Ionic and Location-Based Services: Leveraging Geofencing
In today’s mobile-driven world, location-based services have become integral to app development. Whether it’s enhancing user experiences or providing personalized content, knowing a user’s location can be a game-changer. One of the most potent tools for harnessing location data is geofencing. This blog post will explore the world of geofencing in the context of Ionic, a popular framework for building cross-platform mobile apps. We’ll delve into the concept of geofencing, its applications, and provide you with practical code samples to get started.
Table of Contents
1. What is Geofencing?
1.1. Understanding the Basics
Geofencing, at its core, is a virtual perimeter you define around a real-world geographic area. It allows you to trigger actions when a user’s device enters or exits this defined boundary. These actions can range from sending notifications to updating data or initiating specific functions within your mobile app.
Think of geofencing as an invisible fence around a location. When a device equipped with your app crosses this fence’s boundary, it triggers predefined events. This capability opens up numerous possibilities for location-based services and personalized user experiences.
1.2. Applications of Geofencing
1.2.1. Retail and Marketing
Retailers often use geofencing to target nearby customers with special offers or promotions. For example, when a potential customer walks near a store, the retailer’s app can send a notification about ongoing sales or discounts, enticing them to enter the store.
1.2.2. Navigation
Navigation apps use geofencing to provide real-time alerts for upcoming turns, traffic congestion, or points of interest. This technology enhances the overall navigation experience and ensures users stay informed while on the road.
1.2.3. Social Networking
Social networking apps leverage geofencing to facilitate location-based check-ins and recommendations. Users can discover nearby friends, events, or places of interest, fostering community engagement.
1.2.4. Safety and Security
Geofencing can play a crucial role in enhancing safety and security. For instance, parents can set up geofences around their children’s schools, and if the child leaves the designated area, the parents receive an alert. This application is also used in asset tracking and anti-theft systems.
2. Implementing Geofencing in Ionic
Now that we understand the significance of geofencing let’s explore how to implement it in Ionic. We’ll guide you through the process step by step, including code snippets for clarity.
2.1. Prerequisites
Before diving into geofencing, ensure you have the following prerequisites:
- Ionic Installed: Make sure you have Ionic installed on your development machine. If not, you can install it using npm (Node Package Manager) with the following command:
bash npm install -g @ionic/cli
- A Code Editor: Choose a code editor of your preference. Popular choices include Visual Studio Code, Sublime Text, or Atom.
2.2. Setting Up a New Ionic Project
Let’s start by creating a new Ionic project. Open your terminal and run the following commands:
bash ionic start GeofenceApp blank cd GeofenceApp
This creates a new Ionic project named “GeofenceApp.”
2.3. Installing Required Plugins
To work with geofencing in Ionic, you’ll need to install some plugins that provide geolocation and geofencing functionality. The most commonly used plugin for this purpose is the “Cordova Geolocation Plugin.” Install it by running the following command:
bash ionic cordova plugin add cordova-plugin-geolocation npm install @ionic-native/geolocation
This plugin enables you to access the device’s geolocation capabilities.
Next, you’ll need a geofencing plugin. A popular choice is the “Cordova Background Geolocation Plugin.” Install it with the following command:
bash ionic cordova plugin add cordova-plugin-background-geolocation npm install @ionic-native/background-geolocation
This plugin is essential for running geofencing operations in the background.
3. Implementing Geofencing
With the necessary plugins in place, it’s time to implement geofencing. We’ll outline the steps for creating a simple geofencing scenario: sending a notification when the user enters a predefined geofenced area.
Step 1: Set Up Permissions
Before accessing the device’s geolocation, you need to request permission from the user. Open your Ionic project’s src/app/app.component.ts file and add the following code within the platform.ready() block:
typescript import { Geolocation } from '@ionic-native/geolocation/ngx'; constructor(private platform: Platform, private geolocation: Geolocation) { this.platform.ready().then(() => { // Request location permission this.geolocation .requestPermission() .then(() => { console.log('Location permission granted'); }) .catch((error) => { console.error('Error requesting location permission', error); }); }); }
This code requests location permission when the app is ready.
Step 2: Define Geofence Parameters
Next, define the geofence parameters. Create a new function in your app.component.ts file to set up the geofence details:
typescript import { Geofence } from '@ionic-native/geofence/ngx'; constructor(private geofence: Geofence) {} setupGeofence() { const fence = { id: 'myGeofenceID', latitude: 37.7749, // Replace with your desired latitude longitude: -122.4194, // Replace with your desired longitude radius: 100, // Geofence radius in meters transitionType: 3, // Transition type: 3 (Enter) }; this.geofence .addOrUpdate(fence) .then( () => console.log('Geofence added'), (error) => console.error('Error adding geofence', error) ); }
In this code, we define a geofence with an ID, latitude, longitude, radius, and transition type. The transition type “3” corresponds to entering the geofenced area.
Step 3: Listen for Geofence Events
Now, set up an event listener to respond when the user enters the geofenced area:
typescript import { Events } from '@ionic/angular'; constructor(private events: Events) {} ngOnInit() { this.events.subscribe('geofence:enter', () => { // Handle geofence entry console.log('Entered geofenced area'); // Trigger your desired action, such as sending a notification }); }
This code listens for the ‘geofence:enter’ event and triggers the specified action when the user enters the geofenced area.
Step 4: Trigger Geofence Setup
Finally, call the setupGeofence() function in your ngOnInit() method to set up the geofence when the app initializes:
typescript ngOnInit() { this.setupGeofence(); this.events.subscribe('geofence:enter', () => { // Handle geofence entry console.log('Entered geofenced area'); // Trigger your desired action, such as sending a notification }); }
With these steps completed, your Ionic app is now equipped with geofencing capabilities. When a user enters the defined geofenced area, the app will trigger the specified action, which can include sending notifications, updating data, or performing any custom function you require.
Conclusion
Geofencing is a powerful tool in the world of mobile app development, enabling you to create location-aware and context-aware applications. In this blog post, we’ve explored the basics of geofencing, its applications, and how to implement it in an Ionic app. With the right plugins and code, you can provide users with personalized experiences based on their location, whether it’s for retail, navigation, social networking, or safety and security.
Remember that geofencing should be used responsibly and with respect for user privacy. Always request and handle location permissions appropriately, and provide users with clear information on how their location data will be used. By doing so, you can harness the power of geofencing to enhance your Ionic mobile apps while maintaining trust with your users.
Table of Contents
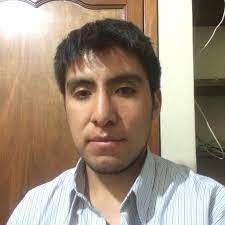
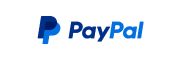