Ionic and Firebase Authentication: Securing Your App
In today’s digital landscape, security is paramount when developing mobile applications. As an Ionic developer, it’s crucial to ensure that your app’s user data remains protected from unauthorized access and potential breaches. Firebase Authentication offers a reliable solution to bolster the security of your Ionic app effortlessly. This blog post will guide you through the process of integrating Firebase Authentication into your Ionic app, allowing you to secure user data and privacy effectively.
1. Understanding Firebase Authentication:
Before diving into the implementation, let’s briefly understand what Firebase Authentication is and how it works. Firebase Authentication is a service provided by Google’s Firebase platform that handles user authentication, making it easy for developers to add authentication features to their apps. It supports various authentication methods, including email and password, phone number, Google, Facebook, Twitter, and more. Firebase Authentication takes care of authentication, identity verification, and data storage, freeing you from implementing complex security mechanisms manually.
2. Setting Up Firebase Project:
2.1 Create a Firebase Project:
First, visit the Firebase Console (console.firebase.google.com) and create a new project. Follow the on-screen instructions to set up your project.
2.2 Add Your App to the Firebase Project:
After creating the project, click on “Add app” and select “Web” to get your Firebase configuration details (API keys, auth domain, etc.). These credentials will be used later in your Ionic app.
3. Installing Dependencies:
To get started with integrating Firebase Authentication into your Ionic app, you need to install the necessary packages. Open your Ionic project’s terminal and enter the following commands:
bash ionic cordova plugin add cordova-plugin-firebase npm install @ionic-native/firebase @angular/fire firebase --save
4. Configuring Firebase in Your Ionic App:
4.1 Import the Required Modules:
In your app.module.ts file, import the Firebase and AngularFire modules.
typescript import { AngularFireModule } from '@angular/fire'; import { environment } from '../environments/environment'; import { Firebase } from '@ionic-native/firebase/ngx'; @NgModule({ declarations: [...], imports: [ AngularFireModule.initializeApp(environment.firebaseConfig), // other imports... ], providers: [ Firebase, // other providers... ], bootstrap: [...], }) export class AppModule {}
4.2 Configure Firebase Environment:
Create an environment.ts file in the src/environments directory and add your Firebase configuration details to it.
typescript export const environment = { production: false, firebaseConfig: { apiKey: '<YOUR_API_KEY>', authDomain: '<YOUR_AUTH_DOMAIN>', projectId: '<YOUR_PROJECT_ID>', storageBucket: '<YOUR_STORAGE_BUCKET>', messagingSenderId: '<YOUR_MESSAGING_SENDER_ID>', appId: '<YOUR_APP_ID>', measurementId: '<YOUR_MEASUREMENT_ID>', }, };
Remember to replace the placeholders with the actual values from your Firebase project.
5. Implementing Firebase Authentication:
Now that you have set up Firebase in your Ionic app, it’s time to implement the authentication features.
5.1 User Sign-Up and Sign-In:
To allow users to sign up and sign in, create a registration and login interface in your app. Then, use the Firebase Authentication API to handle the authentication process.
typescript // Sign Up async signUp(email: string, password: string): Promise<void> { try { await firebase.auth().createUserWithEmailAndPassword(email, password); // Additional user data handling (if required)... } catch (error) { // Handle sign-up errors... } } // Sign In async signIn(email: string, password: string): Promise<void> { try { await firebase.auth().signInWithEmailAndPassword(email, password); // Proceed with authenticated user... } catch (error) { // Handle sign-in errors... } }
5.2 Implement Social Logins:
To offer social login options to your users, such as Google or Facebook login, follow these steps:
1. Enable Social Providers in Firebase Console:
Go to the Firebase Console, navigate to “Authentication” > “Sign-in method,” and enable the desired social providers.
2. Add Capacitor Plugins (for native app):
For Google login:
bash npm install @codetrix-studio/capacitor-google-auth npx cap sync
For Facebook login:
bash npm install cordova-plugin-facebook4 @ionic-native/facebook npx cap sync
3. Implement Social Login in Your App:
typescript // For Google Login async googleSignIn(): Promise<void> { try { const googleUser = await this.firebaseNative.signInWithGoogle(); // Additional user data handling (if required)... } catch (error) { // Handle Google sign-in errors... } } // For Facebook Login async facebookSignIn(): Promise<void> { try { const response = await this.facebook.login(['email']); const facebookCredential = firebase.auth.FacebookAuthProvider.credential(response.authResponse.accessToken); const facebookUser = await firebase.auth().signInWithCredential(facebookCredential); // Additional user data handling (if required)... } catch (error) { // Handle Facebook sign-in errors... } }
6. Handling Authentication State:
To manage the authentication state in your app, you can use Firebase’s authentication observables. These observables provide real-time updates on the user’s authentication status, making it easier to display appropriate UI elements accordingly.
typescript import { AngularFireAuth } from '@angular/fire/auth'; import { auth } from 'firebase/app'; @Injectable({ providedIn: 'root', }) export class AuthService { user$: Observable<firebase.User | null>; constructor(private afAuth: AngularFireAuth) { this.user$ = this.afAuth.authState; } // Logout async signOut(): Promise<void> { try { await this.afAuth.signOut(); } catch (error) { // Handle sign-out errors... } } }
7. Securing API Endpoints:
While Firebase Authentication handles user authentication, you may still have API endpoints that require additional security measures. For instance, you might want to prevent unauthorized access to certain resources. Firebase provides an easy way to secure these endpoints using Firebase’s Security Rules.
Conclusion
With Firebase Authentication and Ionic, you can build secure apps with ease. By following the steps outlined in this blog, you have equipped yourself with the knowledge to safeguard your users’ data and privacy effectively. Remember that security is an ongoing process, so it’s essential to stay updated with the latest security practices and regularly review your app’s security measures. Now, go ahead and create an outstanding and secure app experience for your users! Happy coding!
Table of Contents
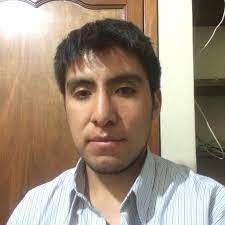
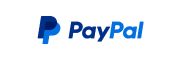