Creating Offline-First Apps with Ionic and PouchDB
In today’s interconnected world, app users expect smooth experiences, whether online or offline. However, network connectivity is not always reliable, especially in remote areas or during transit. To address this challenge, building offline-first apps has become essential. These apps allow users to continue using the app even when they are not connected to the internet, and any data changes made offline will be synchronized once the connection is restored.
In this blog, we will explore how to create offline-first apps using Ionic and PouchDB. Ionic is a popular open-source framework for building cross-platform mobile and web applications, while PouchDB is a NoSQL database that can work seamlessly both online and offline. Combining these technologies will enable us to develop resilient applications that provide a seamless user experience regardless of network availability.
1. Prerequisites
Before we dive into building the offline-first app, make sure you have the following installed:
1. Node.js and npm: Install the latest stable version of Node.js and npm from the official website (https://nodejs.org/).
2. Ionic CLI: Install Ionic globally using npm with the following command:
bash npm install -g @ionic/cli
3. Ionic Capacitor: Capacitor is used for native app integration. Install it globally with npm:
bash npm install -g @capacitor/cli
4. A Code Editor: Choose your preferred code editor. Popular options include Visual Studio Code, Sublime Text, or Atom.
Now that we have all the necessary prerequisites in place, let’s get started with the implementation.
2. Setting Up the Project
The first step is to create a new Ionic project and integrate Capacitor for native support. Open your terminal and run the following commands:
2.1 Create a new Ionic project
sql ionic start OfflineFirstApp blank --type=angular
This command will create a new Ionic project named “OfflineFirstApp” based on the “blank” template using Angular as the framework.
2.2 Navigate to the project folder
bash cd OfflineFirstApp
2.3 Add the platform
csharp ionic capacitor add android ionic capacitor add ios
These commands will add the Android and iOS platforms to the project, allowing us to build and deploy the app on mobile devices.
3. Installing PouchDB
Now that we have our basic project structure in place, let’s integrate PouchDB into the Ionic app.
3.1 Install PouchDB and its required adapter
bash npm install pouchdb @ionic/pouchdb-adapter
3.2 Configure PouchDB in the app
Open the “src/app/app.module.ts” file and import PouchDB and the adapter:
typescript import PouchDB from 'pouchdb'; import { IonicPouchDBAdapter } from '@ionic/pouchdb-adapter';
3.3 Initialize PouchDB
Still in the “app.module.ts” file, add the following code to initialize PouchDB:
typescript const localDB = new PouchDB('my_local_db', { adapter: IonicPouchDBAdapter });
In this example, we’ve named the database ‘my_local_db,’ but you can choose any name you like. The IonicPouchDBAdapter ensures that PouchDB works correctly within the Ionic environment.
4. Creating Offline Data Handling
With PouchDB integrated into our Ionic app, we can now proceed to create offline data handling. This involves storing data locally when the app is offline and synchronizing it with a remote database when the app regains internet connectivity.
4.1 Create a Data Service
In order to manage data interactions with PouchDB, let’s create a data service. Run the following command to generate the service:
bash ionic generate service services/data
This will generate a new service file named “data.service.ts” inside the “src/app/services” folder.
4.2 Implement Data Handling
Open the “data.service.ts” file and import PouchDB:
typescript import PouchDB from 'pouchdb';
Next, define the PouchDB instance and implement functions for data handling. For example, let’s create functions to add and retrieve data:
typescript @Injectable({ providedIn: 'root' }) export class DataService { private localDB: any; constructor() { this.localDB = new PouchDB('my_local_db', { adapter: IonicPouchDBAdapter }); } addData(data: any): Promise<any> { return this.localDB.post(data); } getAllData(): Promise<any> { return this.localDB.allDocs({ include_docs: true }); } }
The addData() function allows us to store data in the local PouchDB database, while the getAllData() function retrieves all the stored data.
5. Synchronizing Data
One of the essential features of an offline-first app is the ability to synchronize data with a remote database when the internet is available. For this, we can use PouchDB’s replication feature.
5.1 Implement Data Replication
In the “data.service.ts” file, let’s create a function to handle data replication:
typescript syncData(remoteURL: string): any { const remoteDB = new PouchDB(remoteURL); return this.localDB.replicate.to(remoteDB).on('complete', () => { console.log('Data replication to remote database successful.'); }).on('error', (err: any) => { console.error('Data replication error:', err); }); }
The syncData() function replicates the data from the local PouchDB database to the specified remote database URL. You can use any compatible database server like CouchDB or Cloudant for the remote database.
5.2 Trigger Data Synchronization
To synchronize data, call the syncData() function whenever the app regains internet connectivity. This can be achieved by adding event listeners for network status changes in the Ionic app.
Open the “app.component.ts” file and import the Network plugin:
typescript import { Network } from '@capacitor/network';
Then, add the following code to trigger data synchronization:
typescript initializeApp() { // Other code... Network.addListener('networkStatusChange', (status) => { if (status.connected) { this.dataService.syncData('https://example.com/my_remote_db').then(() => { // Data synchronized successfully }).catch((err) => { // Handle sync error }); } }); }
The above code listens for network status changes and triggers data synchronization when the device is connected to the internet.
6. Offline Data Access and Real-time Updates
Now that we have set up data synchronization, our offline-first app can seamlessly manage data both online and offline. The data is stored locally using PouchDB, and changes are automatically synchronized with the remote database when the app is online.
Additionally, we can utilize PouchDB’s real-time updates feature to keep the app data in sync with any changes made on the remote database.
6.1 Implement Real-time Updates
In the “data.service.ts” file, add the following code to enable real-time updates:
typescript enableRealTimeUpdates(remoteURL: string): any { const remoteDB = new PouchDB(remoteURL); this.localDB.sync(remoteDB, { live: true, retry: true }).on('change', (change) => { console.log('Real-time update:', change); }).on('error', (err) => { console.error('Real-time update error:', err); }); }
The enableRealTimeUpdates() function enables real-time synchronization between the local and remote databases. Any changes made in one database will be immediately reflected in the other.
6.2 Enable Real-time Updates
Finally, in the “app.component.ts” file, call the enableRealTimeUpdates() function to enable real-time updates:
typescript initializeApp() { // Other code... this.dataService.enableRealTimeUpdates('https://example.com/my_remote_db'); }
Now, your offline-first app will receive real-time updates from the remote database when connected to the internet.
Conclusion
In this blog, we explored the process of creating offline-first apps using Ionic and PouchDB. We learned how to set up the project, install PouchDB, and handle offline data using Ionic’s Capacitor. With PouchDB’s replication and real-time updates features, our app can maintain data integrity and provide a seamless user experience, even when the internet connection is unreliable.
Building offline-first apps is crucial for meeting user expectations in today’s digital landscape. Users will appreciate the uninterrupted experience they get with your app, leading to higher engagement and customer satisfaction.
Start building your offline-first app with Ionic and PouchDB today, and create an exceptional user experience that stands out in the crowded app market. Happy coding!
Table of Contents
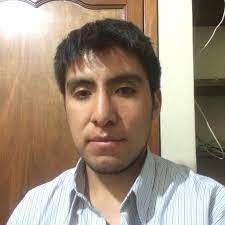
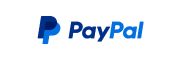