Exploring JavaScript Function Decorators for Code Enhancement
When it comes to writing clean, efficient, and maintainable code in JavaScript, developers often turn to various programming techniques and design patterns. One such technique that has gained significant popularity in recent years is function decorators. Function decorators allow developers to enhance the behavior of functions without modifying their original code, promoting code reusability, modularity, and separation of concerns. In this article, we’ll dive deep into the world of JavaScript function decorators, explore their benefits, and provide practical examples to help you grasp their potential.
Table of Contents
1. Understanding Function Decorators
A function decorator is essentially a higher-order function that wraps another function, adding extra functionality to it. It’s like adding a new layer of behavior to an existing function while keeping its core logic intact. Decorators provide a way to modify or extend the behavior of functions without directly altering their implementation. This makes your code more flexible and easier to maintain.
2. Benefits of Using Function Decorators
Function decorators offer several advantages that contribute to cleaner and more organized code:
- Code Reusability: By separating the concerns of different functionalities into decorators, you can reuse the same decorators across multiple functions. This eliminates code duplication and promotes a more modular codebase.
- Readability: Decorators make it easier to understand the purpose of a function by encapsulating specific behavior within separate decorators. This enhances code readability and makes it more self-explanatory.
- Maintainability: Instead of modifying a function directly to add new features, you can create decorators that encapsulate these features. This reduces the risk of introducing bugs or unintended side effects.
- Separation of Concerns: Decorators enable you to separate cross-cutting concerns, such as logging, authentication, or caching, from the core logic of your functions. This separation simplifies the main function and isolates the additional functionality.
3. Implementing Function Decorators
To implement a function decorator in JavaScript, you need to follow a few steps. Let’s break down the process with a simple example:
Suppose you have a basic function that calculates the sum of two numbers:
javascript function add(a, b) { return a + b; }
Now, let’s say you want to log every call to this function along with its arguments and result. You can achieve this using a decorator:
javascript function logDecorator(func) { return function(...args) { const result = func(...args); console.log(`Function ${func.name} called with args ${args.join(', ')} and returned ${result}`); return result; }; } const decoratedAdd = logDecorator(add); decoratedAdd(3, 5);
In this example, the logDecorator function takes another function as its parameter and returns a new function that wraps the original function. The new function logs the details and then calls the original function, forwarding its arguments. This way, you’ve added logging functionality to the add function without altering its code.
4. Chaining Decorators
One of the fascinating aspects of function decorators is that you can chain them together to enhance a function’s behavior in multiple ways. Let’s illustrate this concept with an example:
Suppose you have a function that calculates the total price of items in a shopping cart:
javascript function calculateTotalPrice(cart) { return cart.reduce((total, item) => total + item.price, 0); }
Now, you want to apply two decorators: one to apply a discount and another to add tax to the calculated total. Here’s how you can achieve this:
javascript function discountDecorator(func) { return function(cart) { const discountedCart = cart.map(item => ({ ...item, price: item.price * 0.9 })); return func(discountedCart); }; } function taxDecorator(func) { return function(cart) { const total = func(cart); const taxedTotal = total * 1.1; return taxedTotal; }; } const enhancedTotal = taxDecorator(discountDecorator(calculateTotalPrice)); const cartItems = [ { name: 'Item 1', price: 100 }, { name: 'Item 2', price: 75 }, { name: 'Item 3', price: 50 } ]; console.log(enhancedTotal(cartItems));
In this example, the discountDecorator modifies the prices of items in the cart before passing it to the next decorator or the original function. The taxDecorator calculates the taxed total and returns the final amount. By stacking decorators, you’ve effectively added discount and tax functionalities to the calculateTotalPrice function.
5. Common Use Cases for Function Decorators
Function decorators find applications in various scenarios, including:
- Logging and Monitoring: Decorators can log function calls, their arguments, execution times, and results. This is especially useful for debugging and performance optimization.
- Caching: You can implement caching decorators that store the results of expensive function calls and return cached results for subsequent calls with the same arguments.
- Authorization and Authentication: Decorators can enforce access control by checking user permissions or verifying authentication before allowing a function to execute.
- Validation: Decorators can validate function inputs, ensuring that arguments meet specific criteria before executing the core logic.
- Memoization: Similar to caching, memoization decorators store results of function calls to avoid redundant calculations, specifically useful for functions with heavy computations.
Conclusion
JavaScript function decorators provide an elegant and powerful way to enhance the behavior of functions without modifying their original code. They promote code reusability, modularity, and separation of concerns, leading to cleaner and more maintainable codebases. By understanding the concept of decorators and exploring practical examples, you can take your coding skills to the next level and write more efficient and flexible JavaScript applications. Whether it’s logging, caching, authentication, or any other cross-cutting concern, function decorators empower you to build more robust and feature-rich applications while keeping your codebase organized and readable. So, dive into the world of decorators and unlock the potential of your JavaScript code.
In conclusion, function decorators are a valuable tool in a JavaScript developer’s toolkit. They empower you to enhance your codebase with additional functionalities without sacrificing the original logic. With the benefits of reusability, readability, maintainability, and separation of concerns, decorators contribute to building scalable and efficient applications. So, the next time you find yourself wanting to augment the behavior of your functions, remember the power of JavaScript function decorators. Happy coding!
Table of Contents
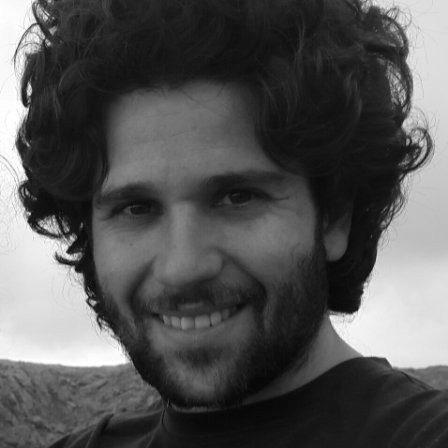
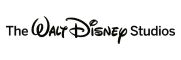