Java XML Processing: Parsing and Manipulating XML Data
In the realm of data exchange and storage, XML (eXtensible Markup Language) has stood the test of time as a versatile format. Java, a powerful and popular programming language, provides robust support for XML processing. Whether you’re working with configuration files, web services, or any other data interchange scenarios, Java XML processing can be your reliable companion. In this blog, we will dive into the world of XML processing using Java, focusing on parsing and manipulating XML data.
Table of Contents
1. Understanding XML and Its Importance:
1.1. What is XML?
XML, or eXtensible Markup Language, is a widely used markup language that defines rules for encoding documents in a human-readable and machine-readable format. Unlike HTML, which has predefined tags, XML allows users to define their tags, making it highly customizable for various data representation needs.
1.2. Why is XML Used?
XML finds applications in various domains, including web services, configuration files, data interchange between different platforms, and more. Its simplicity, readability, and self-descriptive nature have contributed to its longevity in an ever-evolving tech landscape.
1.3. Java’s XML Processing Capabilities:
Java provides comprehensive support for XML processing through a set of built-in APIs. These APIs allow developers to parse, manipulate, and generate XML documents seamlessly.
2. Parsing XML Data:
Parsing involves extracting meaningful information from an XML document. Java offers several APIs for XML parsing:
2.1. Using DOM (Document Object Model):
DOM parsing involves loading the entire XML document into memory as a tree structure. This enables easy navigation and manipulation of elements but can be memory-intensive for large documents.
java // DOM Parser Example DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document document = builder.parse(new File("data.xml")); // Accessing elements Element rootElement = document.getDocumentElement(); NodeList nodeList = rootElement.getElementsByTagName("book");
2.2. Using SAX (Simple API for XML):
SAX parsing is an event-driven approach where the parser reads the document sequentially and triggers events (e.g., start element, end element, text content) as it encounters different parts of the document. This approach is memory-efficient but less intuitive for complex manipulations.
java // SAX Parser Example SAXParserFactory factory = SAXParserFactory.newInstance(); SAXParser saxParser = factory.newSAXParser(); DefaultHandler handler = new DefaultHandler() { // Event handling methods }; saxParser.parse("data.xml", handler);
2.3. Using StAX (Streaming API for XML):
StAX provides a balance between DOM and SAX. It allows developers to iterate through the XML document sequentially like SAX, but it also enables bidirectional movement. This makes it suitable for both reading and writing XML.
java // StAX Parser Example XMLInputFactory factory = XMLInputFactory.newInstance(); XMLStreamReader reader = factory.createXMLStreamReader(new FileInputStream("data.xml")); while (reader.hasNext()) { int eventType = reader.next(); // Event handling based on the eventType }
3. Manipulating XML Data:
Java’s XML APIs also enable manipulation of XML documents:
3.1. Modifying Elements and Attributes:
Once an XML document is parsed, you can modify its elements and attributes using the appropriate APIs.
java // Modifying XML using DOM NodeList bookNodes = rootElement.getElementsByTagName("book"); Element firstBook = (Element) bookNodes.item(0); Element titleElement = (Element) firstBook.getElementsByTagName("title").item(0); titleElement.setTextContent("New Title");
3.2. Adding and Deleting Nodes:
You can add new elements, attributes, or entire nodes to an XML document.
java // Adding a new element using DOM Element newBook = document.createElement("book"); Element newTitle = document.createElement("title"); newTitle.setTextContent("Brand New Title"); newBook.appendChild(newTitle); rootElement.appendChild(newBook); // Deleting an element using DOM NodeList bookNodes = rootElement.getElementsByTagName("book"); Node bookToRemove = bookNodes.item(1); rootElement.removeChild(bookToRemove);
4. Working with Real-world Examples:
4.1. Reading and Updating Configuration Files:
XML is often used for configuration files. Java’s XML processing capabilities make it easy to read and modify these files.
java // Reading and updating configuration using DOM DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance(); DocumentBuilder builder = factory.newDocumentBuilder(); Document configDocument = builder.parse(new File("config.xml")); // Updating a configuration value Element timeoutElement = (Element) configDocument.getElementsByTagName("timeout").item(0); timeoutElement.setTextContent("5000");
4.2. Consuming XML-based Web Services:
Many web services use XML as the data exchange format. Java allows you to parse and process responses from these services effectively.
java // Consuming XML-based web service response using StAX XMLInputFactory factory = XMLInputFactory.newInstance(); XMLStreamReader reader = factory.createXMLStreamReader(webServiceResponse); while (reader.hasNext()) { int eventType = reader.next(); // Process events and extract data }
5. Best Practices for Efficient XML Processing:
- Choose the Right Parsing Approach: Consider the nature of your XML document and the intended manipulations. Choose between DOM, SAX, and StAX based on factors like memory usage, processing speed, and ease of use.
- Handling Large XML Files: For large XML documents, consider using SAX or StAX to avoid excessive memory consumption. Break down the processing into smaller chunks and handle events as they are encountered.
Conclusion
Java’s XML processing capabilities empower developers to work with XML data seamlessly. Whether you’re parsing complex documents, modifying configuration files, or consuming web services, Java’s built-in APIs offer a variety of approaches to cater to your specific needs. By mastering XML processing in Java, you unlock a powerful toolset for managing data interchange and manipulation across various domains.
Table of Contents
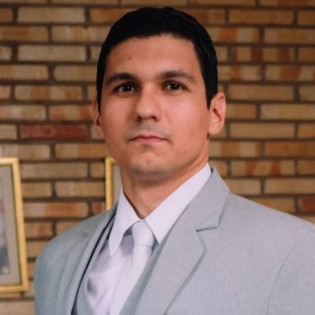
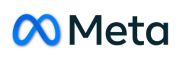