Java Servlets: Building Dynamic Web Applications
In the ever-evolving landscape of web development, building dynamic and interactive web applications is a must. Java Servlets provide a powerful solution for creating such applications, allowing developers to handle client requests, process data, and generate dynamic responses. In this guide, we’ll delve into the world of Java Servlets and learn how to leverage their capabilities to build robust and engaging web applications.
Table of Contents
1. Understanding Java Servlets
1.1. What are Servlets?
Java Servlets are Java classes that extend the capabilities of a server. They handle client requests and generate dynamic responses, making them a cornerstone of web application development. Servlets provide a platform-independent way of building web-based applications and interacting with web clients.
1.2. Servlet Lifecycle
Understanding the lifecycle of a servlet is essential for effective development. The key stages include:
- Initialization: When the servlet container initializes the servlet instance by calling the init() method.
- Request Handling: During this phase, the servlet handles incoming client requests by executing the service() method. This method processes the request and generates the appropriate response.
- Destruction: When the servlet container shuts down or decides to unload the servlet, it calls the destroy() method, allowing the servlet to clean up resources.
2. Setting Up Your Development Environment
2.1. Installing Java Development Kit (JDK)
To start with servlet development, ensure that you have the Java Development Kit (JDK) installed on your machine. You can download the latest version of JDK from the official Oracle website or adopt open-source alternatives like OpenJDK.
2.2. Configuring a Servlet Container (Apache Tomcat)
A servlet container is required to run servlets. Apache Tomcat is one of the most popular servlet containers. Download the appropriate version of Tomcat, extract the archive, and set up environment variables like CATALINA_HOME to point to the Tomcat installation directory.
3. Creating Your First Servlet
3.1. Creating a Maven Project
Maven simplifies project management and dependency resolution. Create a Maven project and add the necessary dependencies for servlet development. Your pom.xml should include the Servlet API dependency.
xml <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>5.0.0</version> <scope>provided</scope> </dependency>
3.2. Writing a Simple Servlet
Create a Java class that extends javax.servlet.http.HttpServlet. Override the doGet() method to handle GET requests.
java import javax.servlet.*; import javax.servlet.http.*; import java.io.IOException; public class MyServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); PrintWriter out = response.getWriter(); out.println("<html><body>"); out.println("<h1>Hello, Servlet!</h1>"); out.println("</body></html>"); } }
4. Handling Client Requests
4.1. HTTP GET and POST Requests
Servlets can handle different types of HTTP requests, primarily GET and POST. Use the doGet() method for GET requests and the doPost() method for POST requests.
java @Override protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // Handle POST request }
4.2. Retrieving Request Parameters
Servlets can extract parameters from the request URL or the request body for processing.
java String username = request.getParameter("username"); String password = request.getParameter("password");
5. Generating Dynamic Responses
5.1. Building HTML with Servlets
Servlets can dynamically generate HTML content and send it as a response.
java PrintWriter out = response.getWriter(); out.println("<html><body>"); out.println("<h1>Welcome to Dynamic Servlets!</h1>"); out.println("</body></html>");
5.2. Incorporating Dynamic Data
Servlets can retrieve data from databases, APIs, or other sources to dynamically populate web pages.
java List<Product> products = productService.getAllProducts(); for (Product product : products) { out.println("<p>" + product.getName() + ": $" + product.getPrice() + "</p>"); }
6. Managing Sessions and Cookies
6.1. Using HttpSession for State Management
Servlets can create and manage sessions to maintain user-specific data across requests.
java HttpSession session = request.getSession(); session.setAttribute("user", currentUser);
6.2. Implementing Cookies for Tracking
Cookies allow servlets to store small pieces of information on the client’s browser.
java Cookie languageCookie = new Cookie("language", "en_US"); languageCookie.setMaxAge(60 * 60 * 24 * 30); // 30 days response.addCookie(languageCookie);
7. Servlet Filters
7.1. Introduction to Filters
Servlet filters intercept requests and responses to perform tasks such as logging, authentication, and data transformation.
java public class LoggingFilter implements Filter { @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { // Log request details chain.doFilter(request, response); // Log response details } }
8. Best Practices for Servlet Development
8.1. Separation of Concerns
Follow the MVC (Model-View-Controller) pattern to separate application logic from presentation.
8.2. Exception Handling
Implement robust error handling to provide meaningful error messages to users and log errors for debugging.
9. Deploying Servlets
9.1. Packaging Your Application
Package your servlet and related files into a WAR (Web Application Archive) file.
9.2. Deploying on a Servlet Container
Copy the WAR file to the appropriate directory in the servlet container (e.g., Tomcat’s webapps directory) to deploy your application.
Conclusion
Java Servlets offer a powerful foundation for building dynamic web applications. From handling client requests to generating dynamic responses, managing sessions, and deploying your application, servlets provide the tools you need to create engaging and interactive web experiences. By mastering the concepts and best practices outlined in this guide, you’re well-equipped to embark on your journey of servlet-based web development.
In conclusion, Java Servlets remain a crucial technology for web application development, allowing developers to create feature-rich and interactive web applications that cater to modern user expectations. With their ability to handle client requests, process data, and generate dynamic responses, Java Servlets offer a versatile and powerful framework for building dynamic web applications. Whether you’re developing e-commerce platforms, social networking sites, or content management systems, understanding Java Servlets is a skill that can significantly enhance your capabilities as a web developer. So, dive into the world of Java Servlets, explore their intricacies, and unlock a realm of possibilities for building the next generation of web applications.
Table of Contents
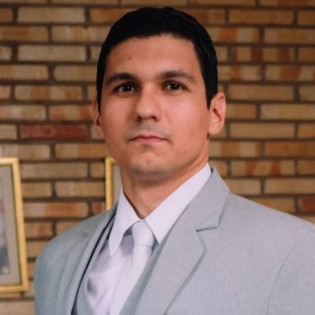
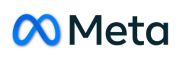