Ionic and User Authentication: Securing App Access
In today’s digital age, user data security is paramount. Whether you’re developing a social networking app, an e-commerce platform, or a simple to-do list application, ensuring the protection of user information is non-negotiable. This is where user authentication comes into play. In this comprehensive guide, we’ll delve into the world of Ionic and user authentication, equipping you with the knowledge and tools needed to secure your app and provide your users with a safe and seamless experience.
Table of Contents
1. Why User Authentication Matters
1.1. Protecting User Data
User authentication is the first line of defense against unauthorized access to sensitive information. It ensures that only authorized individuals can access certain features or data within your app. Without proper authentication, user data can be compromised, leading to privacy breaches and potential legal issues.
1.2. Personalized User Experience
Authentication also plays a pivotal role in tailoring the user experience. By identifying users and their preferences, you can provide a personalized journey through your app. This not only enhances user satisfaction but also encourages continued engagement.
1.3. Building Trust
Trust is a vital element in the success of any app. When users know their data is secure, they’re more likely to engage with your app, share their information, and recommend it to others. A robust authentication system is the foundation of that trust.
2. Getting Started with Ionic
2.1. Setting Up Your Development Environment
Before we dive into authentication, let’s set up our development environment. Ensure you have Node.js and npm (Node Package Manager) installed on your system. You’ll also need Ionic CLI. You can install it globally using the following command:
shell npm install -g @ionic/cli
2.2. Creating an Ionic App
Now that your environment is set up, create a new Ionic app. Use the following command to get started:
shell ionic start my-auth-app blank
Replace my-auth-app with your desired app name. This command initializes a new Ionic app with a blank template.
3. User Authentication in Ionic
3.1. Understanding Authentication Methods
Before implementing authentication, it’s essential to understand the available methods. Ionic supports various authentication options, including email and password, social media authentication (e.g., Facebook, Google), and single sign-on (SSO) solutions like OAuth 2.0.
3.2. Implementing Email and Password Authentication
Email and password authentication is a common method for user registration and login. Ionic provides a user-friendly way to implement this. Below is a basic example of how to set up email and password authentication in your Ionic app:
typescript import { Component } from '@angular/core'; import { AngularFireAuth } from '@angular/fire/auth'; @Component({ selector: 'app-auth', templateUrl: 'auth.page.html', styleUrls: ['auth.page.scss'], }) export class AuthPage { constructor(public auth: AngularFireAuth) {} async login(email: string, password: string) { try { await this.auth.signInWithEmailAndPassword(email, password); // Redirect to the authenticated page } catch (error) { console.error('Authentication failed', error); } } async register(email: string, password: string) { try { await this.auth.createUserWithEmailAndPassword(email, password); // Redirect to the authenticated page } catch (error) { console.error('Registration failed', error); } } }
The above code uses Firebase Authentication, a popular choice for user authentication in Ionic apps.
3.3. Social Media Authentication
Social media authentication allows users to log in using their existing social media accounts, reducing friction during registration. Ionic offers plugins like @ionic-native/facebook and @ionic-native/google-plus to simplify the implementation of social media login.
4. Best Practices for User Authentication
4.1. Secure Password Storage
Storing passwords securely is crucial. Never store plain text passwords in your database. Instead, use strong encryption techniques like bcrypt to hash passwords before saving them.
javascript const bcrypt = require('bcrypt'); const plaintextPassword = 'mySecurePassword'; const saltRounds = 10; bcrypt.hash(plaintextPassword, saltRounds, (err, hash) => { if (err) { throw err; } // Store the 'hash' in your database });
4.2. Session Management
Maintaining user sessions securely is essential for keeping users logged in. Use JSON Web Tokens (JWTs) to create and verify user sessions. Ionic and various backend frameworks offer JWT libraries for easy integration.
javascript const jwt = require('jsonwebtoken'); const user = { id: '123', username: 'exampleUser', }; const secretKey = 'your-secret-key'; const token = jwt.sign(user, secretKey, { expiresIn: '1h' });
4.3. Two-Factor Authentication (2FA)
Enhance security further by implementing two-factor authentication. This adds an extra layer of protection by requiring users to provide two forms of identification, typically something they know (password) and something they have (e.g., a one-time code sent to their email or phone).
5. Securing Your API
User authentication is not limited to the client-side of your app. You must also secure your app’s backend. Here are some essential considerations:
5.1. OAuth 2.0 and JWT
When building an API, consider using OAuth 2.0 for securing endpoints. OAuth allows you to grant limited access to your API to third-party applications, and JWTs can be used to verify the identity of users making requests to your server.
5.2. CORS and Security Headers
Implement Cross-Origin Resource Sharing (CORS) to control which domains can access your API. Additionally, set security headers like Content Security Policy (CSP) and HTTP Strict Transport Security (HSTS) to further enhance your API’s security.
6. Testing Your Authentication System
To ensure your authentication system is robust and secure, you should conduct thorough testing. Here are two types of testing you should consider:
6.1. Unit Testing
Write unit tests for individual components of your authentication system, such as registration, login, and session management. Tools like Jasmine and Karma can help you perform unit tests.
6.2. Integration Testing
Integration testing involves testing the interactions between various components of your app, including the frontend, backend, and database. Tools like Postman and Jest are useful for integration testing.
Conclusion
Securing user access to your Ionic app through robust authentication mechanisms is a fundamental step in ensuring the safety of user data and building trust with your audience. In this guide, we’ve covered the importance of user authentication, the various methods available in Ionic, best practices, and tips for securing your app’s API. By following these guidelines, you can create a safer and more reliable app while instilling confidence in your users.
Remember, user authentication is an ongoing process. Stay informed about the latest security trends and continuously update and improve your authentication system to protect your users and your app’s reputation.
Table of Contents
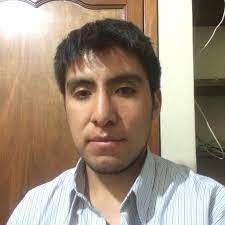
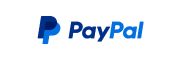