Simplifying JavaScript Animation with Tweening Functions
In the dynamic world of web development, animations play a pivotal role in creating engaging user experiences. From subtle fades to complex transitions, animations bring life to web interfaces and captivate users’ attention. While there are various libraries and frameworks available to handle animations, understanding the underlying concepts is crucial for creating smooth and efficient animations. One such concept is tweening functions.
Table of Contents
1. Understanding Tweening Functions
Tweening functions, also known as easing functions, are mathematical equations that determine how values change over time, typically in animations. They control the pace of change, allowing for smooth and natural transitions rather than linear and abrupt shifts. Think of tweening functions as the artists of animation, influencing the motion’s flow and character.
Consider a scenario where you want to animate an element from its initial position to a final position. Instead of a sudden jump, you can apply a tweening function to control the animation’s progression. This results in a more visually appealing and intuitive effect.
2. Benefits of Using Tweening Functions
- Smooth Transitions: Tweening functions provide gradual transitions between values, making animations more aesthetically pleasing.
- Enhanced User Experience: Smooth animations improve the overall user experience by reducing jarring effects and making interactions feel natural.
- Easy Implementation: Integrating tweening functions into your animations is relatively simple and requires only a few lines of code.
- Code Readability: Tweening functions enhance code readability by abstracting complex animation logic into concise and understandable snippets.
Now, let’s dive into some practical implementations of tweening functions using JavaScript.
3. Basic Linear Tweening
Linear tweening is the simplest form of easing. It creates a consistent rate of change throughout the animation. While it lacks the visual flair of more complex easing functions, it still provides a basic level of smoothness.
javascript function linearTween(t, b, c, d) { return c * t / d + b; } // Usage example const startValue = 0; const endValue = 100; const duration = 1000; // in milliseconds let currentTime = 0; function animate() { const currentValue = linearTween(currentTime, startValue, endValue - startValue, duration); // Update the element's position using currentValue // ... currentTime += 16; // Assuming 60 FPS if (currentTime <= duration) { requestAnimationFrame(animate); } } animate();
4. Ease-In and Ease-Out Functions
Ease-in and ease-out functions provide a more realistic animation experience by gradually accelerating at the beginning and decelerating toward the end. These functions are widely used in animations to simulate natural motion.
javascript // Ease-in function function easeInQuad(t, b, c, d) { t /= d; return c * t * t + b; } // Ease-out function function easeOutQuad(t, b, c, d) { t /= d; return -c * t * (t - 2) + b; }
5. Implementing Ease-In and Ease-Out
javascript const startValue = 0; const endValue = 100; const duration = 1000; let currentTime = 0; function animate() { const currentValue = easeInQuad(currentTime, startValue, endValue - startValue, duration); // Update the element's position using currentValue // ... currentTime += 16; if (currentTime <= duration) { requestAnimationFrame(animate); } } animate();
6. Customizing Easing Curves
If you’re looking to create unique animations, you can experiment with custom easing curves. These curves can produce various effects such as bounce, elastic, and back-and-forth animations.
javascript // Custom bounce easing function function customBounce(t, b, c, d) { if ((t /= d) < 1 / 2.75) { return c * (7.5625 * t * t) + b; } else if (t < 2 / 2.75) { return c * (7.5625 * (t -= 1.5 / 2.75) * t + 0.75) + b; } else if (t < 2.5 / 2.75) { return c * (7.5625 * (t -= 2.25 / 2.75) * t + 0.9375) + b; } else { return c * (7.5625 * (t -= 2.625 / 2.75) * t + 0.984375) + b; } }
7. Utilizing the Custom Easing Curve
javascript const startValue = 0; const endValue = 100; const duration = 1000; let currentTime = 0; function animate() { const currentValue = customBounce(currentTime, startValue, endValue - startValue, duration); // Update the element's position using currentValue // ... currentTime += 16; if (currentTime <= duration) { requestAnimationFrame(animate); } } animate();
8. Combining Multiple Animations
Tweening functions can also be combined to create more complex animations. For instance, you can use an ease-in function followed by an ease-out function to achieve a “pop” effect.
javascript const startValue = 0; const endValue = 100; const duration = 1000; let currentTime = 0; function animate() { let currentValue; if (currentTime < duration / 2) { currentValue = easeInQuad(currentTime, startValue, endValue - startValue, duration / 2); } else { currentValue = easeOutQuad(currentTime - duration / 2, startValue, endValue - startValue, duration / 2); } // Update the element's position using currentValue // ... currentTime += 16; if (currentTime <= duration) { requestAnimationFrame(animate); } } animate();
Conclusion
Tweening functions offer a powerful and accessible way to create captivating animations in JavaScript. By understanding the various easing functions and their applications, you can elevate your web projects with visually appealing and user-friendly animations. From linear motion to custom bounces, the flexibility and simplicity of tweening functions empower developers to bring life to their designs effortlessly. So, the next time you embark on an animation journey, remember that a well-chosen easing function can make all the difference in crafting a delightful user experience.
Table of Contents
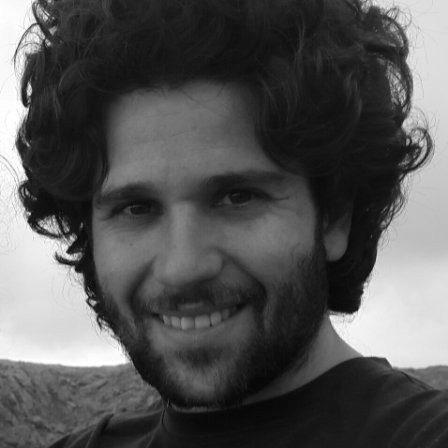
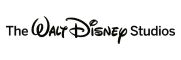