Ionic and Machine Vision: Integrating Image Recognition
In today’s fast-paced digital world, mobile applications are evolving to offer increasingly sophisticated features. One of the most exciting advancements is the integration of machine vision and image recognition into mobile apps. This fusion of technology opens up a world of possibilities for enhancing user experiences and providing innovative solutions.
Table of Contents
In this blog, we will explore the powerful combination of Ionic, a popular framework for building cross-platform mobile apps, and machine vision for image recognition. We’ll delve into the key concepts, tools, and techniques required to seamlessly integrate image recognition into your Ionic apps, complete with practical code examples. So, let’s embark on this exciting journey of merging mobile app development with machine vision.
1. Understanding Image Recognition
1.1. What is Image Recognition?
Image recognition, a subset of computer vision, is the process of identifying and classifying objects or patterns in images or videos. It simulates human visual perception by analyzing digital images and making sense of them using algorithms. Image recognition has a wide range of applications, from automating tasks to enhancing user experiences.
1.2. Use Cases for Image Recognition
Image recognition technology has found its way into various industries and applications, including:
- Healthcare: Identifying diseases from medical images.
- Automotive: Autonomous vehicle navigation and driver assistance systems.
- Retail: Inventory management and cashier-less shopping experiences.
- Security: Facial recognition for access control and surveillance.
- Entertainment: Augmented reality (AR) gaming and interactive experiences.
- E-commerce: Product search using images.
- Manufacturing: Quality control and defect detection.
2. Introduction to Ionic Framework
2.1. What is Ionic?
Ionic is an open-source framework for building cross-platform mobile applications using web technologies like HTML, CSS, and JavaScript. It provides a set of UI components and tools for developing native-like mobile apps for iOS, Android, and the web, all from a single codebase. Ionic is known for its simplicity, speed, and ease of use.
2.2. Advantages of Using Ionic
Why choose Ionic for mobile app development? Here are some compelling advantages:
- Cross-Platform: Write once, run anywhere for multiple platforms.
- UI Components: Pre-designed UI elements for faster development.
- Performance: High-performance, native-like app experiences.
- Plugins: Access to a vast ecosystem of plugins for extended functionality.
- Community: A large and active community for support and resources.
2.3. Setting Up Your Ionic Development Environment
Before we dive into integrating image recognition, you need to set up your Ionic development environment. Follow these steps:
- Install Node.js: Ionic relies on Node.js, so make sure you have it installed. You can download it from the official website.
- Install Ionic CLI: Open your terminal and run the following command to install the Ionic CLI globally:
bash npm install -g @ionic/cli
- Create a New Ionic Project: Once the CLI is installed, create a new Ionic project using the following command:
arduino ionic start my-image-recognition-app blank
- Navigate to Your Project: Change your current directory to the newly created project:
arduino cd my-image-recognition-app
- Run the App: Start a local development server and open your app in a web browser with the following command:
ionic serve
Your Ionic development environment is now set up and ready for image recognition integration.
3. Leveraging Machine Vision for Image Recognition
3.1. Machine Learning vs. Machine Vision
Machine learning and machine vision are closely related fields, but they have distinct focuses. Machine learning involves training algorithms to learn patterns and make predictions, while machine vision specifically deals with the interpretation of visual data. Image recognition is a subset of machine vision that employs machine learning techniques.
3.2. Image Recognition Tools and Libraries
To integrate image recognition into your Ionic app, you’ll need the right tools and libraries. Some popular choices include:
- TensorFlow: An open-source machine learning framework with pre-trained models for image recognition.
- OpenCV: A computer vision library with a wide range of image processing and recognition functions.
- Clarifai: A cloud-based image and video recognition platform with an easy-to-use API.
- Custom Models: You can also train custom image recognition models using tools like TensorFlow, PyTorch, or Keras.
3.3. Key Concepts in Machine Vision
Before you start coding, it’s essential to understand some key concepts in machine vision:
- Image Preprocessing: Techniques like resizing, normalization, and noise reduction to prepare images for recognition.
- Feature Extraction: Identifying distinctive features in an image, such as edges or textures.
- Machine Learning Models: Algorithms like convolutional neural networks (CNNs) for image classification.
- Training and Inference: The process of training a model with labeled data and using it to make predictions.
4. Integrating Image Recognition into Ionic Apps
4.1. Preparing Your Ionic Project
To integrate image recognition into your Ionic app, you’ll need to structure your project and add the necessary dependencies. Here’s a step-by-step guide:
- Project Structure: Organize your project folders to keep image recognition-related code organized.
css src/ ??? app/ ??? assets/ ? ??? images/ ??? pages/ ? ??? recognition/ ? ? ??? recognition.page.html ? ? ??? recognition.page.scss ? ? ??? recognition.page.ts ? ??? ... ??? …
- Install Dependencies: Install the libraries and plugins you’ll need for image recognition. For example, to use TensorFlow.js, run:
bash npm install @tensorflow/tfjs @tensorflow-models/coco-ssd
- Initialize TensorFlow: In your app’s entry point (e.g., app.component.ts), initialize TensorFlow.js:
javascript import * as tf from '@tensorflow/tfjs'; async function initialize() { await tf.ready(); // Your code here } initialize();
4.2. Building a Simple Image Recognition App
Let’s create a basic Ionic app that can recognize objects in images using TensorFlow.js. We’ll start with a simple image upload interface and then perform image recognition on the uploaded image.
- Create the UI: In recognition.page.html, add the following code to create a basic UI:
html <ion-header> <ion-toolbar> <ion-title> Image Recognition </ion-title> </ion-toolbar> </ion-header> <ion-content> <input type="file" (change)="uploadImage($event)" accept="image/*" /> <ion-img [src]="imageUrl" *ngIf="imageUrl"></ion-img> <ion-label *ngIf="prediction">{{ prediction }}</ion-label> </ion-content>
- Handle Image Upload: In recognition.page.ts, implement the uploadImage function to handle image uploads:
javascript async uploadImage(event) { const file = event.target.files[0]; if (file) { this.imageUrl = URL.createObjectURL(file); const model = await cocoSsd.load(); const imageElement = document.createElement('img'); imageElement.src = this.imageUrl; const predictions = await model.detect(imageElement); this.prediction = predictions[0]?.class; } }
This simple app allows users to upload an image, and it uses the COCO-SSD model from TensorFlow.js to perform image recognition. The recognized object’s class is displayed on the screen.
In the next part of this blog, we will explore advanced techniques and best practices for image recognition integration in Ionic apps, including handling image data, training custom models, and real-time image recognition.
5. Advanced Techniques and Best Practices
5.1. Handling Image Data
Handling image data efficiently is crucial for image recognition. Consider the following best practices:
- Image Compression: Compress images to reduce storage and bandwidth usage.
- Caching: Cache preprocessed images to improve recognition speed.
- Streaming: Implement image streaming for real-time applications.
- Data Augmentation: Augment training data with variations of images for better model performance.
5.2. Training Custom Models
While pre-trained models like COCO-SSD are powerful, you may need custom models for specific tasks. Here’s an overview of the process:
- Data Collection: Gather and label a dataset for your task.
- Model Selection: Choose an appropriate architecture (e.g., CNN) for your problem.
- Training: Train the model on your dataset using a deep learning framework.
- Integration: Integrate the custom model into your Ionic app.
5.3. Real-time Image Recognition
Real-time image recognition adds a layer of complexity to your app. Consider the following aspects:
- Camera Access: Use Ionic’s camera plugin or native camera APIs.
- Frame Rate: Optimize frame processing to maintain a smooth experience.
- Concurrency: Implement asynchronous processing to avoid blocking the UI thread.
- Feedback: Provide visual or audio feedback for recognition results.
6. Enhancing User Experiences with Image Recognition
6.1. Augmented Reality (AR) Applications
AR apps overlay digital information on the real world. Examples include gaming, navigation, and interactive experiences.
- Tools: Utilize AR frameworks like AR.js or ARKit for iOS.
- Marker Tracking: Recognize markers or objects in the physical world.
- Gesture Recognition: Recognize user gestures for interaction.
6.2. Image-Based Authentication
Enhance security with image-based authentication:
- Face Recognition: Authenticate users through facial recognition.
- Fingerprint Scanning: Use fingerprint recognition for secure access.
- Behavioral Biometrics: Analyze user behavior for continuous authentication.
6.3. E-commerce and Product Recognition
E-commerce apps can benefit from image recognition for product search and recommendation:
- Product Search: Allow users to search for products by taking pictures.
- Barcode Scanning: Scan barcodes for product details and price comparison.
- Visual Search: Enable visual search within images or photos.
7. Challenges and Considerations
While integrating image recognition into your Ionic app can bring many benefits, it’s essential to address certain challenges and considerations.
- Performance Optimization
- Latency: Minimize recognition latency for a smooth user experience.
- Resource Usage: Optimize memory and CPU usage for mobile devices.
- Edge Cases: Handle challenging conditions like low light or occlusions.
- Privacy and Security
- Data Privacy: Ensure user data and images are handled securely.
- Model Security: Protect your recognition models from tampering.
- Legal Compliance: Be aware of data privacy regulations and compliance requirements.
7.1. Cross-Platform Compatibility
Ensure that your image recognition solution works consistently across iOS, Android, and web platforms. Test thoroughly on different devices and browsers to identify and address platform-specific issues.
Conclusion
In this blog, we’ve explored the exciting world of integrating image recognition into Ionic mobile apps. We began by understanding the fundamentals of image recognition, delved into the Ionic framework, and discussed the tools and libraries for machine vision. We also built a simple image recognition app and explored advanced techniques and best practices.
With image recognition, you can enhance user experiences, create innovative applications, and solve real-world problems. As you embark on your journey to integrate image recognition into your Ionic apps, keep in mind the challenges, considerations, and opportunities that this technology offers. Whether you’re developing AR applications, implementing image-based authentication, or revolutionizing e-commerce, image recognition can be a game-changer in the world of mobile app development.
So, embrace the power of image recognition, and unlock a new dimension of possibilities in your Ionic mobile apps!
Table of Contents
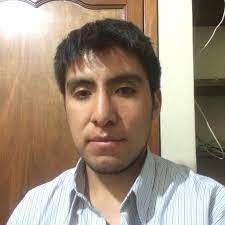
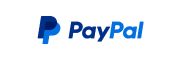