Vote</ion-button> </ion-content>
This example demonstrates the simplicity and flexibility of building a frontend for your DApp with Ionic’s components.
4.2. Connecting to the Ethereum Blockchain
To interact with the Ethereum blockchain from your Ionic app, you’ll need to use a library like Web3.js, which provides a JavaScript API for Ethereum. Here’s how you can set up Web3.js in your Ionic project:
Install Web3.js: Use npm to install Web3.js:
bash npm install web3
Import Web3.js: In your Ionic component or service, import Web3.js:
javascript import Web3 from 'web3';
Connect to Ethereum: Initialize Web3.js and connect it to the Ethereum network. You can do this in your component’s constructor or an initialization method:
javascript const web3 = new Web3(new Web3.providers.HttpProvider('https://infura.io/YOUR_INFURA_API_KEY'));
Replace ‘YOUR_INFURA_API_KEY’ with your Infura API key, which provides access to the Ethereum network.
Now, your Ionic app is connected to the Ethereum blockchain, and you can interact with smart contracts and perform transactions.
4.3. Smart Contracts: The Heart of DApps
Smart contracts are the backbone of decentralized applications. They define the rules and logic of the application and execute autonomously on the blockchain. To create and deploy smart contracts for your DApp, you can use languages like Solidity and development frameworks like Truffle.
Here’s a simplified example of a voting smart contract in Solidity:
solidity // Voting smart contract pragma solidity ^0.8.0; contract Voting { mapping (bytes32 => uint256) public votes; function vote(bytes32 proposal) public { votes[proposal] += 1; } }
This smart contract allows users to vote for different proposals, and the votes are stored on the Ethereum blockchain.
4.4. Handling Transactions and Data Storage
In your Ionic app, you can create functions to interact with your smart contract. For instance, to vote on a proposal, you can write a JavaScript function like this:
javascript async function vote(proposal) { const accounts = await web3.eth.getAccounts(); const contract = new web3.eth.Contract(contractAbi, contractAddress); await contract.methods.vote(web3.utils.asciiToHex(proposal)).send({ from: accounts[0] }); // Update the UI or perform other actions after the transaction is complete }
In this code, we first retrieve the user’s Ethereum account and then use the Web3.js library to interact with the smart contract by calling the vote function. The transaction is sent from the user’s account, and once confirmed, the UI can be updated accordingly.
5. Security and Considerations
5.1. Ensuring Smart Contract Security
Security is paramount in DApp development, especially when dealing with smart contracts. Here are some best practices to ensure the security of your DApp:
- Smart Contract Auditing: Have your smart contracts audited by security experts to identify and fix vulnerabilities.
- Use Secure Development Tools: Choose development tools and frameworks with a focus on security. Frameworks like Truffle offer security features and best practices.
- Test Thoroughly: Test your smart contracts extensively, including edge cases and potential attack vectors.
5.2. User Authentication and Data Privacy
User authentication and data privacy are crucial for DApps. Consider implementing secure authentication mechanisms, such as OAuth or decentralized identity solutions like SelfKey. Additionally, encrypt sensitive data to protect user privacy.
5.3. Testing and Auditing Your DApp
Thoroughly test your DApp by simulating various scenarios, including network congestion and attacks. Perform code audits and security assessments regularly to identify and mitigate vulnerabilities.
6. Deployment and Future Directions
6.1. Deploying Your DApp
Once your DApp is developed and thoroughly tested, it’s time to deploy it to a blockchain network. Ethereum is a popular choice for deployment due to its widespread adoption and support for smart contracts. You can use services like Infura or deploy your own Ethereum nodes for this purpose.
6.2. Scaling and Future Enhancements
As your DApp gains users and transactions, you may need to consider scaling solutions to handle increased traffic efficiently. Ethereum’s scalability solutions, such as layer 2 solutions like Optimistic Rollups, can help.
Additionally, consider user feedback and market trends to enhance your DApp continually. Explore new blockchain platforms and technologies that might offer improved features and scalability.
Conclusion
In this blog, we’ve explored the fusion of Ionic and blockchain technologies to create decentralized applications (DApps). Decentralization and blockchain provide security, transparency, and trust in the world of applications. By harnessing the power of Ionic for frontend development and Ethereum for smart contracts, you can create DApps that are not only user-friendly but also secure and reliable.
Dive into the world of DApp development, experiment with different smart contract ideas, and stay updated with the ever-evolving blockchain ecosystem. As you embark on your DApp development journey, remember that the decentralized future holds endless possibilities, and your innovation can be at the forefront of this revolution.
Table of Contents
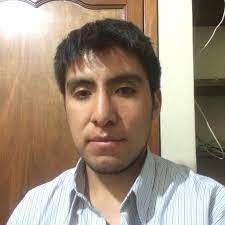
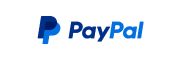