Navigating the Quest for Hiring Java Developers: A Comprehensive Java Developer Interview Questions Guide
Java, the versatile and powerful programming language, has been a cornerstone of software development for decades. To create robust applications and systems, hiring skilled Java developers is paramount. This guide serves as your compass through the hiring expedition, empowering you to evaluate candidates’ technical prowess, problem-solving abilities, and Java expertise. Let’s embark on this exploration, uncovering essential interview questions and strategies to identify the perfect Java developer for your team.
Table of Contents
1. How to Hire Java Developers
Embarking on the mission to hire Java developers? Follow these steps for a successful journey:
- Job Requirements: Define specific job requirements, outlining the skills and experience you’re seeking.
- Search Channels: Utilize job postings, online platforms, and tech communities to discover potential candidates.
- Screening: Scrutinize candidates’ Java proficiency, relevant experience, and additional skills.
- Technical Assessment: Develop a comprehensive technical assessment to evaluate coding abilities and problem-solving aptitude.
2. Core Skills of Java Developers to Look For
When evaluating Java developers, be on the lookout for these core skills:
- Java Proficiency: A strong grasp of Java syntax, object-oriented programming (OOP), and design patterns.
- Framework Knowledge: Familiarity with popular Java frameworks like Spring or Hibernate.
- Database Expertise: Knowledge of working with databases, SQL, and database management.
- API Integration: Experience integrating with external APIs and services to enhance application functionality.
- Multithreading Skills: Expertise in working with multithreading and concurrent programming concepts.
- Unit Testing: Proficiency in writing unit tests using frameworks like JUnit.
- Problem-Solving Abilities: Ability to analyze complex problems and devise elegant solutions.
3. Overview of the Java Developer Hiring Process
Here’s an overview of the Java developer hiring process:
3.1 Defining Job Requirements and Skillsets
Lay the foundation by outlining clear job prerequisites, specifying the skills and knowledge you’re seeking.
3.2 Crafting Compelling Job Descriptions
Create captivating job descriptions that accurately convey the role, attracting the right candidates.
3.3 Crafting Java Developer Interview Questions
Develop a comprehensive set of interview questions covering Java intricacies, problem-solving aptitude, and relevant technologies.
4. Sample Java Developer Interview Questions and Answers
Explore these sample questions with detailed answers to assess candidates’ Java skills:
Q1. Explain the concept of object-oriented programming (OOP) in Java and provide an example of an OOP principle.
A: Object-oriented programming is a programming paradigm that uses objects and classes to organize and structure code. An example of an OOP principle is encapsulation, which restricts direct access to an object’s internal state.
Q2. Write a Java method that sorts an array of integers using the bubble sort algorithm.
public static void bubbleSort(int[] arr) { int n = arr.length; for (int i = 0; i < n - 1; i++) { for (int j = 0; j < n - i - 1; j++) { if (arr[j] > arr[j + 1]) { int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } }
Q3. Explain the difference between the ==
operator and the equals()
method in Java.
A: The ==
operator compares the memory references of objects, while the equals()
method compares the content or values of objects. The equals()
method can be overridden to provide custom comparison logic.
Q4. What is the purpose of the static
keyword in Java, and how is it used?
A: The static
keyword in Java is used to declare a class-level member that belongs to the class itself, rather than an instance of the class. It can be used with variables, methods, and nested classes.
Q5. Write a Java class representing a Person
with properties for name
and age
. Include a constructor and getter methods.
public class Person { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public int getAge() { return age; } }
Q6. Explain the concept of Java Generics and how they enhance type safety in collections.
A: Java Generics allow you to write reusable and type-safe code by parameterizing types. They enable you to define classes, interfaces, and methods that work with various types while ensuring type safety at compile time.
Q7. Write a Java program that reads a file using the FileReader
class and counts the number of words in it.
import java.io.*; public class WordCount { public static void main(String[] args) throws IOException { File file = new File("sample.txt"); FileReader reader = new FileReader(file); BufferedReader br = new BufferedReader(reader); String line; int wordCount = 0; while ((line = br.readLine()) != null) { String[] words = line.split(" "); wordCount += words.length; } br.close(); System.out.println("Total words: " + wordCount); } }
Q8. Explain the concept of polymorphism in Java and how it is achieved using method overriding.
A: Polymorphism in Java allows objects of different classes to be treated as objects of a common superclass. Method overriding enables a subclass to provide a specific implementation of a method defined in its superclass.
Q9. Write a Java program that demonstrates the usage of the try-catch-finally
block for exception handling.
public class ExceptionHandlingDemo { public static void main(String[] args) { try { int result = divide(10, 0); System.out.println("Result: " + result); } catch (ArithmeticException e) { System.out.println("Error: " + e.getMessage()); } finally { System.out.println("Execution completed."); } } public static int divide(int dividend, int divisor) { return dividend / divisor; } }
Q10. Explain the concept of Java Annotations and provide an example of a built-in annotation.
A: Java Annotations provide metadata to code and can be used for documentation, code generation, and runtime processing. An example of a built-in annotation is @Override
, which indicates that a method is intended to override a method in a superclass.
5. Hiring Java Developers through CloudDevs
Step 1: Connect with CloudDevs: Initiate a conversation with a CloudDevs consultant to discuss your project’s requirements, preferred skills, and expected experience.
Step 2: Find Your Ideal Match: Within 24 hours, CloudDevs presents you with carefully selected Java developers from their pool of pre-vetted professionals. Review their profiles and select the candidate who aligns with your project’s vision.
Step 3: Embark on a Risk-Free Trial: Engage in discussions with your chosen developer to ensure a smooth onboarding process. Once satisfied, formalize the collaboration and commence a week-long free trial.
By leveraging the expertise of CloudDevs, you can effortlessly identify and hire exceptional Java developers, ensuring your team possesses the skills required to build remarkable applications and systems.
6. Conclusion
With these additional technical questions and insights at your disposal, you’re now well-prepared to assess Java developers comprehensively. Whether you’re crafting intricate algorithms or architecting powerful applications, securing the right Java developers for your team is the foundation of your project’s success.
Table of Contents
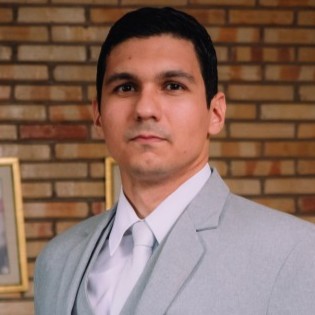
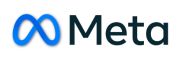