Ionic and User Feedback: Gathering Insights for App Improvement
In today’s fast-paced world of mobile app development, user feedback is the key to creating successful and user-friendly applications. Gathering insights from your users allows you to understand their needs, pain points, and preferences, which in turn enables you to make informed decisions for app improvement. In this blog, we will explore how to leverage Ionic, a popular framework for building cross-platform mobile apps, to gather user feedback effectively. We will delve into strategies, tools, and provide code samples to help you implement user feedback mechanisms seamlessly into your Ionic app.
Table of Contents
1. Why User Feedback Matters
Before we dive into the technical aspects of collecting user feedback with Ionic, let’s first understand why user feedback is crucial for app development.
1.1. Enhancing User Experience
User feedback provides valuable insights into how users interact with your app. It allows you to identify pain points, bugs, and areas where improvements are needed. By addressing these issues, you can enhance the overall user experience and increase user satisfaction.
1.2. Prioritizing Features and Improvements
Not all app features or improvements are created equal. User feedback helps you prioritize what to work on next. You can focus on the most critical issues or features that matter the most to your users, ensuring that your development efforts align with user expectations.
1.3. Increasing User Engagement
When users feel heard and see their suggestions being implemented, they are more likely to engage with your app regularly. This engagement can lead to increased user retention and word-of-mouth recommendations, helping your app grow organically.
Now that we understand the importance of user feedback, let’s explore how to gather it effectively using Ionic.
2. Gathering User Feedback with Ionic
Ionic is a powerful framework for building cross-platform mobile apps using web technologies like HTML, CSS, and JavaScript. It offers various tools and plugins that make it easier to collect user feedback seamlessly.
2.1. In-App Surveys
One effective way to gather user feedback is by integrating in-app surveys into your Ionic app. Surveys allow you to ask specific questions to users about their experience, preferences, and suggestions. To implement in-app surveys in Ionic, you can use plugins like Ionic-Survey-Prompt. Here’s a code sample to get you started:
javascript import { SurveyPrompt } from 'ionic-survey-prompt'; // Create a survey const survey = new SurveyPrompt({ title: 'We value your feedback!', questions: [ { type: 'text', name: 'feedback', title: 'What do you like most about the app?', }, { type: 'text', name: 'improvements', title: 'How can we improve the app?', }, ], }); // Display the survey when needed survey.present();
With this code, you can easily create and display a survey within your Ionic app, allowing users to provide feedback directly.
2.2. In-App Feedback Forms
In addition to surveys, you can implement in-app feedback forms that users can access at any time. These forms should include fields for users to enter their comments, suggestions, and bug reports. Here’s an example of how to create a simple feedback form in Ionic:
html <ion-header> <ion-toolbar> <ion-title>Feedback</ion-title> </ion-toolbar> </ion-header> <ion-content> <ion-item> <ion-label position="stacked">Your Feedback</ion-label> <ion-textarea [(ngModel)]="userFeedback"></ion-textarea> </ion-item> <ion-button expand="full" (click)="submitFeedback()">Submit</ion-button> </ion-content> javascript import { Component } from '@angular/core'; @Component({ selector: 'app-feedback', templateUrl: 'feedback.page.html', styleUrls: ['feedback.page.scss'], }) export class FeedbackPage { userFeedback: string; constructor() {} submitFeedback() { // Send the user feedback to your server or perform any desired action console.log('User feedback:', this.userFeedback); // You can also display a confirmation message to the user } }
This code creates a simple feedback form where users can enter their feedback, and the data can be sent to your server for further analysis.
2.3. Integration with Analytics Tools
Another valuable approach to gather user feedback is by integrating your Ionic app with analytics tools. Services like Google Analytics and Mixpanel can provide insights into user behavior, allowing you to identify areas of improvement based on data. For example, you can track user flows, screen views, and user engagement metrics to pinpoint issues and trends.
To integrate Google Analytics with your Ionic app, follow these steps:
Install the cordova-plugin-google-analytics plugin:
bash ionic cordova plugin add cordova-plugin-google-analytics
Implement Google Analytics in your app by adding the following code to your Ionic project:
javascript import { Injectable } from '@angular/core'; import { Platform } from 'ionic-angular'; import { GoogleAnalytics } from '@ionic-native/google-analytics'; @Injectable() export class AnalyticsProvider { constructor(public platform: Platform, public ga: GoogleAnalytics) { this.platform.ready().then(() => { this.ga.startTrackerWithId('YOUR-GA-TRACKING-ID') .then(() => { console.log('Google Analytics is ready now'); }) .catch(e => console.log('Error starting Google Analytics', e)); }); } trackEvent(category: string, action: string, label?: string, value?: number) { this.ga.trackEvent(category, action, label, value); } }
Don’t forget to replace ‘YOUR-GA-TRACKING-ID’ with your actual Google Analytics tracking ID.
With this integration, you can start tracking user interactions and behavior within your Ionic app. Analyzing this data will help you identify areas where user feedback and improvements are needed.
2.4. Feedback Widgets
Consider adding feedback widgets or buttons within your Ionic app to make it easy for users to provide feedback at any time. These widgets can be placed strategically, such as on settings screens or within the app’s navigation menu. Here’s an example of how to create a simple feedback widget:
html <ion-fab vertical="bottom" horizontal="end" slot="fixed"> <ion-fab-button (click)="openFeedbackModal()"> <ion-icon name="chatbubble-ellipses"></ion-icon> </ion-fab-button> </ion-fab> javascript import { Component } from '@angular/core'; import { ModalController } from '@ionic/angular'; @Component({ selector: 'app-home', templateUrl: 'home.page.html', styleUrls: ['home.page.scss'], }) export class HomePage { constructor(private modalController: ModalController) {} async openFeedbackModal() { const modal = await this.modalController.create({ component: FeedbackModalComponent, cssClass: 'feedback-modal', }); return await modal.present(); } } @Component({ selector: 'app-feedback-modal', templateUrl: 'feedback-modal.page.html', styleUrls: ['feedback-modal.page.scss'], }) export class FeedbackModalComponent { constructor(private modalController: ModalController) {} close() { this.modalController.dismiss(); } }
In this code, a floating action button (FAB) with a chat bubble icon serves as a feedback widget. When users tap the button, a feedback modal is displayed, allowing them to provide comments and suggestions.
3. Analyzing and Acting on User Feedback
Collecting user feedback is just the beginning. To derive meaningful insights and drive app improvement, you need to analyze the feedback systematically and take appropriate actions.
3.1. Feedback Categorization
Start by categorizing the feedback you receive. Group similar feedback together to identify recurring issues or feature requests. Common categories include usability, performance, bugs, and feature requests.
3.2. Prioritization
Prioritize the feedback based on factors such as the frequency of occurrence, impact on user experience, and alignment with your app’s goals. Use a system like the MoSCoW method (Must-haves, Should-haves, Could-haves, Won’t-haves) to prioritize features and improvements.
3.3. Continuous Iteration
Implement a regular feedback loop where you gather feedback, make improvements, and then gather more feedback. This iterative process ensures that your app evolves to meet user expectations continuously.
3.4. Communication
Communicate with your users transparently. Let them know that their feedback is valued and that you are working to address their concerns. Providing updates on implemented changes fosters trust and encourages further feedback.
Conclusion
User feedback is an invaluable resource for app improvement, and Ionic provides various tools and strategies to help you gather feedback effectively. Whether it’s through in-app surveys, feedback forms, analytics integration, or feedback widgets, Ionic empowers you to listen to your users and make data-driven decisions.
Remember that collecting feedback is not a one-time task; it’s an ongoing process. Regularly analyzing and acting on user feedback will lead to a better user experience, increased user engagement, and a more successful app overall. So, start harnessing the power of Ionic to gather insights for app improvement and watch your app thrive in the competitive mobile landscape.
Table of Contents
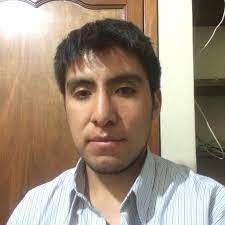
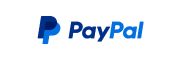