Ionic for E-commerce: Building Mobile Shopping Experiences
In the rapidly evolving digital landscape, e-commerce has become a dominant force in the global economy. As consumers increasingly turn to their mobile devices for shopping, providing an exceptional mobile shopping experience has become crucial for the success of any online store. Ionic, a popular open-source cross-platform framework, empowers developers to build stunning and feature-rich e-commerce mobile apps that work seamlessly across various platforms.
In this blog, we will explore how Ionic can revolutionize your e-commerce business by creating captivating mobile shopping experiences. From responsive UI design to integrating with backend systems, we’ll cover the essential aspects of building an efficient and user-friendly e-commerce app using Ionic.
1. Understanding Ionic and its Advantages for E-commerce
Before diving into the specifics, let’s briefly understand what Ionic is and why it’s a great choice for e-commerce app development.
1.1 What is Ionic?
Ionic is an open-source UI toolkit for building cross-platform mobile and web applications using web technologies such as HTML, CSS, and JavaScript. It leverages Angular, a widely-used JavaScript framework, to build interactive and performant mobile applications.
1.2 Advantages of Ionic for E-commerce
- Cross-platform compatibility: Ionic allows developers to build a single codebase that can run seamlessly on both iOS and Android devices, saving time and effort in development and maintenance.
- Native-like experience: Ionic’s UI components are designed to mimic native elements, providing users with a familiar and intuitive shopping experience.
- Fast development: Ionic’s pre-designed components and tools streamline development, enabling rapid prototyping and quick iterations.
- Plugin ecosystem: Ionic boasts an extensive collection of plugins that facilitate integration with native features like geolocation, camera, and push notifications.
2. Designing a Responsive and User-Friendly E-commerce UI
A visually appealing and user-friendly interface is the cornerstone of any successful e-commerce app. Ionic offers an array of pre-built UI components and themes that can be customized to suit your brand’s identity.
2.1 Navigation and Layout
An effective e-commerce app should have a clear and straightforward navigation structure. Ionic’s navigation components like tabs, side menus, and navigation drawers make it easy to implement a user-friendly navigation system.
Code Sample: Implementing Tabs in Ionic
html <ion-tabs> <ion-tab-bar slot="bottom"> <ion-tab-button tab="home"> <ion-icon name="home"></ion-icon> <ion-label>Home</ion-label> </ion-tab-button> <ion-tab-button tab="categories"> <ion-icon name="list"></ion-icon> <ion-label>Categories</ion-label> </ion-tab-button> <ion-tab-button tab="cart"> <ion-icon name="cart"></ion-icon> <ion-label>Cart</ion-label> </ion-tab-button> <ion-tab-button tab="profile"> <ion-icon name="person"></ion-icon> <ion-label>Profile</ion-label> </ion-tab-button> </ion-tab-bar> </ion-tabs>
2.2 Product Listing and Detail Pages
Presenting products in an appealing manner is crucial for driving sales. Ionic allows you to create visually appealing product listing and detail pages with smooth transitions and interactive elements.
Code Sample: Creating a Product Listing Card
html <ion-card *ngFor="let product of products"> <ion-img [src]="product.image"></ion-img> <ion-card-header> <ion-card-subtitle>{{ product.category }}</ion-card-subtitle> <ion-card-title>{{ product.name }}</ion-card-title> </ion-card-header> <ion-card-content> <p>{{ product.description }}</p> <p>Price: ${{ product.price }}</p> <ion-button expand="full" (click)="addToCart(product)">Add to Cart</ion-button> </ion-card-content> </ion-card>
2.3 Personalization and Recommendations
Leveraging user data to provide personalized product recommendations can significantly enhance the user experience. Ionic enables seamless integration with backend services to analyze user behavior and display personalized content.
3. Integrating Backend Services for E-commerce Functionality
To create a fully functional e-commerce app, seamless integration with backend services is crucial. Ionic supports various backend solutions, making it flexible to work with different e-commerce platforms and databases.
3.1 RESTful API Integration
Most e-commerce platforms expose RESTful APIs that allow access to their data and functionality. Ionic’s built-in HTTP client makes it easy to communicate with these APIs and retrieve product information, user data, and more.
Code Sample: Retrieving Product Data from an API
typescript import { HttpClient } from '@angular/common/http'; // ... constructor(private http: HttpClient) {} getProducts(): Observable<Product[]> { return this.http.get<Product[]>('https://api.example.com/products'); }
3.2 Real-time Data with WebSockets
For features like real-time notifications and chat support, Ionic can be integrated with WebSockets, enabling bidirectional communication between the client and server.
3.3 Authentication and Security
Secure user authentication is vital for e-commerce apps to protect sensitive user data. Ionic offers various authentication plugins that support popular authentication methods like OAuth and JWT (JSON Web Tokens).
4. Optimizing Performance and User Experience
Performance is a critical factor in e-commerce app success. Slow-loading pages and unresponsive UI can lead to user frustration and abandoned carts. Ionic provides several optimization techniques to enhance app performance.
4.1 Lazy Loading
Lazy loading allows the app to load modules only when needed, reducing the initial loading time and optimizing the overall app performance.
Code Sample: Implementing Lazy Loading in Ionic
typescript const routes: Routes = [ { path: 'home', loadChildren: () => import('./home/home.module').then(m => m.HomeModule) }, { path: 'categories', loadChildren: () => import('./categories/categories.module').then(m => m.CategoriesModule) }, { path: 'cart', loadChildren: () => import('./cart/cart.module').then(m => m.CartModule) }, { path: 'profile', loadChildren: () => import('./profile/profile.module').then(m => m.ProfileModule) }, ];
4.2 Image Optimization
Compressing and caching images can significantly reduce the app’s size and load times, ensuring a smoother shopping experience for users.
5. Implementing Payments and Checkout
Seamless payment processing and a smooth checkout experience are crucial to converting potential customers into loyal buyers. Ionic offers various payment integration options to facilitate secure transactions.
5.1 Integrating Payment Gateways
Ionic supports plugins for integrating popular payment gateways like PayPal, Stripe, and Braintree. These plugins handle secure payment processing, providing a seamless checkout experience for users.
Code Sample: Integrating Stripe for Payment Processing
typescript import { Stripe } from '@ionic-native/stripe/ngx'; // ... constructor(private stripe: Stripe) {} async processPayment(cardDetails: any, amount: number) { try { await this.stripe.setPublishableKey('YOUR_STRIPE_PUBLISHABLE_KEY'); const token = await this.stripe.createCardToken(cardDetails); // Send the token to your server for payment processing } catch (error) { console.error('Error processing payment:', error); } }
6. Implementing Push Notifications
Keeping users informed about new products, discounts, and order updates can drive engagement and increase sales. Ionic’s push notification integration simplifies this process.
Code Sample: Implementing Push Notifications with Firebase Cloud Messaging (FCM)
typescript import { FCM } from '@ionic-native/fcm/ngx'; // ... constructor(private fcm: FCM) {} initializePushNotifications() { this.fcm.subscribeToTopic('promotions'); this.fcm.onNotification().subscribe(data => { if (data.wasTapped) { // Handle notification when the app is in the background or closed } else { // Handle notification when the app is in the foreground } }); }
Conclusion
In the competitive world of e-commerce, providing captivating mobile shopping experiences is no longer optional; it’s a necessity. Ionic’s cross-platform capabilities, powerful UI toolkit, and seamless integration with backend services make it a top choice for building e-commerce mobile apps. With this technology at your disposal, you can create stunning, user-friendly, and high-performance mobile shopping experiences that delight your customers and drive your business forward. So, take advantage of Ionic’s potential and unlock new opportunities for your e-commerce venture!
Table of Contents
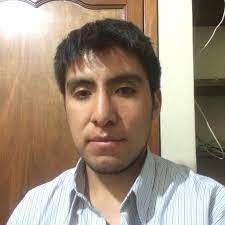
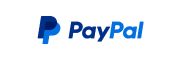